Implementing Push Notifications in Flutter Using Firebase Cloud Messaging
May 6, 2025



Summary
Summary
Summary
Summary
Integrate Firebase Cloud Messaging into your Flutter app to send real-time push notifications. Learn to configure FCM for Android and iOS, handle messages in all app states, and explore advanced techniques like topic subscriptions and silent payloads to boost user engagement.
Integrate Firebase Cloud Messaging into your Flutter app to send real-time push notifications. Learn to configure FCM for Android and iOS, handle messages in all app states, and explore advanced techniques like topic subscriptions and silent payloads to boost user engagement.
Integrate Firebase Cloud Messaging into your Flutter app to send real-time push notifications. Learn to configure FCM for Android and iOS, handle messages in all app states, and explore advanced techniques like topic subscriptions and silent payloads to boost user engagement.
Integrate Firebase Cloud Messaging into your Flutter app to send real-time push notifications. Learn to configure FCM for Android and iOS, handle messages in all app states, and explore advanced techniques like topic subscriptions and silent payloads to boost user engagement.
Key insights:
Key insights:
Key insights:
Key insights:
Cross-Platform Setup: Configure FCM for both Android and iOS using Firebase console and platform-specific setup files.
Notification Handling: Use listeners to manage messages in foreground, background, and terminated app states.
Permissions & Initialization: Request permissions and initialize Firebase in
main.dart
to start receiving messages.Advanced Messaging: Use topic subscriptions, data-only messages, and Android notification channels for deeper control.
Security Best Practices: Protect API keys, validate device tokens, and secure endpoints with Firebase Authentication.
Full Engagement Pipeline: FCM enables timely, personalized communication that enhances retention and user experience.
Introduction
Push notifications are crucial for user engagement in modern mobile apps. By integrating firebase cloud messaging (FCM) with your Flutter project, you can send targeted messages, updates, and alerts to users in real time. This tutorial walks through setting up push notifications in Flutter using Firebase Cloud Messaging, covering both basic configuration and handling background messages. You should have a Flutter project ready and access to a Firebase console.
Setting up Firebase Project
Create a Firebase project at https://console.firebase.google.com.
For Android:
• Register your app with the Android package name.
• Downloadgoogle-services.json
and place it inandroid/app/
.
• Add the Firebase Gradle plugin toandroid/build.gradle
:
dependencies { classpath 'com.google.gms:google-services:4.3.10'
• Apply the plugin in
android/app/build.gradle
:
apply plugin: 'com.google.gms.google-services'
For iOS:
• Register your iOS bundle ID.
• DownloadGoogleService-Info.plist
, add it toios/Runner
in Xcode.
• In your Podfile, set platform to iOS 10+ and runpod install
.Enable Firebase Cloud Messaging in the Firebase Console under Project Settings > Cloud Messaging.
Configuring Flutter App
Add dependencies in pubspec.yaml:
dependencies:
firebase_core: ^2.0.0
firebase_messaging
Then, initialize Firebase and request notification permissions in your main.dart:
import 'package:flutter/material.dart';
import 'package:firebase_core/firebase_core.dart';
import 'package:firebase_messaging/firebase_messaging.dart';
Future<void> _firebaseMessagingBackgroundHandler(RemoteMessage message) async {
await Firebase.initializeApp();
print('Background message: ${message.messageId}');
}
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
FirebaseMessaging.onBackgroundMessage(_firebaseMessagingBackgroundHandler);
runApp(MyApp());
}
class MyApp extends StatefulWidget { /* … */ }
class _MyAppState extends State<MyApp> {
@override
void initState() {
super.initState();
_configureFCM();
}
void _configureFCM() async {
FirebaseMessaging messaging = FirebaseMessaging.instance;
NotificationSettings settings = await messaging.requestPermission();
if (settings.authorizationStatus == AuthorizationStatus.authorized) {
String? token = await messaging.getToken();
print('FCM Token: $token');
}
}
@override
Widget build(BuildContext context) {
return MaterialApp(home: Scaffold(body: Center(child: Text('Home'))));
}
}
Handling Incoming Notifications
Use stream listeners to handle foreground, background, and terminated states.
FirebaseMessaging.onMessage.listen((RemoteMessage message) {
print('Foreground message: ${message.notification?.title}');
// Display a local notification or update UI
});
FirebaseMessaging.onMessageOpenedApp.listen((RemoteMessage message) {
// Navigate the user based on message data
Navigator.pushNamed(context, '/chat', arguments: message.data);
});
• Foreground: onMessage triggers while the app is open.
• Background: _firebaseMessagingBackgroundHandler processes messages when the app is backgrounded.
• Terminated: Use FirebaseMessaging.instance.getInitialMessage() inside initState to catch the message that opened the app.
Advanced Topics and Best Practices
• Topic Subscriptions: Organize users by interests.
await FirebaseMessaging.instance.subscribeToTopic('news');
await FirebaseMessaging.instance.unsubscribeFromTopic('news');
• Data-Only Messages: Send silent data payloads for background processing.
• Notification Channels (Android): Define channels in AndroidManifest.xml for importance levels.
