Reactive Programming in Flutter: Streamlining State Management
May 6, 2025



Summary
Summary
Summary
Summary
The tutorial introduces reactive programming with Dart streams and Flutter’s StreamBuilder, showing how to emit and consume events for real-time UI updates using BLoC patterns—made even easier with no-code support from Vibe Studio.
The tutorial introduces reactive programming with Dart streams and Flutter’s StreamBuilder, showing how to emit and consume events for real-time UI updates using BLoC patterns—made even easier with no-code support from Vibe Studio.
The tutorial introduces reactive programming with Dart streams and Flutter’s StreamBuilder, showing how to emit and consume events for real-time UI updates using BLoC patterns—made even easier with no-code support from Vibe Studio.
The tutorial introduces reactive programming with Dart streams and Flutter’s StreamBuilder, showing how to emit and consume events for real-time UI updates using BLoC patterns—made even easier with no-code support from Vibe Studio.
Key insights:
Key insights:
Key insights:
Key insights:
Stream Fundamentals: Use
StreamController
to emit asynchronous events via.add()
and close them with.close()
.StreamBuilder UI:
StreamBuilder
rebuilds widgets as new stream data arrives, keeping UIs reactive.Resource Management: Always dispose controllers to prevent memory leaks and manage app lifecycle properly.
Bloc Architecture: Encapsulate state logic with input sinks and output streams for testable, modular code.
Advanced Operators: Use RxDart for operators like
debounce
,map
, andmerge
for richer stream control.Firebase Syncing: Combine Firestore’s
.snapshots()
stream withStreamBuilder
for real-time data binding.
Introduction
Reactive programming in Flutter leverages asynchronous data flows—known as streams—to update UI in response to state changes. Instead of manually calling setState, you subscribe widgets to streams that emit events. This approach decouples business logic from presentation, making apps more maintainable. In this tutorial, you’ll learn how to create, consume, and dispose streams for straightforward state management, all with concise Dart code.
Understanding Streams in Dart
A Dart Stream is a sequence of asynchronous data events. You can think of it like a conveyor belt that delivers values over time. There are two main types:
• Single-subscription streams—ideal for one listener.
• Broadcast streams—allow multiple listeners simultaneously.
To produce events, you typically use a StreamController. The controller provides a sink for input and exposes a stream for output.
Key methods:
• controller.add(value) pushes new data.
• controller.close() signals completion.
• Always close your controller to prevent memory leaks.
Building a Reactive Counter with StreamBuilder
Let’s build a simple counter that emits an integer each time a button is pressed.
import 'dart:async';
class CounterBloc {
final _controller = StreamController<int>();
int _count = 0;
Stream<int> get countStream => _controller.stream;
void increment() {
_controller.add(++_count);
}
void dispose() {
_controller.close();
}
}
Here, CounterBloc holds a private counter and exposes a Stream. Calling increment() emits the next value. Always call dispose() when the widget is removed to close the stream.
Displaying Stream Data with StreamBuilder
Flutter’s StreamBuilder widget listens to a stream and rebuilds when new data arrives. Embed it in your UI as follows:
import 'package:flutter/material.dart';
class CounterPage extends StatefulWidget {
@override
_CounterPageState createState() => _CounterPageState();
}
class _CounterPageState extends State<CounterPage> {
final bloc = CounterBloc();
@override
void dispose() {
bloc.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: StreamBuilder<int>(
stream: bloc.countStream,
initialData: 0,
builder: (_, snapshot) => Text(
'Count: ${snapshot.data}',
style: TextStyle(fontSize: 32),
),
),
),
floatingActionButton: FloatingActionButton(
onPressed: bloc.increment,
child: Icon(Icons.add),
),
);
}
}
In this snippet:
• stream binds your stream of events.
• initialData sets the starting UI state.
• The builder callback rebuilds the Text widget whenever the stream emits a new value.
Best Practices for Stream Management
• Always dispose controllers in dispose().
• Choose single-subscription streams for unique flows; use broadcast when multiple widgets need the same data.
• Consider packages like rxdart for advanced operators (map, debounce, merge).
• Structure your code with the BLoC (Business Logic Component) pattern: separate UI from logic, group sinks and streams in one class.
