Introduction to Theming: Light and Dark Modes in Flutter
May 7, 2025



Summary
Summary
Summary
Summary
Flutter supports flexible theming with ThemeData for light and dark modes. This guide covers theme setup, applying themes in MaterialApp, user toggles with state management, and customizing widget styles. Paired with Vibe Studio, developers can design and deploy fully themed apps quickly and visually.
Flutter supports flexible theming with ThemeData for light and dark modes. This guide covers theme setup, applying themes in MaterialApp, user toggles with state management, and customizing widget styles. Paired with Vibe Studio, developers can design and deploy fully themed apps quickly and visually.
Flutter supports flexible theming with ThemeData for light and dark modes. This guide covers theme setup, applying themes in MaterialApp, user toggles with state management, and customizing widget styles. Paired with Vibe Studio, developers can design and deploy fully themed apps quickly and visually.
Flutter supports flexible theming with ThemeData for light and dark modes. This guide covers theme setup, applying themes in MaterialApp, user toggles with state management, and customizing widget styles. Paired with Vibe Studio, developers can design and deploy fully themed apps quickly and visually.
Key insights:
Key insights:
Key insights:
Key insights:
ThemeData Setup: Define light and dark themes using brightness, colors, and text styles.
MaterialApp Integration: Use
theme
,darkTheme
, andthemeMode
for dynamic switching.User-Controlled Toggle: Manage
ThemeMode
with Provider for runtime switching.Themed Widgets: Customize components like
AppBar
,Buttons
, andInputs
consistently.Contextual Styling: Access theme properties using
Theme.of(context)
for deeper control.Branding Consistency: Cascading themes ensure a cohesive, accessible UI in all modes.
Introduction
Theming in Flutter is the process of defining a consistent look and feel across your application. By using light and dark modes, you can improve accessibility, reduce eye strain, and provide a polished user experience. Flutter’s built-in support for theming makes it straightforward to switch between color palettes without rewriting widget styles. In this tutorial, you’ll learn how to set up light and dark themes, apply them in your app, and let users toggle between modes. We’ll also show how to customize themed widgets for a fully branded interface.
Setting Up Themes
Start by defining two ThemeData objects: one for light mode and one for dark mode. You can customize colors, text styles, and component themes here.
final ThemeData lightTheme = ThemeData(
brightness: Brightness.light,
primaryColor: Colors.blue,
accentColor: Colors.amber,
scaffoldBackgroundColor: Colors.white,
textTheme: TextTheme(bodyText1: TextStyle(color: Colors.black)),
);
final ThemeData darkTheme = ThemeData(
brightness: Brightness.dark,
primaryColor: Colors.indigo,
accentColor: Colors.tealAccent,
scaffoldBackgroundColor: Colors.black,
textTheme: TextTheme(bodyText1: TextStyle(color: Colors.white)),
);
Key theming properties:
• brightness – sets overall light/dark mode
• primaryColor – main UI color for AppBar, buttons
• accentColor – highlights for floating buttons, switches
• textTheme – default text styling
Applying Themes in MaterialApp
Wrap your root widget in MaterialApp and supply both themes plus a default ThemeMode. ThemeMode.system will follow the device setting, but you can choose light or dark explicitly.
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Theming Demo',
theme: lightTheme,
darkTheme: darkTheme,
themeMode: ThemeMode.system,
home: HomePage(),
);
}
}
With this setup:
• theme – used when ThemeMode.light is active
• darkTheme – used when ThemeMode.dark is active
• themeMode – controls which theme to apply
Switching Between Light and Dark Modes
To let users toggle themes at runtime, maintain a ThemeMode state in a ChangeNotifier or ValueNotifier. Here’s a simple example using ChangeNotifierProvider (from provider package):
class ThemeNotifier extends ChangeNotifier {
ThemeMode _mode = ThemeMode.system;
ThemeMode get mode => _mode;
void toggleMode(bool isDark) {
_mode = isDark ? ThemeMode.dark : ThemeMode.light;
notifyListeners();
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
final notifier = Provider.of<ThemeNotifier>(context);
return Scaffold(
appBar: AppBar(
title: Text('Theming Demo'),
actions: [
Switch(
value: notifier.mode == ThemeMode.dark,
onChanged: notifier.toggleMode,
),
],
),
body: Center(child: Text('Toggle the switch to change theme')),
);
}
}
With provider:
• Wrap MyApp with ChangeNotifierProvider.
• Use Consumer or Provider.of to read ThemeMode and rebuild MaterialApp.
Customizing Themed Widgets
Flutter themes cascade down the widget tree. To override styles for specific components, use the themed constructors:
ElevatedButtonTheme:
elevatedButtonTheme: ElevatedButtonThemeData(
style: ElevatedButton.styleFrom(
primary: Theme.of(context).accentColor,
onPrimary: Colors.black,
),
),
InputDecorationTheme:
inputDecorationTheme: InputDecorationTheme(
border: OutlineInputBorder(),
labelStyle: TextStyle(color: Theme.of(context).primaryColor),
),
AppBarTheme:
appBarTheme: AppBarTheme(
color: Theme.of(context).primaryColor,
elevation: 2,
),
