Implementing Background Execution with Flutter WorkManager
May 8, 2025



Summary
Summary
Summary
Summary
The guide shows how to set up and implement background execution in Flutter using the WorkManager plugin, including task definition, scheduling strategies, and debugging tips for robust performance on Android and iOS.
The guide shows how to set up and implement background execution in Flutter using the WorkManager plugin, including task definition, scheduling strategies, and debugging tips for robust performance on Android and iOS.
The guide shows how to set up and implement background execution in Flutter using the WorkManager plugin, including task definition, scheduling strategies, and debugging tips for robust performance on Android and iOS.
The guide shows how to set up and implement background execution in Flutter using the WorkManager plugin, including task definition, scheduling strategies, and debugging tips for robust performance on Android and iOS.
Key insights:
Key insights:
Key insights:
Key insights:
Cross-Platform Setup: Configure Android and iOS platforms to support background modes and permissions.
Task Definition Rules: Background functions must be static or top-level, with runtime payload fetching for efficiency.
Scheduling Flexibility: Use one-off or periodic tasks with constraints like network and battery status.
Debugging Tools: Leverage adb, Xcode logs, and mock interfaces for reliable testing.
Cold Start Validation: Ensure tasks execute after app termination or reboot for real-world reliability.
Retry and Monitoring: Handle failures with retry flags and integrate with logging services.
Introduction
In modern mobile applications, background execution is essential for tasks like syncing data, cleaning up storage, sending notifications, or fetching content periodically—all without requiring the app to be open or active. Flutter, while primarily optimized for foreground interactions, offers a powerful plugin—WorkManager—to bridge this gap. By leveraging platform-native capabilities on both Android and iOS, WorkManager allows Flutter apps to perform scheduled or event-driven background tasks in a battery-efficient and reliable manner. This guide walks you through setting up, implementing, and debugging background tasks using WorkManager, ensuring your Flutter app can stay functional and responsive—even when it's not in the foreground.
Setup and Initialization
Add dependency to pubspec.yaml:
dependencies:
workmanager: ^0.5.0
Android:
In
android/app/src/main/AndroidManifest.xml
, add permissions and service registration:<uses-permission android:name="android.permission.WAKE_LOCK"/> <application> <service android:name="be.tramckrijte.workmanager.WorkManagerTaskService" android:exported="false" /> </application>
iOS:
Enable Background Fetch in Xcode (Signing & Capabilities > Background Modes).
In your Dart entrypoint (usually
main.dart
), initialize the Workmanager early:
import 'package:workmanager/workmanager.dart';
void callbackDispatcher() {
Workmanager().executeTask((taskName, inputData) async {
// route tasks by name
if (taskName == "syncTask") {
// perform API sync
}
return Future.value(true);
});
}
void main() {
WidgetsFlutterBinding.ensureInitialized();
Workmanager().initialize(
callbackDispatcher,
isInDebugMode: false, // set true for detailed logs
);
runApp(MyApp());
}
Defining Background Tasks
Every background task must be a top-level or static function. Within executeTask, you can inspect taskName and optional inputData.
Network Calls and Persistence: Use http and sqflite or shared_preferences within the dispatcher.
Handling Large Payloads: Keep data minimal in inputData; fetch additional payload at runtime.
Error Handling: Return false to signal retry or log errors to remote monitoring (e.g., Sentry).
Example dispatcher snippet for file cleanup:
void callbackDispatcher() {
Workmanager().executeTask((task, data) async {
try {
final dir = Directory(data?['path'] ?? '/tmp');
final files = dir.listSync();
for (var f in files) {
if (DateTime.now().difference(f.statSync().modified) > Duration(days: 7)) {
await f.delete();
}
}
return true;
} catch (e) {
print('Cleanup error: $e');
return false;
}
});
}
Scheduling Strategies
You can enqueue one-off or periodic tasks with constraints:
One-off Tasks: Good for ad-hoc operations.
Periodic Tasks: Ideal for regular syncs, with minimum 15-minute interval on Android.
Constraints: NetworkType (none, unmetered, connected), requiresCharging, requiresBatteryNotLow, requiresDeviceIdle.
Example scheduling a periodic sync:
Workmanager().registerPeriodicTask(
"periodicSync", // unique name
"syncTask", // callback name
frequency: Duration(hours: 6), // interval
constraints: Constraints(
networkType: NetworkType.connected, // only when online
requiresCharging: true, // device must be charging
),
inputData: {'endpoint': '/api/v1/update'},
);
To cancel tasks:
Workmanager().cancelByUniqueName("periodicSync");
Testing and Debugging
Debug Mode:
isInDebugMode: true
prints detailed logs.Android Studio: Use “Flutter ► Attach Debugger to Android Process” after scheduling a task.
Cold Start: Terminate your app completely to verify tasks run after reboot.
Logs: On Android use
adb logcat | grep WM_Task;
on iOS check Xcode console.Unit Testing: Mock
Workmanager
