Integrating Firebase Authentication in Your Flutter App
May 12, 2025



Summary
Summary
Summary
Summary
Firebase Authentication provides a secure, scalable way to handle user sign-in in Flutter apps. This guide covers setting up a Firebase project, configuring iOS and Android apps, integrating firebase_auth, and building sign-up/sign-in UIs. Vibe Studio supports this process with no-code tools for fast, Firebase-integrated Flutter development.
Firebase Authentication provides a secure, scalable way to handle user sign-in in Flutter apps. This guide covers setting up a Firebase project, configuring iOS and Android apps, integrating firebase_auth, and building sign-up/sign-in UIs. Vibe Studio supports this process with no-code tools for fast, Firebase-integrated Flutter development.
Firebase Authentication provides a secure, scalable way to handle user sign-in in Flutter apps. This guide covers setting up a Firebase project, configuring iOS and Android apps, integrating firebase_auth, and building sign-up/sign-in UIs. Vibe Studio supports this process with no-code tools for fast, Firebase-integrated Flutter development.
Firebase Authentication provides a secure, scalable way to handle user sign-in in Flutter apps. This guide covers setting up a Firebase project, configuring iOS and Android apps, integrating firebase_auth, and building sign-up/sign-in UIs. Vibe Studio supports this process with no-code tools for fast, Firebase-integrated Flutter development.
Key insights:
Key insights:
Key insights:
Key insights:
Firebase Setup: Register platforms and download config files to connect your app.
Provider Enablement: Activate Email/Password and other auth methods in Firebase Console.
Auth Integration: Use
firebase_auth
to handle user registration and login logic.Reactive Routing: Automatically switch screens based on authentication state changes.
Error Handling: Catch
FirebaseAuthException
for user-friendly feedback.Vibe Studio Simplicity: Build and manage Firebase authentication flows visually with AI assistance.
Introduction
Integrating Firebase Authentication in your Flutter app unlocks secure user sign-in, account management, and session persistence with minimal backend work. In this tutorial, we’ll cover how to set up Flutter Firebase Auth, configure your app, and implement basic email/password sign-in functionality. You’ll learn how to leverage Firebase Auth in Flutter and see a code-forward approach to get you up and running quickly.
Setting Up Your Firebase Project
Before writing any Flutter code, you need a Firebase project:
• Go to the Firebase Console and click Add project.
• Enter a project name and accept Google Analytics settings as needed.
• In your Firebase project overview, select Add app and choose the Flutter icon.
• Register your iOS and Android apps with their respective bundle IDs.
• Download the generated google-services.json (Android) and GoogleService-Info.plist (iOS).
Place google-services.json in android/app/ and GoogleService-Info.plist in ios/Runner/. These files link your Flutter app to your Firebase backend.
Next, enable Authentication providers:
In the Firebase Console, navigate to Authentication > Sign-in method.
Enable Email/Password (and any other providers you need).
Adding Firebase to Your Flutter App
To integrate Flutter Firebase Auth, add these dependencies in your pubspec.yaml:
dependencies:
flutter:
sdk: flutter
firebase_core: ^2.0.0
firebase_auth
Run flutter pub get. Then, initialize Firebase at app startup. In main.dart:
import 'package:firebase_core/firebase_core.dart';
import 'package:flutter/material.dart';
import 'app.dart'; // Your root widget
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(const MyApp());
}
This ensures the Firebase SDK is ready before your Flutter app runs. Now you have the foundation for Firebase Auth in Flutter.
Building the Authentication Logic
With Flutter Firebase Auth integrated, let’s implement sign-up and sign-in. You’ll use the firebase_auth package’s FirebaseAuth instance.
Create an AuthService class:
import 'package:firebase_auth/firebase_auth.dart';
class AuthService {
final FirebaseAuth _auth = FirebaseAuth.instance;
Future<User?> signUp(String email, String password) async {
final cred = await _auth.createUserWithEmailAndPassword(
email: email,
password: password,
);
return cred.user;
}
Future<User?> signIn(String email, String password) async {
final cred = await _auth.signInWithEmailAndPassword(
email: email,
password: password,
);
return cred.user;
}
Future<void> signOut() => _auth.signOut();
Stream<User?> get userChanges => _auth.userChanges();
}
This service exposes methods to register, log in, and observe authentication state changes. It’s your central point for Flutter authentication with Firebase.
