Managing Lists with ListView and GridView in Flutter for Beginners
May 9, 2025



Summary
Summary
Summary
Summary
The article explains how to use ListView, ListView.builder, and GridView.count in Flutter to render scrollable data collections, with guidance on performance, customization, and combining layouts for advanced use cases.
The article explains how to use ListView, ListView.builder, and GridView.count in Flutter to render scrollable data collections, with guidance on performance, customization, and combining layouts for advanced use cases.
The article explains how to use ListView, ListView.builder, and GridView.count in Flutter to render scrollable data collections, with guidance on performance, customization, and combining layouts for advanced use cases.
The article explains how to use ListView, ListView.builder, and GridView.count in Flutter to render scrollable data collections, with guidance on performance, customization, and combining layouts for advanced use cases.
Key insights:
Key insights:
Key insights:
Key insights:
ListView Basics: Best for small, fixed item lists using default constructors.
Efficient Scrolling:
ListView.builder
lazily builds items for large or dynamic data sets.Grid Layouts:
GridView.count
creates multi-column grids with spacing and layout control.Custom Styling: Use padding, separators, and scroll physics to fine-tune behavior.
Advanced UX: Combine lists and grids via
CustomScrollView
and slivers for complex UIs.Refresh Patterns: Add pull-to-refresh using
RefreshIndicator
for modern interactivity.
Introduction
Managing scrollable lists of widgets is a core requirement in many Flutter apps. Flutter offers two primary widgets for this purpose: ListView for vertical (or horizontal) lists and GridView for two-dimensional layouts. In this tutorial, you’ll learn how to:
• Render simple and dynamic lists using ListView and ListView.builder
• Create grid layouts with GridView.count
• Apply basic customizations such as padding, separators, and scroll direction
By the end, you’ll have a clear understanding of how to display collections of data efficiently in Flutter.
ListView Basics
A ListView arranges its children in a scrollable linear array. Use the default constructor when you have a small, fixed number of items.
ListView(
padding: EdgeInsets.all(16),
children: [
ListTile(title: Text('Item 1')),
ListTile(title: Text('Item 2')),
ListTile(title: Text('Item 3')),
ListTile(title: Text('Item 4')),
],
)
Explanation:
padding
adds space around the list content.ListTile
is a convenient widget for list items.
This approach is fine for a handful of widgets, but becomes inefficient for large or dynamic data sources because it builds all children at once.
Dynamic Lists with ListView.builder
For large or dynamically generated lists, use ListView.builder. It lazily builds widgets only as they scroll into view, improving performance.
ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
final item = items[index];
return ListTile(
leading: Icon(Icons.arrow_forward),
title: Text(item.title),
subtitle: Text(item.subtitle),
);
},
)
Key points:
• itemCount specifies the total number of items.
• itemBuilder returns a widget for each index.
• This pattern scales well for hundreds or thousands of list entries.
You can also swap to a horizontal layout:
ListView.builder(
scrollDirection: Axis.horizontal,
itemCount: items.length,
itemBuilder: (c, i) => Container(width: 100, child: Text(items[i])),
)
GridView Basics
To present items in a grid, Flutter provides GridView. A common approach is GridView.count, which lets you specify the number of columns.
GridView.count(
crossAxisCount: 2,
crossAxisSpacing: 8,
mainAxisSpacing: 8,
padding: EdgeInsets.all(16),
children: List.generate(
products.length,
(index) => Card(
child: Column(
children: [
Image.network(products[index].imageUrl),
Text(products[index].name),
],
),
),
),
)
Highlights:
crossAxisCount
: number of columns (e.g., 2 columns).crossAxisSpacing
andmainAxisSpacing
: spacing between grid items.List.generate
creates a list of widgets on demand, similar to ListView.builder.
Advanced Customization
Once you’re comfortable with listview or grid layouts, you can customize further:
• Use ListView.separated to insert dividers between items:
ListView.separated(
itemCount: items.length,
separatorBuilder: (_, __) => Divider(),
itemBuilder: (_, i) => Text(items[i]),
)
• Control scroll physics (BouncingScrollPhysics, ClampingScrollPhysics).
• Wrap children in RefreshIndicator to add pull-to-refresh.
• Combine lists and grids in a CustomScrollView with SliverList and SliverGrid for highly customized scroll effects.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
