Implementing Deep Links and App Links in Flutter
May 8, 2025



Summary
Summary
Summary
Summary
The tutorial details configuring Android and iOS deep/app links, verifying domains, and using the uni_links package to handle external URLs and route users to specific Flutter screens.
The tutorial details configuring Android and iOS deep/app links, verifying domains, and using the uni_links package to handle external URLs and route users to specific Flutter screens.
The tutorial details configuring Android and iOS deep/app links, verifying domains, and using the uni_links package to handle external URLs and route users to specific Flutter screens.
The tutorial details configuring Android and iOS deep/app links, verifying domains, and using the uni_links package to handle external URLs and route users to specific Flutter screens.
Key insights:
Key insights:
Key insights:
Key insights:
Platform Setup Required: Configure Android intent filters and iOS associated domains for deep linking.
Domain Verification: Host assetlinks.json and apple-app-site-association to validate ownership.
Flutter Handling: Use the uni_links package to capture and route incoming URIs in Dart.
Router Integration: Combine with go_router for advanced, pattern-based navigation.
Debugging Tips: Test with adb, Safari, and uriLinkStream; validate JSON and MIME types.
Support All App States: Ensure getInitialUri and uriLinkStream work in cold and warm starts.
Introduction
Deep links and app links allow Flutter apps to respond to external URLs, enabling seamless navigation from emails, websites, or other apps directly to specific content. Deep linking improves user experience, boosts engagement, and supports marketing campaigns by directing users to targeted screens. This tutorial covers intermediate‐level implementation of deep links (also called app links) on Android and iOS, plus handling link data in Flutter using the uni_links package.
Configuring Deep Links on Android
To support Android app links, you declare intent filters in AndroidManifest.xml. App links use the HTTP/HTTPS scheme and require a Digital Asset Links file hosted on your domain. While this is not Dart code, these steps lay the groundwork:
Add an intent filter under your main activity:
• Scheme: http/https
• Host: your domain
• Path prefix: the path you want to handleHost assetlinks.json in https:///.well-known/assetlinks.json to verify your app’s signing certificate.
Example snippet (AndroidManifest.xml):
<intent-filter android:autoVerify="true">
<action android:name="android.intent.action.VIEW"/>
<category android:name="android.intent.category.DEFAULT"/>
<category android:name="android.intent.category.BROWSABLE"/>
<data android:scheme="https"
android:host="example.com"
android:pathPrefix="/product"/>
</intent-filter>
Configuring Deep Links on iOS
On iOS, you configure Universal Links by:
Enabling Associated Domains in Xcode (Capabilities → Associated Domains → add applinks:example.com).
Hosting an apple-app-site-association file at https://example.com/apple-app-site-association (without extension).
This provisioning tells iOS that your app handles links for your domain.
Handling and Routing Deep Links in Flutter
Flutter supports deep linking through packages like uni_links or router solutions such as go_router. Here, we use uni_links for direct URI handling:
import 'package:flutter/material.dart';
import 'package:uni_links/uni_links.dart';
import 'dart:async';
class DeepLinkHandler extends StatefulWidget {
@override
_DeepLinkHandlerState createState() => _DeepLinkHandlerState();
}
class _DeepLinkHandlerState extends State<DeepLinkHandler> {
StreamSubscription<Uri?>? _sub;
@override
void initState() {
super.initState();
// Handle cold start
_initLink();
// Handle warm start
_sub = uriLinkStream.listen(_onUriReceived);
}
Future<void> _initLink() async {
final Uri? initialUri = await getInitialUri();
if (initialUri != null) _onUriReceived(initialUri);
}
void _onUriReceived(Uri? uri) {
if (uri == null) return;
// Example: myapp://product/42 → navigate to product detail
final segments = uri.pathSegments;
if (segments.isNotEmpty && segments.first == 'product') {
final id = segments.length > 1 ? segments[1] : null;
if (id != null) Navigator.pushNamed(context, '/product/$id');
}
}
@override
void dispose() {
_sub?.cancel();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(body: Center(child: Text('Waiting for deep link...')));
}
}
For complex routing, integrate URI parsing with a router package. For example, with go_router you can define routes that match URL patterns and push pages automatically.
Testing and Debugging Deep Links
• Android: Use adb shell am start -a android.intent.action.VIEW -d "https://example.com/product/42" com.yourcompany.app
• iOS Simulator: Open Safari → enter your Universal Link URL.
• Log and inspect uriLinkStream to ensure your app receives the correct URI.
• Check assetlinks.json or apple-app-site-association hosting and MIME type; errors in JSON or headers often break verification.
