Implementing WebSockets for Real‑time Updates in Flutter
May 7, 2025



Summary
Summary
Summary
Summary
Websockets enable efficient, bidirectional communication in Flutter apps, ideal for chat, dashboards, and live updates. This guide details setup, message handling, UI integration, and error management. When combined with Vibe Studio’s no-code tools, developers can rapidly build and deploy real-time apps with Firebase backends.
Websockets enable efficient, bidirectional communication in Flutter apps, ideal for chat, dashboards, and live updates. This guide details setup, message handling, UI integration, and error management. When combined with Vibe Studio’s no-code tools, developers can rapidly build and deploy real-time apps with Firebase backends.
Websockets enable efficient, bidirectional communication in Flutter apps, ideal for chat, dashboards, and live updates. This guide details setup, message handling, UI integration, and error management. When combined with Vibe Studio’s no-code tools, developers can rapidly build and deploy real-time apps with Firebase backends.
Websockets enable efficient, bidirectional communication in Flutter apps, ideal for chat, dashboards, and live updates. This guide details setup, message handling, UI integration, and error management. When combined with Vibe Studio’s no-code tools, developers can rapidly build and deploy real-time apps with Firebase backends.
Key insights:
Key insights:
Key insights:
Key insights:
Websocket Basics: Provides full-duplex communication using
web_socket_channel
across platforms.Message Handling: Decode streams, validate data, and handle disconnections with reconnection logic.
Sending & Heartbeats: Use
sink.add()
for outbound messages and periodic pings to keep connections alive.Flutter Integration: Bind real-time data to widgets via
StreamBuilder
and state management tools.Error Resilience: Implement reconnection strategies, sanitize data, and monitor network conditions.
Production Readiness: Use secure endpoints, renew tokens, and profile performance under load.
Introduction
Real-time features are essential in modern apps for chat, live dashboards, and collaborative tools. Websockets offer a full-duplex communication channel over a single TCP connection, enabling low-latency, bidirectional data transfer. In this tutorial, you’ll learn how to implement websockets in Flutter to push updates instantly, handle incoming messages, and integrate streaming data with your UI.
Setting Up a WebSocket Connection
Add the web_socket_channel package to your pubspec.yaml:
dependencies:
flutter:
sdk: flutter
web_socket_channel
Import and open a channel to your server endpoint:
import 'package:web_socket_channel/io.dart';
final channel = IOWebSocketChannel.connect(
Uri.parse('wss://yourserver.com/socket'),
headers: {'Authorization': 'Bearer $token'},
);
This establishes a websocket connection. Use secure wss:// for production. The IOWebSocketChannel works on mobile and desktop; use WebSocketChannel.connect for web.
Handling Incoming Messages
Once connected, listen to the channel’s stream. Typically, servers send JSON payloads, so decode accordingly:
channel.stream.listen(
(message) {
final data = jsonDecode(message as String);
// process data, e.g., update a model or emit an event
print('New update: ${data['payload']}');
},
onError: (error) => print('WebSocket error: $error'),
onDone: () => print('Connection closed'),
);
Best practices for websocket streams:
Decode partial messages if using binary frames
Validate schema or use a protocol (e.g., Socket.IO)
Gracefully handle
onDone
for reconnection logic
Sending Messages and Heartbeat
To push data from the client, call sink.add(). If your server expects JSON:
void sendUpdate(Map<String, dynamic> payload) {
final message = jsonEncode({'type': 'update', 'payload': payload});
channel.sink.add(message);
}
Maintain a heartbeat or ping/pong to keep idle connections alive. Some servers close stale sockets after 30–60 seconds. Implement a periodic ping:
Timer.periodic(Duration(seconds: 20), (_) {
channel.sink.add(jsonEncode({'type': 'ping'}));
});
Always call channel.sink.close() when disposing to free resources.
Integrating with Flutter Widgets
Use StreamBuilder to bind real-time data to your UI. For example, a simple live feed:
StreamBuilder(
stream: channel.stream,
builder: (context, snapshot) {
if (snapshot.hasError) return Text('Error: ${snapshot.error}');
if (!snapshot.hasData) return Text('Connecting...');
final data = jsonDecode(snapshot.data as String);
return ListTile(
title: Text(data['user']),
subtitle: Text(data['message']),
);
},
);
Combine this with ListView to render a chat history. For state management, integrate with Provider, Riverpod, or Bloc to broadcast updates across multiple widgets.
Best Practices and Error Handling
Reconnect with exponential backoff when
onDone
or errors occur.Use authentication tokens and renew them before expiration.
Sanitize incoming data to prevent injection attacks.
Monitor network status and pause the socket when offline.
