Server‑Driven UI in Flutter Using JSON Configuration
May 7, 2025



Summary
Summary
Summary
Summary
Server-driven UI in Flutter lets apps fetch and render JSON-defined layouts at runtime, enabling fast iterations and personalization. This tutorial outlines schema design, dynamic widget creation, and action handling. With Vibe Studio, developers can rapidly build and iterate Flutter apps powered by flexible, server-controlled interfaces.
Server-driven UI in Flutter lets apps fetch and render JSON-defined layouts at runtime, enabling fast iterations and personalization. This tutorial outlines schema design, dynamic widget creation, and action handling. With Vibe Studio, developers can rapidly build and iterate Flutter apps powered by flexible, server-controlled interfaces.
Server-driven UI in Flutter lets apps fetch and render JSON-defined layouts at runtime, enabling fast iterations and personalization. This tutorial outlines schema design, dynamic widget creation, and action handling. With Vibe Studio, developers can rapidly build and iterate Flutter apps powered by flexible, server-controlled interfaces.
Server-driven UI in Flutter lets apps fetch and render JSON-defined layouts at runtime, enabling fast iterations and personalization. This tutorial outlines schema design, dynamic widget creation, and action handling. With Vibe Studio, developers can rapidly build and iterate Flutter apps powered by flexible, server-controlled interfaces.
Key insights:
Key insights:
Key insights:
Key insights:
JSON-Defined Layouts: Use widget
type
,properties
,children
, andaction
fields in a clear schema.Dynamic Widget Builder: A factory maps JSON nodes to Flutter widgets for runtime rendering.
Action Handling: Actions are string-mapped callbacks, enabling interactive UIs.
Theming Support: Extend JSON to include color, font, and spacing keys merged into
ThemeData
.Offline & Secure: Cache JSON locally and use encryption for sensitive payloads.
Production Ready: Validate schemas and monitor deep UI trees for performance.
Introduction
In a traditional Flutter app, UI definitions live in code. With server-driven UI, the app fetches JSON configurations at runtime and renders widgets dynamically. This approach enables on-the-fly UI modifications, A/B testing, and faster iteration without deploying a new binary. In this tutorial, you’ll learn how to implement a server driven UI in Flutter using JSON configuration. We’ll cover schema design, dynamic widget building, and handling user interactions.
Designing Your JSON Schema
A clear JSON schema is the foundation of any server-driven UI solution. Define a minimal set of widget types, properties, and event hooks. For example:
{
"type": "column",
"children": [
{
"type": "text",
"data": "Welcome to dynamic UI!",
"style": {"fontSize": 20, "color": "#333333"}
},
{
"type": "button",
"label": "Click Me",
"action": "incrementCounter"
}
]
}
Key concepts:
• type: Widget identifier (e.g., “text”, “button”, “container”).
• properties: Styling and content parameters.
• children: Nested elements for multi-child widgets.
• action: Named callback to wire events back into your logic.
Fetching Configuration from Server
Leverage Flutter’s http package to fetch your JSON at startup or upon a pull-to-refresh.
import 'dart:convert';
import 'package:http/http.dart' as http;
Future<Map<String, dynamic>> fetchConfig() async {
final res = await http.get(Uri.parse('https://api.example.com/ui-config'));
if (res.statusCode == 200) return json.decode(res.body);
throw Exception('Failed to load UI config');
}
Invoke fetchConfig() in your main widget’s initState(), then pass the parsed map into your renderer.
Building a Dynamic UI Builder
Create a factory function that maps JSON nodes to Flutter widgets. This core engine enables your server-driven UI. Keep it extensible—each time you support a new widget or style property, add a new case.
Widget buildFromJson(Map<String, dynamic> node, Function actionHandler) {
switch (node['type']) {
case 'text':
return Text(
node['data'] ?? '',
style: TextStyle(
fontSize: (node['style']?['fontSize'] ?? 14).toDouble(),
color: Color(int.parse(node['style']?['color']?.substring(1) ?? 'FF000000', radix: 16)),
),
);
case 'button':
return ElevatedButton(
onPressed: () => actionHandler(node['action']),
child: Text(node['label'] ?? ''),
);
case 'column':
return Column(
children: (node['children'] as List)
.map((child) => buildFromJson(child, actionHandler))
.toList(),
);
default:
return SizedBox.shrink();
}
}
Key points:
• actionHandler: A callback registry that connects JSON-defined actions to Dart functions.
• Default cases: Gracefully handle unsupported types.
Managing State and Actions
Server driven UI shines when your actions update the app state or trigger navigation. Define an actionHandler that maps string keys to methods. For example:
void handleAction(String action) {
switch (action) {
case 'incrementCounter':
setState(() => counter++);
break;
case 'navigateHome':
Navigator.pushNamed(context, '/home');
break;
// add more handlers as needed
}
}
Integrate this into your StatefulWidget:
class DynamicScreen extends StatefulWidget {
@override
_DynamicScreenState createState() => _DynamicScreenState();
}
class _DynamicScreenState extends State<DynamicScreen> {
Map<String, dynamic>? config;
int counter = 0;
@override
void initState() {
super.initState();
fetchConfig().then((cfg) => setState(() => config = cfg));
}
@override
Widget build(BuildContext context) {
if (config == null) return Center(child: CircularProgressIndicator());
return Scaffold(
appBar: AppBar(title: Text('Server-Driven UI')),
body: Padding(
padding: EdgeInsets.all(16),
child: buildFromJson(config!, handleAction),
),
);
}
}
Styling and Theming
Extend your JSON schema to include theme keys—colors, fonts, spacing—and merge them with Flutter’s ThemeData. This enables global style overrides from the server, but always validate incoming values to prevent runtime errors.
Tips for Production
• Validate JSON schemas server-side.
• Cache configurations locally for offline support.
