Implementing MVVM Architecture in Flutter
May 9, 2025



Summary
Summary
Summary
Summary
The article guides developers through implementing MVVM in Flutter by organizing code into models, repositories, ViewModels, and views, enabling clear data flow, improved testability, and simplified UI binding using provider or similar tools.
The article guides developers through implementing MVVM in Flutter by organizing code into models, repositories, ViewModels, and views, enabling clear data flow, improved testability, and simplified UI binding using provider or similar tools.
The article guides developers through implementing MVVM in Flutter by organizing code into models, repositories, ViewModels, and views, enabling clear data flow, improved testability, and simplified UI binding using provider or similar tools.
The article guides developers through implementing MVVM in Flutter by organizing code into models, repositories, ViewModels, and views, enabling clear data flow, improved testability, and simplified UI binding using provider or similar tools.
Key insights:
Key insights:
Key insights:
Key insights:
Layered Folder Structure: Organize
models
,repositories
,services
,view_models
, andviews
for maintainability.Repository Abstraction: Decouple data sources for flexibility and easier testing.
Reactive ViewModel: Use
ChangeNotifier
or streams to notify UI of state changes.UI Binding: Inject ViewModels into widgets using
ChangeNotifierProvider
for reactive updates.Testability: Isolated ViewModel logic enables easy unit testing with mocked repositories.
Scalability Tips: Integrate DI (get_it), rxdart, or bloc to extend MVVM for larger apps.
Introduction
Implementing MVVM architecture in Flutter helps you separate UI, business logic, and data layers. The Model-View-ViewModel (MVVM) pattern improves testability, maintainability, and scalability of your Flutter apps. In this intermediate tutorial, you’ll learn how to structure a simple counter app using mvvm, create repositories for data access, implement a ViewModel for state management, and bind your Flutter widgets to reactive ViewModel properties.
Project Structure
A clean folder structure enforces the MVVM pattern. Create these directories under lib/:
Example:
lib/
├─ models/
├─ repositories/
├─ services/
├─ view_models/
└─ views
models/ holds plain Dart classes
repositories/ abstracts data sources, like network or local storage
services/ can wrap Firebase or HTTP clients
view_models/ contains your business logic and reactive state
views/ holds UI widgets that observe ViewModel changes.
Implementing the Model and Repository
First, define a simple counter model:
class Counter {
final int value;
Counter(this.value);
}
Next, create a repository that simulates fetching and updating the counter. This decouples your data layer from business logic, a key aspect of the mvvm pattern.
class CounterRepository {
int _counter = 0;
Future<Counter> fetchCounter() async {
// Simulate network/database delay
await Future.delayed(Duration(milliseconds: 300));
return Counter(_counter);
}
Future<Counter> incrementCounter() async {
await Future.delayed(Duration(milliseconds: 200));
_counter++;
return Counter(_counter);
}
}
By isolating data operations, you can easily swap the implementation with a real API or local database without touching your ViewModel or UI code.
Building the ViewModel
The ViewModel should extend ChangeNotifier or use a reactive library like provider to notify listeners. It calls the repository and exposes streams or properties for the view. Here’s a basic ViewModel:
class CounterViewModel extends ChangeNotifier {
final CounterRepository _repo;
int _count = 0;
bool _isLoading = false;
CounterViewModel(this._repo);
int get count => _count;
bool get isLoading => _isLoading;
Future<void> loadCounter() async {
_isLoading = true;
notifyListeners();
final counter = await _repo.fetchCounter();
_count = counter.value;
_isLoading = false;
notifyListeners();
}
Future<void> increment() async {
_isLoading = true;
notifyListeners();
final counter = await _repo.incrementCounter();
_count = counter.value;
_isLoading = false;
notifyListeners();
}
}
This ViewModel follows the MVVM pattern by handling all business logic and state transitions, while keeping the UI layer simple.
Connecting the View with ViewModel
In your views/ folder, create a CounterView widget. Use ChangeNotifierProvider from the provider package to inject the ViewModel.
class CounterView extends StatelessWidget {
@override
Widget build(BuildContext context) {
return ChangeNotifierProvider(
create: (_) => CounterViewModel(CounterRepository())..loadCounter(),
child: Scaffold(
appBar: AppBar(title: Text('MVVM Counter')),
body: Consumer<CounterViewModel>(
builder: (_, vm, __) {
if (vm.isLoading) return Center(child: CircularProgressIndicator());
return Center(
child: Text('Count: ${vm.count}', style: TextStyle(fontSize: 32)),
);
},
),
floatingActionButton: Consumer<CounterViewModel>
This view listens to changes in the ViewModel and rebuilds only the necessary widgets. It demonstrates a clear separation: the UI layer never directly calls the repository or manages business logic.
Best Practices and Variations
Dependency Injection: Use get_it or similar service locators to manage repository and service instances.
Reactive Extensions: Consider rxdart or flutter_bloc for event-driven mvvm.
Testing: Write unit tests for ViewModel methods. Mock repositories to verify behavior without hitting real APIs.
Error Handling: Expose error states in your ViewModel and update the UI with proper error messages.