ListView and GridView are powerful, flexible widgets for displaying data collections in Flutter. Start with the simple ListView constructor for small datasets, then move to ListView.builder for large, dynamic lists. When you need multi-column layouts, GridView.count has you covered. Finally, leverage separators, custom scroll physics, and slivers to achieve advanced designs. Practicing these patterns will help you build efficient, responsive UIs for your next Flutter project.
Introduction
Managing scrollable lists of widgets is a core requirement in many Flutter apps. Flutter offers two primary widgets for this purpose: ListView for vertical (or horizontal) lists and GridView for two-dimensional layouts. In this tutorial, you’ll learn how to:
• Render simple and dynamic lists using ListView and ListView.builder
• Create grid layouts with GridView.count
• Apply basic customizations such as padding, separators, and scroll direction
By the end, you’ll have a clear understanding of how to display collections of data efficiently in Flutter.
ListView Basics
A ListView arranges its children in a scrollable linear array. Use the default constructor when you have a small, fixed number of items.
ListView(
padding: EdgeInsets.all(16),
children: [
ListTile(title: Text('Item 1')),
ListTile(title: Text('Item 2')),
ListTile(title: Text('Item 3')),
ListTile(title: Text('Item 4')),
],
)
Explanation:
padding
adds space around the list content.ListTile
is a convenient widget for list items.
This approach is fine for a handful of widgets, but becomes inefficient for large or dynamic data sources because it builds all children at once.
Dynamic Lists with ListView.builder
For large or dynamically generated lists, use ListView.builder. It lazily builds widgets only as they scroll into view, improving performance.
ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
final item = items[index];
return ListTile(
leading: Icon(Icons.arrow_forward),
title: Text(item.title),
subtitle: Text(item.subtitle),
);
},
)
Key points:
• itemCount specifies the total number of items.
• itemBuilder returns a widget for each index.
• This pattern scales well for hundreds or thousands of list entries.
You can also swap to a horizontal layout:
ListView.builder(
scrollDirection: Axis.horizontal,
itemCount: items.length,
itemBuilder: (c, i) => Container(width: 100, child: Text(items[i])),
)
GridView Basics
To present items in a grid, Flutter provides GridView. A common approach is GridView.count, which lets you specify the number of columns.
GridView.count(
crossAxisCount: 2,
crossAxisSpacing: 8,
mainAxisSpacing: 8,
padding: EdgeInsets.all(16),
children: List.generate(
products.length,
(index) => Card(
child: Column(
children: [
Image.network(products[index].imageUrl),
Text(products[index].name),
],
),
),
),
)
Highlights:
crossAxisCount
: number of columns (e.g., 2 columns).crossAxisSpacing
andmainAxisSpacing
: spacing between grid items.List.generate
creates a list of widgets on demand, similar to ListView.builder.
Advanced Customization
Once you’re comfortable with listview or grid layouts, you can customize further:
• Use ListView.separated to insert dividers between items:
ListView.separated(
itemCount: items.length,
separatorBuilder: (_, __) => Divider(),
itemBuilder: (_, i) => Text(items[i]),
)
• Control scroll physics (BouncingScrollPhysics, ClampingScrollPhysics).
• Wrap children in RefreshIndicator to add pull-to-refresh.
• Combine lists and grids in a CustomScrollView with SliverList and SliverGrid for highly customized scroll effects.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
ListView and GridView are powerful, flexible widgets for displaying data collections in Flutter. Start with the simple ListView constructor for small datasets, then move to ListView.builder for large, dynamic lists. When you need multi-column layouts, GridView.count has you covered. Finally, leverage separators, custom scroll physics, and slivers to achieve advanced designs. Practicing these patterns will help you build efficient, responsive UIs for your next Flutter project.
Build Fast, Scroll Smooth
Build Fast, Scroll Smooth
Build Fast, Scroll Smooth
Build Fast, Scroll Smooth
Design performant list and grid views effortlessly with Vibe Studio’s visual builder and Steve’s AI-powered guidance.
Design performant list and grid views effortlessly with Vibe Studio’s visual builder and Steve’s AI-powered guidance.
Design performant list and grid views effortlessly with Vibe Studio’s visual builder and Steve’s AI-powered guidance.
Design performant list and grid views effortlessly with Vibe Studio’s visual builder and Steve’s AI-powered guidance.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






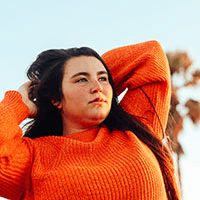



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025