This results in testable, modular components. If you’re streaming data from Firebase, use FirebaseFirestore.instance.collection('items').snapshots() directly in a StreamBuilder for real-time syncing.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Reactive programming with streams in Flutter allows you to build responsive, maintainable applications by treating state changes as events. By encapsulating logic in classes like BLoCs, exposing streams, and consuming them via StreamBuilder, you achieve a clean separation of concerns and seamless UI updates. As you grow more comfortable, explore RxDart operators and higher-level state management libraries that build on streams.
Introduction
Reactive programming in Flutter leverages asynchronous data flows—known as streams—to update UI in response to state changes. Instead of manually calling setState, you subscribe widgets to streams that emit events. This approach decouples business logic from presentation, making apps more maintainable. In this tutorial, you’ll learn how to create, consume, and dispose streams for straightforward state management, all with concise Dart code.
Understanding Streams in Dart
A Dart Stream is a sequence of asynchronous data events. You can think of it like a conveyor belt that delivers values over time. There are two main types:
• Single-subscription streams—ideal for one listener.
• Broadcast streams—allow multiple listeners simultaneously.
To produce events, you typically use a StreamController. The controller provides a sink for input and exposes a stream for output.
Key methods:
• controller.add(value) pushes new data.
• controller.close() signals completion.
• Always close your controller to prevent memory leaks.
Building a Reactive Counter with StreamBuilder
Let’s build a simple counter that emits an integer each time a button is pressed.
import 'dart:async';
class CounterBloc {
final _controller = StreamController<int>();
int _count = 0;
Stream<int> get countStream => _controller.stream;
void increment() {
_controller.add(++_count);
}
void dispose() {
_controller.close();
}
}
Here, CounterBloc holds a private counter and exposes a Stream. Calling increment() emits the next value. Always call dispose() when the widget is removed to close the stream.
Displaying Stream Data with StreamBuilder
Flutter’s StreamBuilder widget listens to a stream and rebuilds when new data arrives. Embed it in your UI as follows:
import 'package:flutter/material.dart';
class CounterPage extends StatefulWidget {
@override
_CounterPageState createState() => _CounterPageState();
}
class _CounterPageState extends State<CounterPage> {
final bloc = CounterBloc();
@override
void dispose() {
bloc.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: StreamBuilder<int>(
stream: bloc.countStream,
initialData: 0,
builder: (_, snapshot) => Text(
'Count: ${snapshot.data}',
style: TextStyle(fontSize: 32),
),
),
),
floatingActionButton: FloatingActionButton(
onPressed: bloc.increment,
child: Icon(Icons.add),
),
);
}
}
In this snippet:
• stream binds your stream of events.
• initialData sets the starting UI state.
• The builder callback rebuilds the Text widget whenever the stream emits a new value.
Best Practices for Stream Management
• Always dispose controllers in dispose().
• Choose single-subscription streams for unique flows; use broadcast when multiple widgets need the same data.
• Consider packages like rxdart for advanced operators (map, debounce, merge).
• Structure your code with the BLoC (Business Logic Component) pattern: separate UI from logic, group sinks and streams in one class.
This results in testable, modular components. If you’re streaming data from Firebase, use FirebaseFirestore.instance.collection('items').snapshots() directly in a StreamBuilder for real-time syncing.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Reactive programming with streams in Flutter allows you to build responsive, maintainable applications by treating state changes as events. By encapsulating logic in classes like BLoCs, exposing streams, and consuming them via StreamBuilder, you achieve a clean separation of concerns and seamless UI updates. As you grow more comfortable, explore RxDart operators and higher-level state management libraries that build on streams.
Streamline reactivity with Vibe Studio
Streamline reactivity with Vibe Studio
Streamline reactivity with Vibe Studio
Streamline reactivity with Vibe Studio
Vibe Studio and Steve help you build and manage reactive Flutter apps with live stream data—no boilerplate needed.
Vibe Studio and Steve help you build and manage reactive Flutter apps with live stream data—no boilerplate needed.
Vibe Studio and Steve help you build and manage reactive Flutter apps with live stream data—no boilerplate needed.
Vibe Studio and Steve help you build and manage reactive Flutter apps with live stream data—no boilerplate needed.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






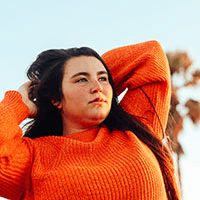



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025