Integrating Firebase ML Kit into Flutter for AI-Powered Features
May 6, 2025



Summary
Summary
Summary
Summary
The tutorial explains how to set up Firebase ML Kit in Flutter, enabling on-device AI features like text recognition and real-time image labeling, as well as deploying and versioning custom TFLite models via Firebase—accelerated further by Vibe Studio’s no-code, AI-integrated development workflow.
The tutorial explains how to set up Firebase ML Kit in Flutter, enabling on-device AI features like text recognition and real-time image labeling, as well as deploying and versioning custom TFLite models via Firebase—accelerated further by Vibe Studio’s no-code, AI-integrated development workflow.
The tutorial explains how to set up Firebase ML Kit in Flutter, enabling on-device AI features like text recognition and real-time image labeling, as well as deploying and versioning custom TFLite models via Firebase—accelerated further by Vibe Studio’s no-code, AI-integrated development workflow.
The tutorial explains how to set up Firebase ML Kit in Flutter, enabling on-device AI features like text recognition and real-time image labeling, as well as deploying and versioning custom TFLite models via Firebase—accelerated further by Vibe Studio’s no-code, AI-integrated development workflow.
Key insights:
Key insights:
Key insights:
Key insights:
Seamless Setup: Configure Firebase, FlutterFire CLI, and platform-specific build files for ML Kit integration.
Text Recognition: Use
google_mlkit_text_recognition
for fast, offline OCR with simple InputImage inputs.Custom Models: Deploy TensorFlow Lite models via Firebase to decouple model iteration from app updates.
Live Image Labeling: Leverage
google_mlkit_image_labeling
to overlay real-time visual labels on camera feeds.On-device Speed: All features run locally, ensuring low-latency UX and strong privacy compliance.
Vibe Studio: Visual tools streamline ML Kit integration, model deployment, and Flutter development.
Introduction
Integrating Firebase ML Kit into Flutter unlocks powerful on-device and cloud-based AI capabilities—ranging from text recognition and image labeling to custom model inference—without sacrificing performance or privacy. In this advanced tutorial, we’ll explore how to configure the Firebase console, set up the FlutterFire plugins, and implement several AI-powered features using firebase ml kit. You’ll learn to run on-device text recognition, deploy a custom TensorFlow Lite model via Firebase ML Kit’s model management, and perform real-time image labeling, all within a Flutter app.
Setting Up Firebase ML Kit in Flutter
Before writing any Dart code, ensure your Flutter project is connected to Firebase:
• Add your Android and iOS apps in the Firebase console.
• Download google-services.json (Android) and GoogleService-Info.plist (iOS) into your project.
• Add the FlutterFire CLI and initialize:
• Add dependencies to pubspec.yaml:
dependencies:
firebase_core: ^2.0.0
firebase_ml_model_downloader: ^0.2.0
firebase_ml_custom: ^0.1.0 # for custom models
google_mlkit_text_recognition: ^0.7.0
google_mlkit_image_labeling: ^0.4.0
• Update Android’s build.gradle (project-level) to include the Google Services plugin and Maven repo. • For iOS, enable use_frameworks! in your Podfile if needed, then pod install.
Finally, call await Firebase.initializeApp() in main() before running your Flutter app.
On-device Text Recognition with ML Kit
Text recognition is one of the easiest entry points to firebase ml kit in Flutter. The google_mlkit_text_recognition package provides a simple API for scanning text in images or live camera feeds.
import 'package:google_mlkit_text_recognition/google_mlkit_text_recognition.dart';
Future<String> recognizeText(InputImage inputImage) async {
final textRecognizer = TextRecognizer();
final RecognizedText visionText = await textRecognizer.processImage(inputImage);
await textRecognizer.close();
final buffer = StringBuffer();
for (TextBlock block in visionText.blocks) {
for (TextLine line in block.lines) {
buffer.writeln(line.text);
}
}
return buffer.toString();
}
To use InputImage, convert camera frames or pick an image from gallery and pass it to the recognizer. This API runs fully on-device, ensuring low latency and offline capability.