Creating the Authentication UI
Now you need screens for email/password registration and login. Using Flutter’s TextFormField and ElevatedButton:
class SignInScreen extends StatefulWidget {
@override
_SignInScreenState createState() => _SignInScreenState();
}
class _SignInScreenState extends State<SignInScreen> {
final _emailCtrl = TextEditingController();
final _pwdCtrl = TextEditingController();
final _auth = AuthService();
@override
Widget build(BuildContext context) {
return Scaffold(
body: Padding(
padding: const EdgeInsets.all(16),
child: Column(
children: [
TextFormField(controller: _emailCtrl, decoration: const InputDecoration(labelText: 'Email')),
TextFormField(controller: _pwdCtrl, decoration: const InputDecoration(labelText: 'Password'), obscureText: true),
ElevatedButton(
onPressed: () async {
final user = await _auth.signIn(_emailCtrl.text, _pwdCtrl.text);
if (user != null) Navigator.pushReplacementNamed(context, '/home');
},
child: const Text('Sign In'),
),
ElevatedButton(
onPressed: () => Navigator.pushNamed(context, '/signup'),
child: const Text('Create Account'),
),
],
),
),
);
}
}
Repeat similarly for a SignUpScreen that uses _auth.signUp(...). Finally, in your MaterialApp, direct routes based on AuthService().userChanges:
return StreamBuilder<User?>(
stream: AuthService().userChanges,
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.active) {
return snapshot.data != null ? const HomeScreen() : SignInScreen();
}
return const CircularProgressIndicator();
},
);
This automatically shows the sign-in screen when no user is signed in and your app’s home screen when authentication succeeds.
Testing and Debugging
• Use the Firebase Console’s Authentication tab to view registered users.
• On Android, run flutter run and test email/password sign-up and sign-in flows.
• For iOS, ensure you’ve added GoogleService-Info.plist and configured the Podfile.
• Catch and display errors from FirebaseAuthException for improved UX.
Example error handling in sign-in:
try {
await _auth.signIn(email, password);
} on FirebaseAuthException catch (e) {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text(e.message ?? 'Sign-in failed')),
);
}
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
You’ve now integrated Flutter Firebase Auth into your app, created sign-up and sign-in flows, and handled user state changes. From here, you can expand to social logins, phone authentication, and email verification using Firebase’s rich authentication suite.
With this foundation, you’re ready to build secure, scalable Flutter applications using Flutter Firebase Auth. Experiment with more providers, custom user data in Firestore, and advanced session management to fit your app’s needs.
Introduction
Integrating Firebase Authentication in your Flutter app unlocks secure user sign-in, account management, and session persistence with minimal backend work. In this tutorial, we’ll cover how to set up Flutter Firebase Auth, configure your app, and implement basic email/password sign-in functionality. You’ll learn how to leverage Firebase Auth in Flutter and see a code-forward approach to get you up and running quickly.
Setting Up Your Firebase Project
Before writing any Flutter code, you need a Firebase project:
• Go to the Firebase Console and click Add project.
• Enter a project name and accept Google Analytics settings as needed.
• In your Firebase project overview, select Add app and choose the Flutter icon.
• Register your iOS and Android apps with their respective bundle IDs.
• Download the generated google-services.json (Android) and GoogleService-Info.plist (iOS).
Place google-services.json in android/app/ and GoogleService-Info.plist in ios/Runner/. These files link your Flutter app to your Firebase backend.
Next, enable Authentication providers:
In the Firebase Console, navigate to Authentication > Sign-in method.
Enable Email/Password (and any other providers you need).
Adding Firebase to Your Flutter App
To integrate Flutter Firebase Auth, add these dependencies in your pubspec.yaml:
dependencies:
flutter:
sdk: flutter
firebase_core: ^2.0.0
firebase_auth
Run flutter pub get. Then, initialize Firebase at app startup. In main.dart:
import 'package:firebase_core/firebase_core.dart';
import 'package:flutter/material.dart';
import 'app.dart'; // Your root widget
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(const MyApp());
}
This ensures the Firebase SDK is ready before your Flutter app runs. Now you have the foundation for Firebase Auth in Flutter.