Profile memory and CPU if handling high-frequency messages.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing websockets in Flutter unlocks true real-time capabilities for chat apps, live dashboards, multiplayer games, and more. By establishing a robust connection, handling messages with safety checks, and binding streams to widgets, you achieve seamless, low-latency updates. Start experimenting with websockets today to enhance user engagement and reactive interfaces in your Flutter projects.
Introduction
Real-time features are essential in modern apps for chat, live dashboards, and collaborative tools. Websockets offer a full-duplex communication channel over a single TCP connection, enabling low-latency, bidirectional data transfer. In this tutorial, you’ll learn how to implement websockets in Flutter to push updates instantly, handle incoming messages, and integrate streaming data with your UI.
Setting Up a WebSocket Connection
Add the web_socket_channel package to your pubspec.yaml:
dependencies:
flutter:
sdk: flutter
web_socket_channel
Import and open a channel to your server endpoint:
import 'package:web_socket_channel/io.dart';
final channel = IOWebSocketChannel.connect(
Uri.parse('wss://yourserver.com/socket'),
headers: {'Authorization': 'Bearer $token'},
);
This establishes a websocket connection. Use secure wss:// for production. The IOWebSocketChannel works on mobile and desktop; use WebSocketChannel.connect for web.
Handling Incoming Messages
Once connected, listen to the channel’s stream. Typically, servers send JSON payloads, so decode accordingly:
channel.stream.listen(
(message) {
final data = jsonDecode(message as String);
// process data, e.g., update a model or emit an event
print('New update: ${data['payload']}');
},
onError: (error) => print('WebSocket error: $error'),
onDone: () => print('Connection closed'),
);
Best practices for websocket streams:
Decode partial messages if using binary frames
Validate schema or use a protocol (e.g., Socket.IO)
Gracefully handle
onDone
for reconnection logic
Sending Messages and Heartbeat
To push data from the client, call sink.add(). If your server expects JSON:
void sendUpdate(Map<String, dynamic> payload) {
final message = jsonEncode({'type': 'update', 'payload': payload});
channel.sink.add(message);
}
Maintain a heartbeat or ping/pong to keep idle connections alive. Some servers close stale sockets after 30–60 seconds. Implement a periodic ping:
Timer.periodic(Duration(seconds: 20), (_) {
channel.sink.add(jsonEncode({'type': 'ping'}));
});
Always call channel.sink.close() when disposing to free resources.
Integrating with Flutter Widgets
Use StreamBuilder to bind real-time data to your UI. For example, a simple live feed:
StreamBuilder(
stream: channel.stream,
builder: (context, snapshot) {
if (snapshot.hasError) return Text('Error: ${snapshot.error}');
if (!snapshot.hasData) return Text('Connecting...');
final data = jsonDecode(snapshot.data as String);
return ListTile(
title: Text(data['user']),
subtitle: Text(data['message']),
);
},
);
Combine this with ListView to render a chat history. For state management, integrate with Provider, Riverpod, or Bloc to broadcast updates across multiple widgets.
Best Practices and Error Handling
Reconnect with exponential backoff when
onDone
or errors occur.Use authentication tokens and renew them before expiration.
Sanitize incoming data to prevent injection attacks.
Monitor network status and pause the socket when offline.
Profile memory and CPU if handling high-frequency messages.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing websockets in Flutter unlocks true real-time capabilities for chat apps, live dashboards, multiplayer games, and more. By establishing a robust connection, handling messages with safety checks, and binding streams to widgets, you achieve seamless, low-latency updates. Start experimenting with websockets today to enhance user engagement and reactive interfaces in your Flutter projects.
Launch Real-Time Flutter Apps Instantly
Launch Real-Time Flutter Apps Instantly
Launch Real-Time Flutter Apps Instantly
Launch Real-Time Flutter Apps Instantly
Vibe Studio makes it easy to build and deploy websocket-powered Flutter apps—no coding required.
Vibe Studio makes it easy to build and deploy websocket-powered Flutter apps—no coding required.
Vibe Studio makes it easy to build and deploy websocket-powered Flutter apps—no coding required.
Vibe Studio makes it easy to build and deploy websocket-powered Flutter apps—no coding required.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






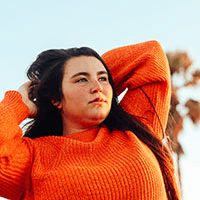



The Jacx Office: 16-120
2807 Jackson Ave
Queens NY 11101, United States


The Jacx Office: 16-120
2807 Jackson Ave
Queens NY 11101, United States


The Jacx Office: 16-120
2807 Jackson Ave
Queens NY 11101, United States


The Jacx Office: 16-120
2807 Jackson Ave
Queens NY 11101, United States


The Jacx Office: 16-120
2807 Jackson Ave
Queens NY 11101, United States


The Jacx Office: 16-120
2807 Jackson Ave
Queens NY 11101, United States