Working with Date and Time Pickers in Flutter
May 7, 2025



Summary
Summary
Summary
Summary
Flutter’s built-in showDatePicker and showTimePicker functions allow developers to capture user-selected date and time inputs easily. This tutorial walks through integrating these widgets, handling asynchronous selection, formatting output with intl, and customizing themes—all with minimal code.
Flutter’s built-in showDatePicker and showTimePicker functions allow developers to capture user-selected date and time inputs easily. This tutorial walks through integrating these widgets, handling asynchronous selection, formatting output with intl, and customizing themes—all with minimal code.
Flutter’s built-in showDatePicker and showTimePicker functions allow developers to capture user-selected date and time inputs easily. This tutorial walks through integrating these widgets, handling asynchronous selection, formatting output with intl, and customizing themes—all with minimal code.
Flutter’s built-in showDatePicker and showTimePicker functions allow developers to capture user-selected date and time inputs easily. This tutorial walks through integrating these widgets, handling asynchronous selection, formatting output with intl, and customizing themes—all with minimal code.
Key insights:
Key insights:
Key insights:
Key insights:
Async Picker Integration: Both pickers return
Future
values and can be chained for combined datetime selection.Reusable UI Components: Separating pickers into standalone methods keeps your form code modular.
Intl-Based Formatting: Use the
intl
package to format dates and times based on locale.Theming and Styling: Customize pickers’ appearance by wrapping them in themed
Builder
widgets.Localized UX: The
locale
parameter ensures pickers align with user language preferences.No External Dependencies Needed: Native Flutter pickers cover most common use cases with full customization.
Introduction
Working with date picker and time picker widgets is fundamental for many Flutter apps—whether scheduling events, logging timestamps, or simply letting users select a date range. In this tutorial, you’ll learn how to integrate Flutter’s built-in pickers, handle asynchronous results, format user selections, and apply basic customizations. By the end, you’ll have a clean, reusable picker component you can drop into any form.
Setting Up Your Flutter Project
Before writing picker code, ensure you have:
• Flutter SDK (v2.0+) installed
• A new or existing Flutter app scaffolded (flutter create my_app)
• The intl package added for date formatting
Add intl to your pubspec.yaml:
dependencies:
flutter:
sdk: flutter
intl
Run flutter pub get
then import it where needed.
Implementing a Date Picker
Use Flutter’s showDatePicker function inside a stateful widget to let users pick dates. Here’s a minimal example:
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
class DatePickerExample extends StatefulWidget {
@override
_DatePickerExampleState createState() => _DatePickerExampleState();
}
class _DatePickerExampleState extends State<DatePickerExample> {
DateTime? _selectedDate;
Future<void> _pickDate() async {
final now = DateTime.now();
final picked = await showDatePicker(
context: context,
initialDate: _selectedDate ?? now,
firstDate: DateTime(now.year - 5),
lastDate: DateTime(now.year + 5),
);
if (picked != null) {
setState(() => _selectedDate = picked);
}
}
@override
Widget build(BuildContext context) {
final text = _selectedDate == null
? 'No date chosen'
: DateFormat.yMMMMd().format(_selectedDate!);
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text('Selected date: $text'),
ElevatedButton(
onPressed: _pickDate,
child: Text('Open Date Picker'),
),
],
);
}
}
Key points:
initialDate
,firstDate
,lastDate
define the selectable range.The result is
Future<DateTime?>
, so you await it.Format with
DateFormat
fromintl
.
Adding a Time Picker
Similarly, use Flutter’s showTimePicker
to pick times:
Future<void> _pickTime() async {
final picked = await showTimePicker(
context: context,
initialTime: TimeOfDay.now(),
);
if (picked != null) {
setState(() => _selectedTime = picked);
}
}
Integrate it in your widget:
• Declare TimeOfDay? _selectedTime;
• Display the value via _selectedTime?.format(context) ?? 'No time chosen'
• Trigger with a button labeled “Open Time Picker”
By separating date picker and time picker logic, you maintain modular code and can reuse each component independently.
Building a Combined DateTime Picker
To let users pick both date and time in sequence, chain the two pickers:
• Call _pickDate(), then if a date is chosen, call _pickTime().
• Combine into a DateTime object.
Example snippet inside your state class:
Future<void> _pickDateTime() async {
final date = await showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2020),
lastDate: DateTime(2030),
);
if (date == null) return;
final time = await showTimePicker(
context: context,
initialTime: TimeOfDay(hour: 9, minute: 0),
);
if (time == null) return;
setState(() {
_selectedDateTime = DateTime(
date.year, date.month, date.day, time.hour, time.minute);
});
}
This approach delivers a simple datetime picker without external packages.
Customizing the Pickers
Out of the box, Flutter’s pickers inherit your app’s theme. You can override colors, shapes, and locale:
• Wrap showDatePicker in a Theme widget to supply a custom ThemeData for dialog.
• Pass locale parameter to localize month and weekday names.
• Use builder to wrap the dialog in additional styling:
await showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2000),
lastDate: DateTime(2100),
builder: (ctx, child) {
