Secure Storage with flutter secure storage
May 7, 2025



Summary
Summary
Summary
Summary
flutter_secure_storage provides encrypted, platform-backed key-value storage using Keychain and Keystore. This tutorial outlines its installation, usage, and best practices for safely handling sensitive data in Flutter apps. Integrated with Vibe Studio, developers can create secure, production-ready applications quickly and easily.
flutter_secure_storage provides encrypted, platform-backed key-value storage using Keychain and Keystore. This tutorial outlines its installation, usage, and best practices for safely handling sensitive data in Flutter apps. Integrated with Vibe Studio, developers can create secure, production-ready applications quickly and easily.
flutter_secure_storage provides encrypted, platform-backed key-value storage using Keychain and Keystore. This tutorial outlines its installation, usage, and best practices for safely handling sensitive data in Flutter apps. Integrated with Vibe Studio, developers can create secure, production-ready applications quickly and easily.
flutter_secure_storage provides encrypted, platform-backed key-value storage using Keychain and Keystore. This tutorial outlines its installation, usage, and best practices for safely handling sensitive data in Flutter apps. Integrated with Vibe Studio, developers can create secure, production-ready applications quickly and easily.
Key insights:
Key insights:
Key insights:
Key insights:
Cross-Platform Security: Uses iOS Keychain and Android Keystore for encrypted storage.
Simple API: Offers easy-to-use read, write, and delete methods for secure data handling.
Advanced Controls: Supports platform-specific options like Keychain accessibility and AES encryption.
Key Management: Namespacing and environment separation help manage and isolate stored data.
Exception Handling: Robust against edge cases like emulator limitations and device-specific quirks.
Biometric Integration: Can be paired with local_auth for added security during data access.
Introduction
In mobile development, sensitive data—API tokens, user credentials, encryption keys—must be safeguarded. Flutter provides several options, but for an intermediate-level, cross-platform, secure storage solution, flutter_secure_storage stands out. It leverages Keychain on iOS and Keystore on Android, offering a simple API to encrypt and securely store key-value pairs. In this tutorial, you’ll learn how to integrate and use flutter_secure_storage to securely store data in your Flutter apps.
Installation and Setup
Begin by adding the package to your pubspec.yaml:
dependencies:
flutter_secure_storage
Then fetch packages:
flutter pub get
On Android, ensure the minimum SDK version is at least 23 (Android 6.0) in android/app/build.gradle:
android {
defaultConfig {
minSdkVersion 23
}
}
No extra setup is needed for iOS, as Keychain access is enabled by default.
Initializing the Storage Client
Import and instantiate the client in your Dart code. This instance is the gateway to your secure storage operations.
import 'package:flutter_secure_storage/flutter_secure_storage.dart';
// Create a single instance for the entire app
final FlutterSecureStorage secureStorage = FlutterSecureStorage();
By default, data is encrypted and sandboxed per application. The secureStorage instance supports read/write/delete operations on key-value pairs.
Basic Usage: Read, Write, Delete
Use the following primary methods to interact with your secure storage.
Write a value:
await secureStorage.write(
key: 'accessToken',
value: 'your_jwt_token_here',
);
Read a value:
String? token = await secureStorage.read(key: 'accessToken');
Delete a single entry:
await secureStorage.delete(key: 'accessToken');
Delete all entries (clear storage):
await secureStorage.deleteAll();
These operations provide a robust foundation for securely storing small pieces of sensitive data.
Advanced Options and Best Practices
Secure storage isn’t just about storing and retrieving. Consider the following advanced techniques to maximize security:
• iOS: accessibility controls when Keychain items are accessible (e.g., AccessibleWhenUnlocked).
await secureStorage.write(
key: 'pinCode',
value: '1234',
iOptions: IOSOptions(
accessibility: IOSAccessibility.first_unlock,
),
);
• Android: encryptedSharedPreferences uses AES encryption under the hood for a fallback.
await secureStorage.write(
key: 'refreshToken',
value: 'abcd',
aOptions: AndroidOptions(
encryptedSharedPreferences: true,
),
);
• Namespacing Keys: Prefix your keys by feature or module to avoid collisions and ease cleanup:
const String authPrefix = 'auth_';
await secureStorage.write(
key: '${authPrefix}token',
value: token,
);
• Environment Separation: During development, use a different storage instance or key namespace to prevent accidental overwrites in production.
Handling Exceptions and Edge Cases
Even though flutter_secure_storage simplifies encryption, you should handle potential exceptions:
try {
await secureStorage.write(key: 'credential', value: data);
} on Exception catch (e) {
// Log or handle errors: Device might not support secure storage
debugPrint('Error writing to secure storage: $e');
}
• Device compatibility: Not all devices’ Keystore implementations are bulletproof. • Biometric authentication: Combine this package with local_auth for user verification before reading sensitive values.
Platform-Specific Considerations
