Real-Time Data Syncing in Flutter with Firebase Realtime Database
May 7, 2025



Summary
Summary
Summary
Summary
Firebase Realtime Database enables real-time syncing in Flutter apps through live data streams, offline support, and structured reads/writes. This tutorial shows how to set up Firebase RTDB, listen to data changes using StreamBuilder, write data with set() and push(), and enable seamless offline handling for robust user experiences.
Firebase Realtime Database enables real-time syncing in Flutter apps through live data streams, offline support, and structured reads/writes. This tutorial shows how to set up Firebase RTDB, listen to data changes using StreamBuilder, write data with set() and push(), and enable seamless offline handling for robust user experiences.
Firebase Realtime Database enables real-time syncing in Flutter apps through live data streams, offline support, and structured reads/writes. This tutorial shows how to set up Firebase RTDB, listen to data changes using StreamBuilder, write data with set() and push(), and enable seamless offline handling for robust user experiences.
Firebase Realtime Database enables real-time syncing in Flutter apps through live data streams, offline support, and structured reads/writes. This tutorial shows how to set up Firebase RTDB, listen to data changes using StreamBuilder, write data with set() and push(), and enable seamless offline handling for robust user experiences.
Key insights:
Key insights:
Key insights:
Key insights:
Real-Time UI Updates:
onValue
streams ensure your widgets reflect the latest backend changes instantly.Simple Data Writes: Methods like
push()
andupdate()
make adding and modifying data intuitive.Offline First Support: Local caching ensures smooth user interactions without active connectivity.
Typed Data Access: Snapshots can be parsed into Dart maps for strong-typed UI rendering.
Performance Optimization: Flat data structures and
.indexOn
rules improve load times and queries.Safe Sync Practices: Clean up listeners with
cancel()
to prevent memory leaks in dynamic UIs.
Introduction
Real-time synchronization is at the heart of many modern mobile apps—chat, collaboration, live dashboards and more. In Flutter, pairing the firebase realtime database with its robust SDK delivers immediately updated data streams to your UI with minimal boilerplate. This tutorial dives into intermediate patterns for reading, writing, and syncing data using Firebase RTDB (Realtime Database) in Flutter.
Setting Up Flutter and Firebase Realtime Database Integration
Before diving into code, ensure you’ve:
• Created a Firebase project and enabled Realtime Database in the console.
• Added your Android and/or iOS app under Project Settings → Your Apps.
• Included the google-services.json (Android) or GoogleService-Info.plist (iOS) files in your project.
• Added dependencies to pubspec.yaml:
dependencies:
flutter:
sdk: flutter
firebase_core: ^2.0.0
firebase_database
Initialize Firebase early in your app—typically in main.dart:
import 'package:firebase_core/firebase_core.dart';
import 'package:flutter/material.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
This setup readies the firebase realtime database client.
Listening to Real-Time Data Changes
Flutter’s firebase_database package exposes a DatabaseReference that you can listen to as a stream. Wrap your UI in a StreamBuilder to rebuild on every change.
import 'package:firebase_database/firebase_database.dart';
import 'package:flutter/material.dart';
class ChatMessages extends StatelessWidget {
final DatabaseReference _messagesRef =
FirebaseDatabase.instance.ref('chat/messages');
@override
Widget build(BuildContext context) {
return StreamBuilder<DatabaseEvent>(
stream: _messagesRef.onValue,
builder: (context, snapshot) {
if (!snapshot.hasData || snapshot.data!.snapshot.value == null) {
return Center(child: Text('No messages yet.'));
}
final data = Map<String, dynamic>.from(
snapshot.data!.snapshot.value as Map);
final messages = data.values.toList();
return ListView.builder(
itemCount: messages.length,
itemBuilder: (c, i) => ListTile(
title: Text(messages[i]['author']),
subtitle: Text(messages[i]['text']),
),
);
},
);
}
}
Key points:
• ref('path') targets a node in your Firebase Realtime DB.
• onValue yields a continuous stream of DatabaseEvent.
• Converting raw snapshot data to Dart maps allows typed access.
Writing and Updating Data
To push new entries or update existing nodes, use push(), set(), and update() on a DatabaseReference.
final messagesRef = FirebaseDatabase.instance.ref('chat/messages');
void sendMessage(String author, String text) {
final newMsgRef = messagesRef.push();
newMsgRef.set({
'author': author,
'text': text,
'timestamp': ServerValue.timestamp,
});
}
void editMessage(String key, String newText) {
messagesRef.child(key).update({'text': newText});
}
• push() generates a unique child key—ideal for list-like data.
• set() writes or overwrites data at that location.
• update() modifies specific fields without clobbering siblings.
Handling Offline Scenarios
One of the firebase realtime database’s strengths is built-in offline support. To enable local persistence:
void enableOffline() {
FirebaseDatabase.instance.setPersistenceEnabled(true);
FirebaseDatabase.instance.setPersistenceCacheSizeBytes(10000000);