Building the Authentication Logic
With Flutter Firebase Auth integrated, let’s implement sign-up and sign-in. You’ll use the firebase_auth package’s FirebaseAuth instance.
Create an AuthService class:
import 'package:firebase_auth/firebase_auth.dart';
class AuthService {
final FirebaseAuth _auth = FirebaseAuth.instance;
Future<User?> signUp(String email, String password) async {
final cred = await _auth.createUserWithEmailAndPassword(
email: email,
password: password,
);
return cred.user;
}
Future<User?> signIn(String email, String password) async {
final cred = await _auth.signInWithEmailAndPassword(
email: email,
password: password,
);
return cred.user;
}
Future<void> signOut() => _auth.signOut();
Stream<User?> get userChanges => _auth.userChanges();
}
This service exposes methods to register, log in, and observe authentication state changes. It’s your central point for Flutter authentication with Firebase.
Creating the Authentication UI
Now you need screens for email/password registration and login. Using Flutter’s TextFormField and ElevatedButton:
class SignInScreen extends StatefulWidget {
@override
_SignInScreenState createState() => _SignInScreenState();
}
class _SignInScreenState extends State<SignInScreen> {
final _emailCtrl = TextEditingController();
final _pwdCtrl = TextEditingController();
final _auth = AuthService();
@override
Widget build(BuildContext context) {
return Scaffold(
body: Padding(
padding: const EdgeInsets.all(16),
child: Column(
children: [
TextFormField(controller: _emailCtrl, decoration: const InputDecoration(labelText: 'Email')),
TextFormField(controller: _pwdCtrl, decoration: const InputDecoration(labelText: 'Password'), obscureText: true),
ElevatedButton(
onPressed: () async {
final user = await _auth.signIn(_emailCtrl.text, _pwdCtrl.text);
if (user != null) Navigator.pushReplacementNamed(context, '/home');
},
child: const Text('Sign In'),
),
ElevatedButton(
onPressed: () => Navigator.pushNamed(context, '/signup'),
child: const Text('Create Account'),
),
],
),
),
);
}
}
Repeat similarly for a SignUpScreen that uses _auth.signUp(...). Finally, in your MaterialApp, direct routes based on AuthService().userChanges:
return StreamBuilder<User?>(
stream: AuthService().userChanges,
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.active) {
return snapshot.data != null ? const HomeScreen() : SignInScreen();
}
return const CircularProgressIndicator();
},
);
This automatically shows the sign-in screen when no user is signed in and your app’s home screen when authentication succeeds.
Testing and Debugging
• Use the Firebase Console’s Authentication tab to view registered users.
• On Android, run flutter run and test email/password sign-up and sign-in flows.
• For iOS, ensure you’ve added GoogleService-Info.plist and configured the Podfile.
• Catch and display errors from FirebaseAuthException for improved UX.
Example error handling in sign-in:
try {
await _auth.signIn(email, password);
} on FirebaseAuthException catch (e) {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text(e.message ?? 'Sign-in failed')),
);
}
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
You’ve now integrated Flutter Firebase Auth into your app, created sign-up and sign-in flows, and handled user state changes. From here, you can expand to social logins, phone authentication, and email verification using Firebase’s rich authentication suite.
With this foundation, you’re ready to build secure, scalable Flutter applications using Flutter Firebase Auth. Experiment with more providers, custom user data in Firestore, and advanced session management to fit your app’s needs.
Secure Sign-In with Vibe Studio
Secure Sign-In with Vibe Studio
Secure Sign-In with Vibe Studio
Secure Sign-In with Vibe Studio
Integrate Firebase Auth faster and build full-stack login flows visually using Vibe Studio’s no-code Flutter platform.
Integrate Firebase Auth faster and build full-stack login flows visually using Vibe Studio’s no-code Flutter platform.
Integrate Firebase Auth faster and build full-stack login flows visually using Vibe Studio’s no-code Flutter platform.
Integrate Firebase Auth faster and build full-stack login flows visually using Vibe Studio’s no-code Flutter platform.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






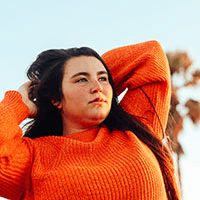



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025