• Test cold starts (app closed) and warm starts (app backgrounded) to verify both getInitialUri and uriLinkStream deliver the URL.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing deep links and app links in Flutter involves configuration on both Android and iOS platforms, plus handling incoming URIs in your Dart code. By using the uni_links package, you can react to external URLs and navigate users directly to relevant screens, improving engagement and providing a fluid user experience.
Introduction
Deep links and app links allow Flutter apps to respond to external URLs, enabling seamless navigation from emails, websites, or other apps directly to specific content. Deep linking improves user experience, boosts engagement, and supports marketing campaigns by directing users to targeted screens. This tutorial covers intermediate‐level implementation of deep links (also called app links) on Android and iOS, plus handling link data in Flutter using the uni_links package.
Configuring Deep Links on Android
To support Android app links, you declare intent filters in AndroidManifest.xml. App links use the HTTP/HTTPS scheme and require a Digital Asset Links file hosted on your domain. While this is not Dart code, these steps lay the groundwork:
Add an intent filter under your main activity:
• Scheme: http/https
• Host: your domain
• Path prefix: the path you want to handleHost assetlinks.json in https:///.well-known/assetlinks.json to verify your app’s signing certificate.
Example snippet (AndroidManifest.xml):
<intent-filter android:autoVerify="true">
<action android:name="android.intent.action.VIEW"/>
<category android:name="android.intent.category.DEFAULT"/>
<category android:name="android.intent.category.BROWSABLE"/>
<data android:scheme="https"
android:host="example.com"
android:pathPrefix="/product"/>
</intent-filter>
Configuring Deep Links on iOS
On iOS, you configure Universal Links by:
Enabling Associated Domains in Xcode (Capabilities → Associated Domains → add applinks:example.com).
Hosting an apple-app-site-association file at https://example.com/apple-app-site-association (without extension).
This provisioning tells iOS that your app handles links for your domain.
Handling and Routing Deep Links in Flutter
Flutter supports deep linking through packages like uni_links or router solutions such as go_router. Here, we use uni_links for direct URI handling:
import 'package:flutter/material.dart';
import 'package:uni_links/uni_links.dart';
import 'dart:async';
class DeepLinkHandler extends StatefulWidget {
@override
_DeepLinkHandlerState createState() => _DeepLinkHandlerState();
}
class _DeepLinkHandlerState extends State<DeepLinkHandler> {
StreamSubscription<Uri?>? _sub;
@override
void initState() {
super.initState();
// Handle cold start
_initLink();
// Handle warm start
_sub = uriLinkStream.listen(_onUriReceived);
}
Future<void> _initLink() async {
final Uri? initialUri = await getInitialUri();
if (initialUri != null) _onUriReceived(initialUri);
}
void _onUriReceived(Uri? uri) {
if (uri == null) return;
// Example: myapp://product/42 → navigate to product detail
final segments = uri.pathSegments;
if (segments.isNotEmpty && segments.first == 'product') {
final id = segments.length > 1 ? segments[1] : null;
if (id != null) Navigator.pushNamed(context, '/product/$id');
}
}
@override
void dispose() {
_sub?.cancel();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(body: Center(child: Text('Waiting for deep link...')));
}
}
For complex routing, integrate URI parsing with a router package. For example, with go_router you can define routes that match URL patterns and push pages automatically.
Testing and Debugging Deep Links
• Android: Use adb shell am start -a android.intent.action.VIEW -d "https://example.com/product/42" com.yourcompany.app
• iOS Simulator: Open Safari → enter your Universal Link URL.
• Log and inspect uriLinkStream to ensure your app receives the correct URI.
• Check assetlinks.json or apple-app-site-association hosting and MIME type; errors in JSON or headers often break verification.
• Test cold starts (app closed) and warm starts (app backgrounded) to verify both getInitialUri and uriLinkStream deliver the URL.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing deep links and app links in Flutter involves configuration on both Android and iOS platforms, plus handling incoming URIs in your Dart code. By using the uni_links package, you can react to external URLs and navigate users directly to relevant screens, improving engagement and providing a fluid user experience.
Link Smarter with Vibe Studio
Link Smarter with Vibe Studio
Link Smarter with Vibe Studio
Link Smarter with Vibe Studio
Vibe Studio lets you build Flutter apps with deep link support and Firebase backend—fast, no code, AI-driven.
Vibe Studio lets you build Flutter apps with deep link support and Firebase backend—fast, no code, AI-driven.
Vibe Studio lets you build Flutter apps with deep link support and Firebase backend—fast, no code, AI-driven.
Vibe Studio lets you build Flutter apps with deep link support and Firebase backend—fast, no code, AI-driven.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






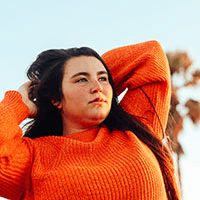



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025