These variations allow you to scale the MVVM implementation for larger Flutter apps and handle complicated business requirements.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
By following this guide, you’ve implemented an MVVM architecture in Flutter with a clean separation of concerns. You’ve learned how to define models, abstract data access with repositories, encapsulate business logic in ViewModels, and bind UI widgets to reactive properties. This approach enhances code readability, testability, and maintainability.
Introduction
Implementing MVVM architecture in Flutter helps you separate UI, business logic, and data layers. The Model-View-ViewModel (MVVM) pattern improves testability, maintainability, and scalability of your Flutter apps. In this intermediate tutorial, you’ll learn how to structure a simple counter app using mvvm, create repositories for data access, implement a ViewModel for state management, and bind your Flutter widgets to reactive ViewModel properties.
Project Structure
A clean folder structure enforces the MVVM pattern. Create these directories under lib/:
Example:
lib/
├─ models/
├─ repositories/
├─ services/
├─ view_models/
└─ views
models/ holds plain Dart classes
repositories/ abstracts data sources, like network or local storage
services/ can wrap Firebase or HTTP clients
view_models/ contains your business logic and reactive state
views/ holds UI widgets that observe ViewModel changes.
Implementing the Model and Repository
First, define a simple counter model:
class Counter {
final int value;
Counter(this.value);
}
Next, create a repository that simulates fetching and updating the counter. This decouples your data layer from business logic, a key aspect of the mvvm pattern.
class CounterRepository {
int _counter = 0;
Future<Counter> fetchCounter() async {
// Simulate network/database delay
await Future.delayed(Duration(milliseconds: 300));
return Counter(_counter);
}
Future<Counter> incrementCounter() async {
await Future.delayed(Duration(milliseconds: 200));
_counter++;
return Counter(_counter);
}
}
By isolating data operations, you can easily swap the implementation with a real API or local database without touching your ViewModel or UI code.
Building the ViewModel
The ViewModel should extend ChangeNotifier or use a reactive library like provider to notify listeners. It calls the repository and exposes streams or properties for the view. Here’s a basic ViewModel:
class CounterViewModel extends ChangeNotifier {
final CounterRepository _repo;
int _count = 0;
bool _isLoading = false;
CounterViewModel(this._repo);
int get count => _count;
bool get isLoading => _isLoading;
Future<void> loadCounter() async {
_isLoading = true;
notifyListeners();
final counter = await _repo.fetchCounter();
_count = counter.value;
_isLoading = false;
notifyListeners();
}
Future<void> increment() async {
_isLoading = true;
notifyListeners();
final counter = await _repo.incrementCounter();
_count = counter.value;
_isLoading = false;
notifyListeners();
}
}
This ViewModel follows the MVVM pattern by handling all business logic and state transitions, while keeping the UI layer simple.
Connecting the View with ViewModel
In your views/ folder, create a CounterView widget. Use ChangeNotifierProvider from the provider package to inject the ViewModel.
class CounterView extends StatelessWidget {
@override
Widget build(BuildContext context) {
return ChangeNotifierProvider(
create: (_) => CounterViewModel(CounterRepository())..loadCounter(),
child: Scaffold(
appBar: AppBar(title: Text('MVVM Counter')),
body: Consumer<CounterViewModel>(
builder: (_, vm, __) {
if (vm.isLoading) return Center(child: CircularProgressIndicator());
return Center(
child: Text('Count: ${vm.count}', style: TextStyle(fontSize: 32)),
);
},
),
floatingActionButton: Consumer<CounterViewModel>
This view listens to changes in the ViewModel and rebuilds only the necessary widgets. It demonstrates a clear separation: the UI layer never directly calls the repository or manages business logic.
Best Practices and Variations
Dependency Injection: Use get_it or similar service locators to manage repository and service instances.
Reactive Extensions: Consider rxdart or flutter_bloc for event-driven mvvm.
Testing: Write unit tests for ViewModel methods. Mock repositories to verify behavior without hitting real APIs.
Error Handling: Expose error states in your ViewModel and update the UI with proper error messages.
These variations allow you to scale the MVVM implementation for larger Flutter apps and handle complicated business requirements.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
By following this guide, you’ve implemented an MVVM architecture in Flutter with a clean separation of concerns. You’ve learned how to define models, abstract data access with repositories, encapsulate business logic in ViewModels, and bind UI widgets to reactive properties. This approach enhances code readability, testability, and maintainability.
Architect Cleanly with AI Assistance
Architect Cleanly with AI Assistance
Architect Cleanly with AI Assistance
Architect Cleanly with AI Assistance
Vibe Studio streamlines MVVM setup—automating ViewModel bindings, repository scaffolds, and reactive UI design.
Vibe Studio streamlines MVVM setup—automating ViewModel bindings, repository scaffolds, and reactive UI design.
Vibe Studio streamlines MVVM setup—automating ViewModel bindings, repository scaffolds, and reactive UI design.
Vibe Studio streamlines MVVM setup—automating ViewModel bindings, repository scaffolds, and reactive UI design.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






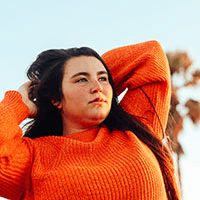



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025