Implementing AdaptiveLayout in Flutter for Seamless Multi-Platform Design
May 6, 2025



Summary
Summary
Summary
Summary
The article details how Flutter's AdaptiveLayout system enables responsive UIs across phones, tablets, foldables, and desktops by defining layout slots and breakpoints. It outlines best practices for integrating adaptive patterns incrementally and optimizing performance, while also showcasing how Vibe Studio simplifies multi-platform Flutter development.
The article details how Flutter's AdaptiveLayout system enables responsive UIs across phones, tablets, foldables, and desktops by defining layout slots and breakpoints. It outlines best practices for integrating adaptive patterns incrementally and optimizing performance, while also showcasing how Vibe Studio simplifies multi-platform Flutter development.
The article details how Flutter's AdaptiveLayout system enables responsive UIs across phones, tablets, foldables, and desktops by defining layout slots and breakpoints. It outlines best practices for integrating adaptive patterns incrementally and optimizing performance, while also showcasing how Vibe Studio simplifies multi-platform Flutter development.
The article details how Flutter's AdaptiveLayout system enables responsive UIs across phones, tablets, foldables, and desktops by defining layout slots and breakpoints. It outlines best practices for integrating adaptive patterns incrementally and optimizing performance, while also showcasing how Vibe Studio simplifies multi-platform Flutter development.
Key insights:
Key insights:
Key insights:
Key insights:
AdaptiveLayout Simplifies UI Logic: Centralizes responsive behavior by mapping UI slots to device breakpoints.
Custom BreakpointSets: Tailor layout behavior to your design system using predefined or custom breakpoints.
DisplayFeature Support: Handle foldables and notches by dynamically adjusting layout padding and positioning.
Incremental Refactoring: Retrofit AdaptiveLayout into legacy codebases by wrapping top-level pages and reducing MediaQuery logic.
Performance Best Practices: Lazy-load widgets in slot builders and use DevTools to monitor layout behavior.
Test for Device Variants: Write widget tests and use emulator previews to ensure consistent behavior across platforms.
Introduction
Creating a seamless user experience across phones, tablets, foldables, and desktops is a core challenge for modern Flutter apps. With the flutter adaptive layout paradigm, you can declaratively define multiple UI variants for different screen sizes and orientations. This tutorial explores how to leverage the AdaptiveLayout widget and related tools to build fluid, multi-platform designs without duplicating code.
Understanding AdaptiveLayout
AdaptiveLayout in Flutter is a high-level widget that orchestrates different UI “slots” based on defined breakpoints. It evaluates device characteristics at runtime—screen width, height, and display features (like hinges or cutouts)—and picks the appropriate layout builder for your UI. Key concepts:
• BreakpointSet: A collection of size thresholds (e.g., small <600px, medium 600–1024px, large >1024px).
• LayoutSlot: A named region (small, medium, large) with its own widget builder.
• DisplayFeature: Extra constraints from foldables or cutouts, enabling you to adjust padding or reposition content.
By using flutter adaptive layout patterns, you reduce conditional logic scattered across build methods and centralize your responsive rules.
Managing Breakpoints and LayoutSlots
Define breakpoints that align with your design system. Flutter’s SDK provides default sets, or you can craft a custom one:
final myBreakpoints = Breakpoints.fromMap({
LayoutSize.small: 0,
LayoutSize.medium: 600,
LayoutSize.large: 1024,
});
To wire them into an AdaptiveLayout:
AdaptiveLayout(
breakpoints: myBreakpoints,
small: (context) => SmallDashboard(),
medium: (context) => MediumDashboard(),
large: (context) => LargeDashboard(),
);
Here:
– The framework measures MediaQuery size and selects the matching slot.
– You can omit a slot; AdaptiveLayout falls back to the next-largest or next-smallest variant.
Pro tip: Combine DisplayFeatures to detect foldable hinges:
AdaptiveLayout(
displayFeatures: MediaQuery.of(context).displayFeatures,
// Slot builders...
);
Building Responsive Widgets
Within each slot, you can still apply nuanced responsive tweaks:
class MediumDashboard extends StatelessWidget {
@override
Widget build(BuildContext context) {
final orientation = MediaQuery.of(context).orientation;
return Row(
children: [
Expanded(child: OverviewCard()),
if (orientation == Orientation.landscape)
Expanded(child: StatsPanel()),
],
);
}
}
Best practices:
• Use Expanded, Flexible, and LayoutBuilder for fluid sizing.
• Favor Wrap or GridView for lists that reflow across breakpoints.
• Extract shared components and parameterize only layout-critical properties.
Additionally, consider the AdaptiveNavigationRail widget for medium and large layouts, and fall back to a BottomNavigationBar on small screens.
Integration with Existing Codebases
Transitioning an established Flutter project to a flutter adaptive layout architecture can be incremental:
• Identify top-level pages (e.g., HomePage, SettingsPage). Wrap their Scaffold in AdaptiveLayout.
• Replace manual MediaQuery checks with slot builders.
• Consolidate duplicate sizing constants into your BreakpointSet.
• Test on multiple emulators (phone, tablet, foldable). Flutter’s device previews can simulate common sizes.
Sample integration:
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return AdaptiveLayout(
breakpoints: kDefaultBreakpoints,
small: (_) => MobileHome(),
medium: (_) => TabletHome(),
large: (_) => DesktopHome(),
);
}
}
This pattern keeps your code modular and emphasizes design logic over spread-out conditionals.