• Security: Secure server keys, validate tokens, and use Firebase Authentication to restrict access.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing push notifications with firebase cloud messaging in Flutter unlocks powerful engagement tools for your app. You’ve set up your Firebase project, configured FCM in Flutter, and learned to handle messages in all app states. Dive deeper into advanced topics like topic management and data-only payloads to build robust notification strategies.
Introduction
Push notifications are crucial for user engagement in modern mobile apps. By integrating firebase cloud messaging (FCM) with your Flutter project, you can send targeted messages, updates, and alerts to users in real time. This tutorial walks through setting up push notifications in Flutter using Firebase Cloud Messaging, covering both basic configuration and handling background messages. You should have a Flutter project ready and access to a Firebase console.
Setting up Firebase Project
Create a Firebase project at https://console.firebase.google.com.
For Android:
• Register your app with the Android package name.
• Downloadgoogle-services.json
and place it inandroid/app/
.
• Add the Firebase Gradle plugin toandroid/build.gradle
:
dependencies { classpath 'com.google.gms:google-services:4.3.10'
• Apply the plugin in
android/app/build.gradle
:
apply plugin: 'com.google.gms.google-services'
For iOS:
• Register your iOS bundle ID.
• DownloadGoogleService-Info.plist
, add it toios/Runner
in Xcode.
• In your Podfile, set platform to iOS 10+ and runpod install
.Enable Firebase Cloud Messaging in the Firebase Console under Project Settings > Cloud Messaging.
Configuring Flutter App
Add dependencies in pubspec.yaml:
dependencies:
firebase_core: ^2.0.0
firebase_messaging
Then, initialize Firebase and request notification permissions in your main.dart:
import 'package:flutter/material.dart';
import 'package:firebase_core/firebase_core.dart';
import 'package:firebase_messaging/firebase_messaging.dart';
Future<void> _firebaseMessagingBackgroundHandler(RemoteMessage message) async {
await Firebase.initializeApp();
print('Background message: ${message.messageId}');
}
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
FirebaseMessaging.onBackgroundMessage(_firebaseMessagingBackgroundHandler);
runApp(MyApp());
}
class MyApp extends StatefulWidget { /* … */ }
class _MyAppState extends State<MyApp> {
@override
void initState() {
super.initState();
_configureFCM();
}
void _configureFCM() async {
FirebaseMessaging messaging = FirebaseMessaging.instance;
NotificationSettings settings = await messaging.requestPermission();
if (settings.authorizationStatus == AuthorizationStatus.authorized) {
String? token = await messaging.getToken();
print('FCM Token: $token');
}
}
@override
Widget build(BuildContext context) {
return MaterialApp(home: Scaffold(body: Center(child: Text('Home'))));
}
}
Handling Incoming Notifications
Use stream listeners to handle foreground, background, and terminated states.
FirebaseMessaging.onMessage.listen((RemoteMessage message) {
print('Foreground message: ${message.notification?.title}');
// Display a local notification or update UI
});
FirebaseMessaging.onMessageOpenedApp.listen((RemoteMessage message) {
// Navigate the user based on message data
Navigator.pushNamed(context, '/chat', arguments: message.data);
});
• Foreground: onMessage triggers while the app is open.
• Background: _firebaseMessagingBackgroundHandler processes messages when the app is backgrounded.
• Terminated: Use FirebaseMessaging.instance.getInitialMessage() inside initState to catch the message that opened the app.
Advanced Topics and Best Practices
• Topic Subscriptions: Organize users by interests.
await FirebaseMessaging.instance.subscribeToTopic('news');
await FirebaseMessaging.instance.unsubscribeFromTopic('news');
• Data-Only Messages: Send silent data payloads for background processing.
• Notification Channels (Android): Define channels in AndroidManifest.xml for importance levels.
• Security: Secure server keys, validate tokens, and use Firebase Authentication to restrict access.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing push notifications with firebase cloud messaging in Flutter unlocks powerful engagement tools for your app. You’ve set up your Firebase project, configured FCM in Flutter, and learned to handle messages in all app states. Dive deeper into advanced topics like topic management and data-only payloads to build robust notification strategies.
Supercharge Notifications with Vibe Studio
Supercharge Notifications with Vibe Studio
Supercharge Notifications with Vibe Studio
Supercharge Notifications with Vibe Studio
Leverage Steve’s AI agents in Vibe Studio to seamlessly integrate FCM into your Flutter app. Configure notifications and manage user engagement—all visually.
Leverage Steve’s AI agents in Vibe Studio to seamlessly integrate FCM into your Flutter app. Configure notifications and manage user engagement—all visually.
Leverage Steve’s AI agents in Vibe Studio to seamlessly integrate FCM into your Flutter app. Configure notifications and manage user engagement—all visually.
Leverage Steve’s AI agents in Vibe Studio to seamlessly integrate FCM into your Flutter app. Configure notifications and manage user engagement—all visually.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






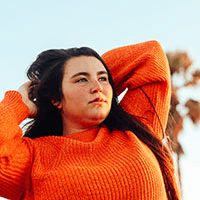



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025