Securing Your Flutter App with Firebase Security Rules
May 6, 2025



Summary
Summary
Summary
Summary
The article explains best practices for using Firebase Security Rules in Flutter apps, covering syntax, fine-grained access control, testing strategies, and production deployment. It also introduces Vibe Studio, a no-code platform powered by Steve, to streamline app development with secure Firebase integration.
The article explains best practices for using Firebase Security Rules in Flutter apps, covering syntax, fine-grained access control, testing strategies, and production deployment. It also introduces Vibe Studio, a no-code platform powered by Steve, to streamline app development with secure Firebase integration.
The article explains best practices for using Firebase Security Rules in Flutter apps, covering syntax, fine-grained access control, testing strategies, and production deployment. It also introduces Vibe Studio, a no-code platform powered by Steve, to streamline app development with secure Firebase integration.
The article explains best practices for using Firebase Security Rules in Flutter apps, covering syntax, fine-grained access control, testing strategies, and production deployment. It also introduces Vibe Studio, a no-code platform powered by Steve, to streamline app development with secure Firebase integration.
Key insights:
Key insights:
Key insights:
Key insights:
Declarative Rule Syntax: Firebase Security Rules use a structured, JSON-like language to control database access.
Fine-Grained Permissions: Implement row- and role-based access using request.auth and custom claims.
Deny-by-Default Approach: Only explicitly allow necessary operations to reduce security risks.
Local Testing Tools: Use the Firebase emulator and testing libraries to validate rule behavior pre-deployment.
Seamless Flutter Integration: Rules automatically apply to calls from initialized Firebase packages in Flutter.
Production Best Practices: Use rules_version = '2', enforce data validation, and monitor usage via Firebase Console.
Introduction
Securing your Flutter app backend is critical to protect user data and maintain trust. Firebase Security Rules offer a declarative way to enforce authentication, authorization, and data validation directly at the database layer. In this tutorial, you’ll learn best practices for writing and deploying firebase security rules, how to implement fine-grained access control, and how to integrate these rules seamlessly into your Flutter project.
Understanding Firebase Security Rules Syntax and Structure
Firebase security rules are written in a JSON-like language that executes on the server before any read or write operation. Key elements include:
• match/path: Specifies which document or collection the rule applies to
• allow read, write: Defines permitted operations
• request.auth: Contains authentication information
• resource.data: The existing data for reads; request.resource.data is new data for writes
Example of basic Firestore rules:
rules_version = '2';
service cloud.firestore {
match /databases/{database}/documents {
match /posts/{postId} {
allow read: if true;
allow write: if
In the snippet above, anyone can read posts, but only the original author can update them. This enforces row-level security via Firebase rules.
Implementing Granular Read/Write Permissions
To secure your collections, define both collection- and document-level rules. For example, an “orders” collection needs different constraints for creation, updates, and deletions:
service cloud.firestore {
match /databases/{database}/documents {
match /orders/{orderId} {
allow create: if request.auth.uid != null
&& request.resource.data.userId == request.auth.uid;
allow update: if request.auth.uid == resource.data.userId
&& request.resource.data.status in ['shipped','delivered'];
allow delete: if false; // Prevent deletions
allow read: if request.auth.uid == resource.data.userId;
}
}
}
Best practices:
• Deny by default. Only open up the minimal read/write paths your app requires.
• Leverage request.auth.token claims to implement role-based access by adding custom claims on the server.
• Validate data shape and types using conditional checks (is string, size(), matches()).
Testing and Debugging Your Rules
Before going live, simulate read/write operations in the Firebase Console Rules Playground or use the Firebase emulator suite:
• emulator: Run firebase emulators:start to test security rules locally against your Flutter app.
• firestore.rules.testing: Write unit tests in Node.js or use the Flutter integration test package to trigger operations and assert their outcomes.
Emulator example (Node.js snippet):
const firebase = require("@firebase/testing");
const projectId = "demo-app";
const auth = { uid: "alice" };
const db = firebase
.initializeTestApp({ projectId, auth })
.firestore();
await firebase.assertSucceeds(
db.collection("orders").add({ userId: "alice", item: "widget" })
);
Robust rule testing ensures you don’t expose or block data incorrectly once deployed.
Integrating Security Rules in Flutter
Your Flutter app interacts with Firebase through the official packages. Ensure you initialize Firebase Auth and Firestore before any operation:
import 'package:firebase_core/firebase_core.dart';
import 'package:cloud_firestore/cloud_firestore.dart';
import 'package:firebase_auth/firebase_auth.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
Future<void> createOrder(String item) async {
final uid = FirebaseAuth.instance.currentUser?.uid;