Android emulator vs. physical device: Emulators may not fully implement hardware-backed Keystore. Always test on real devices. iOS simulator vs. device: Keychain behaves differently; simulators may lose stored values after app restart.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing a secure storage solution with flutter_secure_storage is straightforward yet powerful. You’ve covered installation, basic CRUD operations, platform-specific options, namespacing, and best practices for securely storing data. By integrating this package, you ensure that critical user information remains encrypted and protected at rest.
With a robust secure storage layer in place, your Flutter apps will stand resilient against data exposure—an essential step for any production-grade mobile application.
Introduction
In mobile development, sensitive data—API tokens, user credentials, encryption keys—must be safeguarded. Flutter provides several options, but for an intermediate-level, cross-platform, secure storage solution, flutter_secure_storage stands out. It leverages Keychain on iOS and Keystore on Android, offering a simple API to encrypt and securely store key-value pairs. In this tutorial, you’ll learn how to integrate and use flutter_secure_storage to securely store data in your Flutter apps.
Installation and Setup
Begin by adding the package to your pubspec.yaml:
dependencies:
flutter_secure_storage
Then fetch packages:
flutter pub get
On Android, ensure the minimum SDK version is at least 23 (Android 6.0) in android/app/build.gradle:
android {
defaultConfig {
minSdkVersion 23
}
}
No extra setup is needed for iOS, as Keychain access is enabled by default.
Initializing the Storage Client
Import and instantiate the client in your Dart code. This instance is the gateway to your secure storage operations.
import 'package:flutter_secure_storage/flutter_secure_storage.dart';
// Create a single instance for the entire app
final FlutterSecureStorage secureStorage = FlutterSecureStorage();
By default, data is encrypted and sandboxed per application. The secureStorage instance supports read/write/delete operations on key-value pairs.
Basic Usage: Read, Write, Delete
Use the following primary methods to interact with your secure storage.
Write a value:
await secureStorage.write(
key: 'accessToken',
value: 'your_jwt_token_here',
);
Read a value:
String? token = await secureStorage.read(key: 'accessToken');
Delete a single entry:
await secureStorage.delete(key: 'accessToken');
Delete all entries (clear storage):
await secureStorage.deleteAll();
These operations provide a robust foundation for securely storing small pieces of sensitive data.
Advanced Options and Best Practices
Secure storage isn’t just about storing and retrieving. Consider the following advanced techniques to maximize security:
• iOS: accessibility controls when Keychain items are accessible (e.g., AccessibleWhenUnlocked).
await secureStorage.write(
key: 'pinCode',
value: '1234',
iOptions: IOSOptions(
accessibility: IOSAccessibility.first_unlock,
),
);
• Android: encryptedSharedPreferences uses AES encryption under the hood for a fallback.
await secureStorage.write(
key: 'refreshToken',
value: 'abcd',
aOptions: AndroidOptions(
encryptedSharedPreferences: true,
),
);
• Namespacing Keys: Prefix your keys by feature or module to avoid collisions and ease cleanup:
const String authPrefix = 'auth_';
await secureStorage.write(
key: '${authPrefix}token',
value: token,
);
• Environment Separation: During development, use a different storage instance or key namespace to prevent accidental overwrites in production.
Handling Exceptions and Edge Cases
Even though flutter_secure_storage simplifies encryption, you should handle potential exceptions:
try {
await secureStorage.write(key: 'credential', value: data);
} on Exception catch (e) {
// Log or handle errors: Device might not support secure storage
debugPrint('Error writing to secure storage: $e');
}
• Device compatibility: Not all devices’ Keystore implementations are bulletproof. • Biometric authentication: Combine this package with local_auth for user verification before reading sensitive values.
Platform-Specific Considerations
Android emulator vs. physical device: Emulators may not fully implement hardware-backed Keystore. Always test on real devices. iOS simulator vs. device: Keychain behaves differently; simulators may lose stored values after app restart.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing a secure storage solution with flutter_secure_storage is straightforward yet powerful. You’ve covered installation, basic CRUD operations, platform-specific options, namespacing, and best practices for securely storing data. By integrating this package, you ensure that critical user information remains encrypted and protected at rest.
With a robust secure storage layer in place, your Flutter apps will stand resilient against data exposure—an essential step for any production-grade mobile application.
Secure Your Flutter Apps with Ease
Secure Your Flutter Apps with Ease
Secure Your Flutter Apps with Ease
Secure Your Flutter Apps with Ease
With Vibe Studio, you can integrate secure storage effortlessly and build production-ready apps faster.
With Vibe Studio, you can integrate secure storage effortlessly and build production-ready apps faster.
With Vibe Studio, you can integrate secure storage effortlessly and build production-ready apps faster.
With Vibe Studio, you can integrate secure storage effortlessly and build production-ready apps faster.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






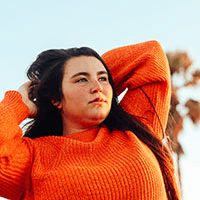



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025