by abstracting scheduling calls behind an interface.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing background execution with Flutter WorkManager (workmanager plugin) empowers your app with robust, platform-native scheduling capabilities. By properly initializing the dispatcher, defining tasks as top-level functions, applying constraints, and leveraging one-off or periodic schedules, you’ll build reliable background processes for data sync, notifications, or maintenance. Rigorous testing and debug configurations ensure these tasks run under real-world conditions, delivering a seamless user experience even when your app isn’t in the foreground.
Introduction
In modern mobile applications, background execution is essential for tasks like syncing data, cleaning up storage, sending notifications, or fetching content periodically—all without requiring the app to be open or active. Flutter, while primarily optimized for foreground interactions, offers a powerful plugin—WorkManager—to bridge this gap. By leveraging platform-native capabilities on both Android and iOS, WorkManager allows Flutter apps to perform scheduled or event-driven background tasks in a battery-efficient and reliable manner. This guide walks you through setting up, implementing, and debugging background tasks using WorkManager, ensuring your Flutter app can stay functional and responsive—even when it's not in the foreground.
Setup and Initialization
Add dependency to pubspec.yaml:
dependencies:
workmanager: ^0.5.0
Android:
In
android/app/src/main/AndroidManifest.xml
, add permissions and service registration:<uses-permission android:name="android.permission.WAKE_LOCK"/> <application> <service android:name="be.tramckrijte.workmanager.WorkManagerTaskService" android:exported="false" /> </application>
iOS:
Enable Background Fetch in Xcode (Signing & Capabilities > Background Modes).
In your Dart entrypoint (usually
main.dart
), initialize the Workmanager early:
import 'package:workmanager/workmanager.dart';
void callbackDispatcher() {
Workmanager().executeTask((taskName, inputData) async {
// route tasks by name
if (taskName == "syncTask") {
// perform API sync
}
return Future.value(true);
});
}
void main() {
WidgetsFlutterBinding.ensureInitialized();
Workmanager().initialize(
callbackDispatcher,
isInDebugMode: false, // set true for detailed logs
);
runApp(MyApp());
}
Defining Background Tasks
Every background task must be a top-level or static function. Within executeTask, you can inspect taskName and optional inputData.
Network Calls and Persistence: Use http and sqflite or shared_preferences within the dispatcher.
Handling Large Payloads: Keep data minimal in inputData; fetch additional payload at runtime.
Error Handling: Return false to signal retry or log errors to remote monitoring (e.g., Sentry).
Example dispatcher snippet for file cleanup:
void callbackDispatcher() {
Workmanager().executeTask((task, data) async {
try {
final dir = Directory(data?['path'] ?? '/tmp');
final files = dir.listSync();
for (var f in files) {
if (DateTime.now().difference(f.statSync().modified) > Duration(days: 7)) {
await f.delete();
}
}
return true;
} catch (e) {
print('Cleanup error: $e');
return false;
}
});
}
Scheduling Strategies
You can enqueue one-off or periodic tasks with constraints:
One-off Tasks: Good for ad-hoc operations.
Periodic Tasks: Ideal for regular syncs, with minimum 15-minute interval on Android.
Constraints: NetworkType (none, unmetered, connected), requiresCharging, requiresBatteryNotLow, requiresDeviceIdle.
Example scheduling a periodic sync:
Workmanager().registerPeriodicTask(
"periodicSync", // unique name
"syncTask", // callback name
frequency: Duration(hours: 6), // interval
constraints: Constraints(
networkType: NetworkType.connected, // only when online
requiresCharging: true, // device must be charging
),
inputData: {'endpoint': '/api/v1/update'},
);
To cancel tasks:
Workmanager().cancelByUniqueName("periodicSync");
Testing and Debugging
Debug Mode:
isInDebugMode: true
prints detailed logs.Android Studio: Use “Flutter ► Attach Debugger to Android Process” after scheduling a task.
Cold Start: Terminate your app completely to verify tasks run after reboot.
Logs: On Android use
adb logcat | grep WM_Task;
on iOS check Xcode console.Unit Testing: Mock
Workmanager
by abstracting scheduling calls behind an interface.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing background execution with Flutter WorkManager (workmanager plugin) empowers your app with robust, platform-native scheduling capabilities. By properly initializing the dispatcher, defining tasks as top-level functions, applying constraints, and leveraging one-off or periodic schedules, you’ll build reliable background processes for data sync, notifications, or maintenance. Rigorous testing and debug configurations ensure these tasks run under real-world conditions, delivering a seamless user experience even when your app isn’t in the foreground.
Run Background Tasks with Ease
Run Background Tasks with Ease
Run Background Tasks with Ease
Run Background Tasks with Ease
Vibe Studio helps you build full-stack Flutter apps with WorkManager and Firebase—no code, just results.
Vibe Studio helps you build full-stack Flutter apps with WorkManager and Firebase—no code, just results.
Vibe Studio helps you build full-stack Flutter apps with WorkManager and Firebase—no code, just results.
Vibe Studio helps you build full-stack Flutter apps with WorkManager and Firebase—no code, just results.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






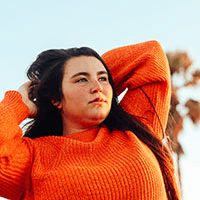



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025