Deploying a Custom Model via Firebase ML Kit
Firebase ML Kit’s model management allows you to host and version custom TensorFlow Lite models in the cloud and download them securely at runtime. This is powerful for rapidly iterating on AI features without shipping new app binaries.
import 'package:firebase_ml_model_downloader/firebase_ml_model_downloader.dart';
import 'package:tflite_flutter/tflite_flutter.dart';
Future<Interpreter> loadCustomModel(String modelName) async {
final model = await FirebaseModelDownloader.instance
.getModel(modelName, FirebaseModelDownloadType.latestModel, FirebaseModelDownloadConditions(
iosAllowsCellularAccess: true,
androidChargingRequired: false,
));
final modelFile = model.file;
return Interpreter.fromFile(modelFile);
}
Once you have an Interpreter, you can run inference:
final interpreter = await loadCustomModel('my_segmentation_model');
var input = [/* formatted image tensor */];
var output = List.filled(1 * 224 * 224 * 3, 0).reshape([1, 224, 224, 3]);
interpreter.run(input, output);
Adjust dimensions to your model spec. This approach decouples your ML pipeline from your Flutter release cycle.
Real-time Image Labeling
Realtime image labeling uses google_mlkit_image_labeling to detect objects and concepts in a camera feed. It’s optimized on-device and supports custom label sets.
import 'package:google_mlkit_image_labeling/google_mlkit_image_labeling.dart';
Future<List<ImageLabel>> labelImage(InputImage inputImage) async {
final options = ImageLabelerOptions(confidenceThreshold: 0.5);
final labeler = ImageLabeler(options: options);
final labels = await labeler.processImage(inputImage);
await labeler.close();
return labels;
}
// Usage
final labels = await labelImage(cameraFrame);
for (var label in labels) {
print('${label.label} (${(label.confidence * 100).toStringAsFixed(1)}%)');
}
You can overlay these labels on a camera preview widget for a rich AR-like experience. Combine this with custom models to build domain-specific classifiers (e.g., plant species or product SKUs).
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
In this tutorial, you’ve learned to integrate firebase ml kit into a Flutter application: from basic on-device text recognition to custom model deployment and real-time image labeling. By leveraging ML Kit’s hosting and versioning features, you maintain agility in your AI workflows and reduce friction between model updates and app distribution.
With Firebase ML Kit and tools like Vibe Studio, you can prototype and ship AI-driven Flutter apps at unprecedented speed, focusing on innovation rather than infrastructure.
Introduction
Integrating Firebase ML Kit into Flutter unlocks powerful on-device and cloud-based AI capabilities—ranging from text recognition and image labeling to custom model inference—without sacrificing performance or privacy. In this advanced tutorial, we’ll explore how to configure the Firebase console, set up the FlutterFire plugins, and implement several AI-powered features using firebase ml kit. You’ll learn to run on-device text recognition, deploy a custom TensorFlow Lite model via Firebase ML Kit’s model management, and perform real-time image labeling, all within a Flutter app.
Setting Up Firebase ML Kit in Flutter
Before writing any Dart code, ensure your Flutter project is connected to Firebase:
• Add your Android and iOS apps in the Firebase console.
• Download google-services.json (Android) and GoogleService-Info.plist (iOS) into your project.
• Add the FlutterFire CLI and initialize:
• Add dependencies to pubspec.yaml:
dependencies:
firebase_core: ^2.0.0
firebase_ml_model_downloader: ^0.2.0
firebase_ml_custom: ^0.1.0 # for custom models
google_mlkit_text_recognition: ^0.7.0
google_mlkit_image_labeling: ^0.4.0
• Update Android’s build.gradle (project-level) to include the Google Services plugin and Maven repo. • For iOS, enable use_frameworks! in your Podfile if needed, then pod install.
Finally, call await Firebase.initializeApp() in main() before running your Flutter app.