}
With persistence enabled, read/write operations are queued locally when offline and synchronized when connectivity returns. Your UI stream from onValue will reflect local cache updates instantly—giving users a snappy experience.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
By integrating firebase realtime database in Flutter, you gain a reactive, low-latency data layer that’s remarkably easy to wire into your widgets. Listening via StreamBuilder, writing with push(), and enabling offline persistence unlock powerful UX capabilities for shared and collaborative experiences.
Introduction
Real-time synchronization is at the heart of many modern mobile apps—chat, collaboration, live dashboards and more. In Flutter, pairing the firebase realtime database with its robust SDK delivers immediately updated data streams to your UI with minimal boilerplate. This tutorial dives into intermediate patterns for reading, writing, and syncing data using Firebase RTDB (Realtime Database) in Flutter.
Setting Up Flutter and Firebase Realtime Database Integration
Before diving into code, ensure you’ve:
• Created a Firebase project and enabled Realtime Database in the console.
• Added your Android and/or iOS app under Project Settings → Your Apps.
• Included the google-services.json (Android) or GoogleService-Info.plist (iOS) files in your project.
• Added dependencies to pubspec.yaml:
dependencies:
flutter:
sdk: flutter
firebase_core: ^2.0.0
firebase_database
Initialize Firebase early in your app—typically in main.dart:
import 'package:firebase_core/firebase_core.dart';
import 'package:flutter/material.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
This setup readies the firebase realtime database client.
Listening to Real-Time Data Changes
Flutter’s firebase_database package exposes a DatabaseReference that you can listen to as a stream. Wrap your UI in a StreamBuilder to rebuild on every change.
import 'package:firebase_database/firebase_database.dart';
import 'package:flutter/material.dart';
class ChatMessages extends StatelessWidget {
final DatabaseReference _messagesRef =
FirebaseDatabase.instance.ref('chat/messages');
@override
Widget build(BuildContext context) {
return StreamBuilder<DatabaseEvent>(
stream: _messagesRef.onValue,
builder: (context, snapshot) {
if (!snapshot.hasData || snapshot.data!.snapshot.value == null) {
return Center(child: Text('No messages yet.'));
}
final data = Map<String, dynamic>.from(
snapshot.data!.snapshot.value as Map);
final messages = data.values.toList();
return ListView.builder(
itemCount: messages.length,
itemBuilder: (c, i) => ListTile(
title: Text(messages[i]['author']),
subtitle: Text(messages[i]['text']),
),
);
},
);
}
}
Key points:
• ref('path') targets a node in your Firebase Realtime DB.
• onValue yields a continuous stream of DatabaseEvent.
• Converting raw snapshot data to Dart maps allows typed access.
Writing and Updating Data
To push new entries or update existing nodes, use push(), set(), and update() on a DatabaseReference.
final messagesRef = FirebaseDatabase.instance.ref('chat/messages');
void sendMessage(String author, String text) {
final newMsgRef = messagesRef.push();
newMsgRef.set({
'author': author,
'text': text,
'timestamp': ServerValue.timestamp,
});
}
void editMessage(String key, String newText) {
messagesRef.child(key).update({'text': newText});
}
• push() generates a unique child key—ideal for list-like data.
• set() writes or overwrites data at that location.
• update() modifies specific fields without clobbering siblings.
Handling Offline Scenarios
One of the firebase realtime database’s strengths is built-in offline support. To enable local persistence:
void enableOffline() {
FirebaseDatabase.instance.setPersistenceEnabled(true);
FirebaseDatabase.instance.setPersistenceCacheSizeBytes(10000000);
}
With persistence enabled, read/write operations are queued locally when offline and synchronized when connectivity returns. Your UI stream from onValue will reflect local cache updates instantly—giving users a snappy experience.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
By integrating firebase realtime database in Flutter, you gain a reactive, low-latency data layer that’s remarkably easy to wire into your widgets. Listening via StreamBuilder, writing with push(), and enabling offline persistence unlock powerful UX capabilities for shared and collaborative experiences.
Build real-time apps visually with Vibe Studio
Build real-time apps visually with Vibe Studio
Build real-time apps visually with Vibe Studio
Build real-time apps visually with Vibe Studio
Vibe Studio makes it effortless to integrate Firebase Realtime Database into your Flutter app—unlocking live syncing without code.
Vibe Studio makes it effortless to integrate Firebase Realtime Database into your Flutter app—unlocking live syncing without code.
Vibe Studio makes it effortless to integrate Firebase Realtime Database into your Flutter app—unlocking live syncing without code.
Vibe Studio makes it effortless to integrate Firebase Realtime Database into your Flutter app—unlocking live syncing without code.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






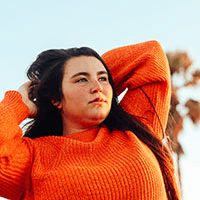



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025