if (uid == null) throw Exception('Not authenticated');
await FirebaseFirestore.instance.collection('orders').add({
'userId': uid,
'item': item,
'status': 'pending'
});
}
The security rules you authored will automatically enforce on each call. No extra client-side code is required to “turn on” authorization.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing robust security rules for Firebase is indispensable for any production-grade Flutter application. By following a deny-by-default approach, validating inputs at the rules layer, and thoroughly testing via the emulator, you’ll lock down your Firestore or Realtime Database against unauthorized access.
Introduction
Securing your Flutter app backend is critical to protect user data and maintain trust. Firebase Security Rules offer a declarative way to enforce authentication, authorization, and data validation directly at the database layer. In this tutorial, you’ll learn best practices for writing and deploying firebase security rules, how to implement fine-grained access control, and how to integrate these rules seamlessly into your Flutter project.
Understanding Firebase Security Rules Syntax and Structure
Firebase security rules are written in a JSON-like language that executes on the server before any read or write operation. Key elements include:
• match/path: Specifies which document or collection the rule applies to
• allow read, write: Defines permitted operations
• request.auth: Contains authentication information
• resource.data: The existing data for reads; request.resource.data is new data for writes
Example of basic Firestore rules:
rules_version = '2';
service cloud.firestore {
match /databases/{database}/documents {
match /posts/{postId} {
allow read: if true;
allow write: if
In the snippet above, anyone can read posts, but only the original author can update them. This enforces row-level security via Firebase rules.
Implementing Granular Read/Write Permissions
To secure your collections, define both collection- and document-level rules. For example, an “orders” collection needs different constraints for creation, updates, and deletions:
service cloud.firestore {
match /databases/{database}/documents {
match /orders/{orderId} {
allow create: if request.auth.uid != null
&& request.resource.data.userId == request.auth.uid;
allow update: if request.auth.uid == resource.data.userId
&& request.resource.data.status in ['shipped','delivered'];
allow delete: if false; // Prevent deletions
allow read: if request.auth.uid == resource.data.userId;
}
}
}
Best practices:
• Deny by default. Only open up the minimal read/write paths your app requires.
• Leverage request.auth.token claims to implement role-based access by adding custom claims on the server.
• Validate data shape and types using conditional checks (is string, size(), matches()).
Testing and Debugging Your Rules
Before going live, simulate read/write operations in the Firebase Console Rules Playground or use the Firebase emulator suite:
• emulator: Run firebase emulators:start to test security rules locally against your Flutter app.
• firestore.rules.testing: Write unit tests in Node.js or use the Flutter integration test package to trigger operations and assert their outcomes.
Emulator example (Node.js snippet):
const firebase = require("@firebase/testing");
const projectId = "demo-app";
const auth = { uid: "alice" };
const db = firebase
.initializeTestApp({ projectId, auth })
.firestore();
await firebase.assertSucceeds(
db.collection("orders").add({ userId: "alice", item: "widget" })
);
Robust rule testing ensures you don’t expose or block data incorrectly once deployed.
Integrating Security Rules in Flutter
Your Flutter app interacts with Firebase through the official packages. Ensure you initialize Firebase Auth and Firestore before any operation:
import 'package:firebase_core/firebase_core.dart';
import 'package:cloud_firestore/cloud_firestore.dart';
import 'package:firebase_auth/firebase_auth.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
Future<void> createOrder(String item) async {
final uid = FirebaseAuth.instance.currentUser?.uid;
if (uid == null) throw Exception('Not authenticated');
await FirebaseFirestore.instance.collection('orders').add({
'userId': uid,
'item': item,
'status': 'pending'
});
}
The security rules you authored will automatically enforce on each call. No extra client-side code is required to “turn on” authorization.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing robust security rules for Firebase is indispensable for any production-grade Flutter application. By following a deny-by-default approach, validating inputs at the rules layer, and thoroughly testing via the emulator, you’ll lock down your Firestore or Realtime Database against unauthorized access.
Secure Flutter development—fast.
Secure Flutter development—fast.
Secure Flutter development—fast.
Secure Flutter development—fast.
Build production-ready Flutter apps with secure Firebase integration using Vibe Studio's conversational, no-code tools.
Build production-ready Flutter apps with secure Firebase integration using Vibe Studio's conversational, no-code tools.
Build production-ready Flutter apps with secure Firebase integration using Vibe Studio's conversational, no-code tools.
Build production-ready Flutter apps with secure Firebase integration using Vibe Studio's conversational, no-code tools.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






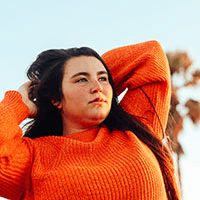



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025