return Theme(
data: Theme.of(ctx).copyWith(
colorScheme: ColorScheme.light(
primary: Colors.deepPurple,
onSurface: Colors.black,
),
),
child: child!,
);
},
);
This snippet changes the primary color of the calendar header and selected date.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
You now know how to integrate date picker, time picker, and combined datetime picker widgets in Flutter. With this foundation, you can extend pickers further or integrate third-party datetime packages for advanced use cases. Happy coding!
Introduction
Working with date picker and time picker widgets is fundamental for many Flutter apps—whether scheduling events, logging timestamps, or simply letting users select a date range. In this tutorial, you’ll learn how to integrate Flutter’s built-in pickers, handle asynchronous results, format user selections, and apply basic customizations. By the end, you’ll have a clean, reusable picker component you can drop into any form.
Setting Up Your Flutter Project
Before writing picker code, ensure you have:
• Flutter SDK (v2.0+) installed
• A new or existing Flutter app scaffolded (flutter create my_app)
• The intl package added for date formatting
Add intl to your pubspec.yaml:
dependencies:
flutter:
sdk: flutter
intl
Run flutter pub get
then import it where needed.
Implementing a Date Picker
Use Flutter’s showDatePicker function inside a stateful widget to let users pick dates. Here’s a minimal example:
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
class DatePickerExample extends StatefulWidget {
@override
_DatePickerExampleState createState() => _DatePickerExampleState();
}
class _DatePickerExampleState extends State<DatePickerExample> {
DateTime? _selectedDate;
Future<void> _pickDate() async {
final now = DateTime.now();
final picked = await showDatePicker(
context: context,
initialDate: _selectedDate ?? now,
firstDate: DateTime(now.year - 5),
lastDate: DateTime(now.year + 5),
);
if (picked != null) {
setState(() => _selectedDate = picked);
}
}
@override
Widget build(BuildContext context) {
final text = _selectedDate == null
? 'No date chosen'
: DateFormat.yMMMMd().format(_selectedDate!);
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text('Selected date: $text'),
ElevatedButton(
onPressed: _pickDate,
child: Text('Open Date Picker'),
),
],
);
}
}
Key points:
initialDate
,firstDate
,lastDate
define the selectable range.The result is
Future<DateTime?>
, so you await it.Format with
DateFormat
fromintl
.
Adding a Time Picker
Similarly, use Flutter’s showTimePicker
to pick times:
Future<void> _pickTime() async {
final picked = await showTimePicker(
context: context,
initialTime: TimeOfDay.now(),
);
if (picked != null) {
setState(() => _selectedTime = picked);
}
}
Integrate it in your widget:
• Declare TimeOfDay? _selectedTime;
• Display the value via _selectedTime?.format(context) ?? 'No time chosen'
• Trigger with a button labeled “Open Time Picker”
By separating date picker and time picker logic, you maintain modular code and can reuse each component independently.
Building a Combined DateTime Picker
To let users pick both date and time in sequence, chain the two pickers:
• Call _pickDate(), then if a date is chosen, call _pickTime().
• Combine into a DateTime object.
Example snippet inside your state class:
Future<void> _pickDateTime() async {
final date = await showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2020),
lastDate: DateTime(2030),
);
if (date == null) return;
final time = await showTimePicker(
context: context,
initialTime: TimeOfDay(hour: 9, minute: 0),
);
if (time == null) return;
setState(() {
_selectedDateTime = DateTime(
date.year, date.month, date.day, time.hour, time.minute);
});
}
This approach delivers a simple datetime picker without external packages.
Customizing the Pickers
Out of the box, Flutter’s pickers inherit your app’s theme. You can override colors, shapes, and locale:
• Wrap showDatePicker in a Theme widget to supply a custom ThemeData for dialog.
• Pass locale parameter to localize month and weekday names.
• Use builder to wrap the dialog in additional styling:
await showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2000),
lastDate: DateTime(2100),
builder: (ctx, child) {
return Theme(
data: Theme.of(ctx).copyWith(
colorScheme: ColorScheme.light(
primary: Colors.deepPurple,
onSurface: Colors.black,
),
),
child: child!,
);
},
);
This snippet changes the primary color of the calendar header and selected date.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
You now know how to integrate date picker, time picker, and combined datetime picker widgets in Flutter. With this foundation, you can extend pickers further or integrate third-party datetime packages for advanced use cases. Happy coding!
Add pickers effortlessly with Vibe Studio
Add pickers effortlessly with Vibe Studio
Add pickers effortlessly with Vibe Studio
Add pickers effortlessly with Vibe Studio
Vibe Studio’s visual builder and AI assistant help you integrate localized, themed pickers into your app—no boilerplate required.
Vibe Studio’s visual builder and AI assistant help you integrate localized, themed pickers into your app—no boilerplate required.
Vibe Studio’s visual builder and AI assistant help you integrate localized, themed pickers into your app—no boilerplate required.
Vibe Studio’s visual builder and AI assistant help you integrate localized, themed pickers into your app—no boilerplate required.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






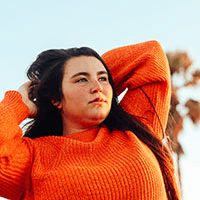



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025