These themed widgets ensure your colors and typography remain consistent when you switch modes. Use Theme.of(context) to access current theme properties inside build methods.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Theming is an essential skill in Flutter development. By defining light and dark ThemeData, wiring them into MaterialApp, and providing a toggle, you deliver a dynamic user experience. Customizing themed widgets further refines your brand consistency. As you build more complex UIs, leverage Flutter’s rich theming capabilities to maintain a coherent design system. Now that you understand the basics of theming, experiment with color schemes, typography, and component themes to create stunning, accessible apps in both light and dark modes.
Introduction
Theming in Flutter is the process of defining a consistent look and feel across your application. By using light and dark modes, you can improve accessibility, reduce eye strain, and provide a polished user experience. Flutter’s built-in support for theming makes it straightforward to switch between color palettes without rewriting widget styles. In this tutorial, you’ll learn how to set up light and dark themes, apply them in your app, and let users toggle between modes. We’ll also show how to customize themed widgets for a fully branded interface.
Setting Up Themes
Start by defining two ThemeData objects: one for light mode and one for dark mode. You can customize colors, text styles, and component themes here.
final ThemeData lightTheme = ThemeData(
brightness: Brightness.light,
primaryColor: Colors.blue,
accentColor: Colors.amber,
scaffoldBackgroundColor: Colors.white,
textTheme: TextTheme(bodyText1: TextStyle(color: Colors.black)),
);
final ThemeData darkTheme = ThemeData(
brightness: Brightness.dark,
primaryColor: Colors.indigo,
accentColor: Colors.tealAccent,
scaffoldBackgroundColor: Colors.black,
textTheme: TextTheme(bodyText1: TextStyle(color: Colors.white)),
);
Key theming properties:
• brightness – sets overall light/dark mode
• primaryColor – main UI color for AppBar, buttons
• accentColor – highlights for floating buttons, switches
• textTheme – default text styling
Applying Themes in MaterialApp
Wrap your root widget in MaterialApp and supply both themes plus a default ThemeMode. ThemeMode.system will follow the device setting, but you can choose light or dark explicitly.
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Theming Demo',
theme: lightTheme,
darkTheme: darkTheme,
themeMode: ThemeMode.system,
home: HomePage(),
);
}
}
With this setup:
• theme – used when ThemeMode.light is active
• darkTheme – used when ThemeMode.dark is active
• themeMode – controls which theme to apply
Switching Between Light and Dark Modes
To let users toggle themes at runtime, maintain a ThemeMode state in a ChangeNotifier or ValueNotifier. Here’s a simple example using ChangeNotifierProvider (from provider package):
class ThemeNotifier extends ChangeNotifier {
ThemeMode _mode = ThemeMode.system;
ThemeMode get mode => _mode;
void toggleMode(bool isDark) {
_mode = isDark ? ThemeMode.dark : ThemeMode.light;
notifyListeners();
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
final notifier = Provider.of<ThemeNotifier>(context);
return Scaffold(
appBar: AppBar(
title: Text('Theming Demo'),
actions: [
Switch(
value: notifier.mode == ThemeMode.dark,
onChanged: notifier.toggleMode,
),
],
),
body: Center(child: Text('Toggle the switch to change theme')),
);
}
}
With provider:
• Wrap MyApp with ChangeNotifierProvider.
• Use Consumer or Provider.of to read ThemeMode and rebuild MaterialApp.
Customizing Themed Widgets
Flutter themes cascade down the widget tree. To override styles for specific components, use the themed constructors:
ElevatedButtonTheme:
elevatedButtonTheme: ElevatedButtonThemeData(
style: ElevatedButton.styleFrom(
primary: Theme.of(context).accentColor,
onPrimary: Colors.black,
),
),
InputDecorationTheme:
inputDecorationTheme: InputDecorationTheme(
border: OutlineInputBorder(),
labelStyle: TextStyle(color: Theme.of(context).primaryColor),
),
AppBarTheme:
appBarTheme: AppBarTheme(
color: Theme.of(context).primaryColor,
elevation: 2,
),
These themed widgets ensure your colors and typography remain consistent when you switch modes. Use Theme.of(context) to access current theme properties inside build methods.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Theming is an essential skill in Flutter development. By defining light and dark ThemeData, wiring them into MaterialApp, and providing a toggle, you deliver a dynamic user experience. Customizing themed widgets further refines your brand consistency. As you build more complex UIs, leverage Flutter’s rich theming capabilities to maintain a coherent design system. Now that you understand the basics of theming, experiment with color schemes, typography, and component themes to create stunning, accessible apps in both light and dark modes.
Design Beautiful, Themed Flutter Apps
Design Beautiful, Themed Flutter Apps
Design Beautiful, Themed Flutter Apps
Design Beautiful, Themed Flutter Apps
Vibe Studio helps you build and manage fully branded Flutter apps—light or dark—without writing code.
Vibe Studio helps you build and manage fully branded Flutter apps—light or dark—without writing code.
Vibe Studio helps you build and manage fully branded Flutter apps—light or dark—without writing code.
Vibe Studio helps you build and manage fully branded Flutter apps—light or dark—without writing code.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






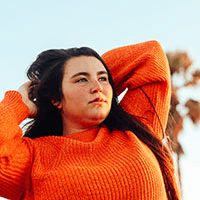



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025