• Encrypt or sign payloads if your UI includes sensitive logic.
• Monitor performance: deep widget trees may need optimization (e.g., ListView.builder).
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
By offloading UI definitions to a backend, your team can deploy visual updates without waiting for app store approvals—a true server driven ui approach. You now have the building blocks: a clear JSON schema, a dynamic widget builder, and a robust action handler.
Embrace server-driven UI to iterate faster, personalize experiences, and decouple UI changes from app releases—your next update can be as simple as tweaking a JSON file on your server.
Introduction
In a traditional Flutter app, UI definitions live in code. With server-driven UI, the app fetches JSON configurations at runtime and renders widgets dynamically. This approach enables on-the-fly UI modifications, A/B testing, and faster iteration without deploying a new binary. In this tutorial, you’ll learn how to implement a server driven UI in Flutter using JSON configuration. We’ll cover schema design, dynamic widget building, and handling user interactions.
Designing Your JSON Schema
A clear JSON schema is the foundation of any server-driven UI solution. Define a minimal set of widget types, properties, and event hooks. For example:
{
"type": "column",
"children": [
{
"type": "text",
"data": "Welcome to dynamic UI!",
"style": {"fontSize": 20, "color": "#333333"}
},
{
"type": "button",
"label": "Click Me",
"action": "incrementCounter"
}
]
}
Key concepts:
• type: Widget identifier (e.g., “text”, “button”, “container”).
• properties: Styling and content parameters.
• children: Nested elements for multi-child widgets.
• action: Named callback to wire events back into your logic.
Fetching Configuration from Server
Leverage Flutter’s http package to fetch your JSON at startup or upon a pull-to-refresh.
import 'dart:convert';
import 'package:http/http.dart' as http;
Future<Map<String, dynamic>> fetchConfig() async {
final res = await http.get(Uri.parse('https://api.example.com/ui-config'));
if (res.statusCode == 200) return json.decode(res.body);
throw Exception('Failed to load UI config');
}
Invoke fetchConfig() in your main widget’s initState(), then pass the parsed map into your renderer.
Building a Dynamic UI Builder
Create a factory function that maps JSON nodes to Flutter widgets. This core engine enables your server-driven UI. Keep it extensible—each time you support a new widget or style property, add a new case.
Widget buildFromJson(Map<String, dynamic> node, Function actionHandler) {
switch (node['type']) {
case 'text':
return Text(
node['data'] ?? '',
style: TextStyle(
fontSize: (node['style']?['fontSize'] ?? 14).toDouble(),
color: Color(int.parse(node['style']?['color']?.substring(1) ?? 'FF000000', radix: 16)),
),
);
case 'button':
return ElevatedButton(
onPressed: () => actionHandler(node['action']),
child: Text(node['label'] ?? ''),
);
case 'column':
return Column(
children: (node['children'] as List)
.map((child) => buildFromJson(child, actionHandler))
.toList(),
);
default:
return SizedBox.shrink();
}
}
Key points:
• actionHandler: A callback registry that connects JSON-defined actions to Dart functions.
• Default cases: Gracefully handle unsupported types.
Managing State and Actions
Server driven UI shines when your actions update the app state or trigger navigation. Define an actionHandler that maps string keys to methods. For example:
void handleAction(String action) {
switch (action) {
case 'incrementCounter':
setState(() => counter++);
break;
case 'navigateHome':
Navigator.pushNamed(context, '/home');
break;
// add more handlers as needed
}
}
Integrate this into your StatefulWidget:
class DynamicScreen extends StatefulWidget {
@override
_DynamicScreenState createState() => _DynamicScreenState();
}
class _DynamicScreenState extends State<DynamicScreen> {
Map<String, dynamic>? config;
int counter = 0;
@override
void initState() {
super.initState();
fetchConfig().then((cfg) => setState(() => config = cfg));
}
@override
Widget build(BuildContext context) {
if (config == null) return Center(child: CircularProgressIndicator());
return Scaffold(
appBar: AppBar(title: Text('Server-Driven UI')),
body: Padding(
padding: EdgeInsets.all(16),
child: buildFromJson(config!, handleAction),
),
);
}
}
Styling and Theming
Extend your JSON schema to include theme keys—colors, fonts, spacing—and merge them with Flutter’s ThemeData. This enables global style overrides from the server, but always validate incoming values to prevent runtime errors.
Tips for Production
• Validate JSON schemas server-side.
• Cache configurations locally for offline support.
• Encrypt or sign payloads if your UI includes sensitive logic.
• Monitor performance: deep widget trees may need optimization (e.g., ListView.builder).
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
By offloading UI definitions to a backend, your team can deploy visual updates without waiting for app store approvals—a true server driven ui approach. You now have the building blocks: a clear JSON schema, a dynamic widget builder, and a robust action handler.
Embrace server-driven UI to iterate faster, personalize experiences, and decouple UI changes from app releases—your next update can be as simple as tweaking a JSON file on your server.
Iterate UI Without Releasing
Iterate UI Without Releasing
Iterate UI Without Releasing
Iterate UI Without Releasing
Vibe Studio empowers you to create and update server-driven UIs for Flutter—fast, safe, and visually.
Vibe Studio empowers you to create and update server-driven UIs for Flutter—fast, safe, and visually.
Vibe Studio empowers you to create and update server-driven UIs for Flutter—fast, safe, and visually.
Vibe Studio empowers you to create and update server-driven UIs for Flutter—fast, safe, and visually.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






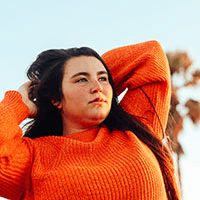



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025