On-device Text Recognition with ML Kit
Text recognition is one of the easiest entry points to firebase ml kit in Flutter. The google_mlkit_text_recognition package provides a simple API for scanning text in images or live camera feeds.
import 'package:google_mlkit_text_recognition/google_mlkit_text_recognition.dart';
Future<String> recognizeText(InputImage inputImage) async {
final textRecognizer = TextRecognizer();
final RecognizedText visionText = await textRecognizer.processImage(inputImage);
await textRecognizer.close();
final buffer = StringBuffer();
for (TextBlock block in visionText.blocks) {
for (TextLine line in block.lines) {
buffer.writeln(line.text);
}
}
return buffer.toString();
}
To use InputImage, convert camera frames or pick an image from gallery and pass it to the recognizer. This API runs fully on-device, ensuring low latency and offline capability.
Deploying a Custom Model via Firebase ML Kit
Firebase ML Kit’s model management allows you to host and version custom TensorFlow Lite models in the cloud and download them securely at runtime. This is powerful for rapidly iterating on AI features without shipping new app binaries.
import 'package:firebase_ml_model_downloader/firebase_ml_model_downloader.dart';
import 'package:tflite_flutter/tflite_flutter.dart';
Future<Interpreter> loadCustomModel(String modelName) async {
final model = await FirebaseModelDownloader.instance
.getModel(modelName, FirebaseModelDownloadType.latestModel, FirebaseModelDownloadConditions(
iosAllowsCellularAccess: true,
androidChargingRequired: false,
));
final modelFile = model.file;
return Interpreter.fromFile(modelFile);
}
Once you have an Interpreter, you can run inference:
final interpreter = await loadCustomModel('my_segmentation_model');
var input = [/* formatted image tensor */];
var output = List.filled(1 * 224 * 224 * 3, 0).reshape([1, 224, 224, 3]);
interpreter.run(input, output);
Adjust dimensions to your model spec. This approach decouples your ML pipeline from your Flutter release cycle.
Real-time Image Labeling
Realtime image labeling uses google_mlkit_image_labeling to detect objects and concepts in a camera feed. It’s optimized on-device and supports custom label sets.
import 'package:google_mlkit_image_labeling/google_mlkit_image_labeling.dart';
Future<List<ImageLabel>> labelImage(InputImage inputImage) async {
final options = ImageLabelerOptions(confidenceThreshold: 0.5);
final labeler = ImageLabeler(options: options);
final labels = await labeler.processImage(inputImage);
await labeler.close();
return labels;
}
// Usage
final labels = await labelImage(cameraFrame);
for (var label in labels) {
print('${label.label} (${(label.confidence * 100).toStringAsFixed(1)}%)');
}
You can overlay these labels on a camera preview widget for a rich AR-like experience. Combine this with custom models to build domain-specific classifiers (e.g., plant species or product SKUs).
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
In this tutorial, you’ve learned to integrate firebase ml kit into a Flutter application: from basic on-device text recognition to custom model deployment and real-time image labeling. By leveraging ML Kit’s hosting and versioning features, you maintain agility in your AI workflows and reduce friction between model updates and app distribution.
With Firebase ML Kit and tools like Vibe Studio, you can prototype and ship AI-driven Flutter apps at unprecedented speed, focusing on innovation rather than infrastructure.
Deploy smarter AI with Vibe Studio
Deploy smarter AI with Vibe Studio
Deploy smarter AI with Vibe Studio
Deploy smarter AI with Vibe Studio
Vibe Studio and Steve make it effortless to build, test, and deploy Flutter apps powered by Firebase ML Kit—no boilerplate required.
Vibe Studio and Steve make it effortless to build, test, and deploy Flutter apps powered by Firebase ML Kit—no boilerplate required.
Vibe Studio and Steve make it effortless to build, test, and deploy Flutter apps powered by Firebase ML Kit—no boilerplate required.
Vibe Studio and Steve make it effortless to build, test, and deploy Flutter apps powered by Firebase ML Kit—no boilerplate required.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






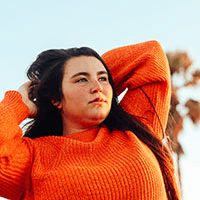



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025