Performance and Testing Tips
• Lazy-load heavy widgets within slot builders to avoid overhead on unused layouts.
• Use Flutter DevTools to inspect MediaQuery values and DisplayFeatures in real time.
• Write widget tests that pump different DeviceSize constraints, verifying that AdaptiveLayout selects the correct builder.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing flutter adaptive layouts unlocks a truly multi-platform strategy, letting you maintain a single codebase while delivering optimized experiences for phones, tablets, foldables, and desktops. By defining clear breakpoints, centralizing your layout logic, and incrementally refactoring existing pages, you’ll achieve a responsive, maintainable UI architecture.
Introduction
Creating a seamless user experience across phones, tablets, foldables, and desktops is a core challenge for modern Flutter apps. With the flutter adaptive layout paradigm, you can declaratively define multiple UI variants for different screen sizes and orientations. This tutorial explores how to leverage the AdaptiveLayout widget and related tools to build fluid, multi-platform designs without duplicating code.
Understanding AdaptiveLayout
AdaptiveLayout in Flutter is a high-level widget that orchestrates different UI “slots” based on defined breakpoints. It evaluates device characteristics at runtime—screen width, height, and display features (like hinges or cutouts)—and picks the appropriate layout builder for your UI. Key concepts:
• BreakpointSet: A collection of size thresholds (e.g., small <600px, medium 600–1024px, large >1024px).
• LayoutSlot: A named region (small, medium, large) with its own widget builder.
• DisplayFeature: Extra constraints from foldables or cutouts, enabling you to adjust padding or reposition content.
By using flutter adaptive layout patterns, you reduce conditional logic scattered across build methods and centralize your responsive rules.
Managing Breakpoints and LayoutSlots
Define breakpoints that align with your design system. Flutter’s SDK provides default sets, or you can craft a custom one:
final myBreakpoints = Breakpoints.fromMap({
LayoutSize.small: 0,
LayoutSize.medium: 600,
LayoutSize.large: 1024,
});
To wire them into an AdaptiveLayout:
AdaptiveLayout(
breakpoints: myBreakpoints,
small: (context) => SmallDashboard(),
medium: (context) => MediumDashboard(),
large: (context) => LargeDashboard(),
);
Here:
– The framework measures MediaQuery size and selects the matching slot.
– You can omit a slot; AdaptiveLayout falls back to the next-largest or next-smallest variant.
Pro tip: Combine DisplayFeatures to detect foldable hinges:
AdaptiveLayout(
displayFeatures: MediaQuery.of(context).displayFeatures,
// Slot builders...
);
Building Responsive Widgets
Within each slot, you can still apply nuanced responsive tweaks:
class MediumDashboard extends StatelessWidget {
@override
Widget build(BuildContext context) {
final orientation = MediaQuery.of(context).orientation;
return Row(
children: [
Expanded(child: OverviewCard()),
if (orientation == Orientation.landscape)
Expanded(child: StatsPanel()),
],
);
}
}
Best practices:
• Use Expanded, Flexible, and LayoutBuilder for fluid sizing.
• Favor Wrap or GridView for lists that reflow across breakpoints.
• Extract shared components and parameterize only layout-critical properties.
Additionally, consider the AdaptiveNavigationRail widget for medium and large layouts, and fall back to a BottomNavigationBar on small screens.
Integration with Existing Codebases
Transitioning an established Flutter project to a flutter adaptive layout architecture can be incremental:
• Identify top-level pages (e.g., HomePage, SettingsPage). Wrap their Scaffold in AdaptiveLayout.
• Replace manual MediaQuery checks with slot builders.
• Consolidate duplicate sizing constants into your BreakpointSet.
• Test on multiple emulators (phone, tablet, foldable). Flutter’s device previews can simulate common sizes.
Sample integration:
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return AdaptiveLayout(
breakpoints: kDefaultBreakpoints,
small: (_) => MobileHome(),
medium: (_) => TabletHome(),
large: (_) => DesktopHome(),
);
}
}
This pattern keeps your code modular and emphasizes design logic over spread-out conditionals.
Performance and Testing Tips
• Lazy-load heavy widgets within slot builders to avoid overhead on unused layouts.
• Use Flutter DevTools to inspect MediaQuery values and DisplayFeatures in real time.
• Write widget tests that pump different DeviceSize constraints, verifying that AdaptiveLayout selects the correct builder.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Implementing flutter adaptive layouts unlocks a truly multi-platform strategy, letting you maintain a single codebase while delivering optimized experiences for phones, tablets, foldables, and desktops. By defining clear breakpoints, centralizing your layout logic, and incrementally refactoring existing pages, you’ll achieve a responsive, maintainable UI architecture.
Design once. Deploy everywhere.
Design once. Deploy everywhere.
Design once. Deploy everywhere.
Design once. Deploy everywhere.
Vibe Studio empowers you to build adaptive Flutter UIs visually, with Steve’s AI agents guiding multi-platform layout creation.
Vibe Studio empowers you to build adaptive Flutter UIs visually, with Steve’s AI agents guiding multi-platform layout creation.
Vibe Studio empowers you to build adaptive Flutter UIs visually, with Steve’s AI agents guiding multi-platform layout creation.
Vibe Studio empowers you to build adaptive Flutter UIs visually, with Steve’s AI agents guiding multi-platform layout creation.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






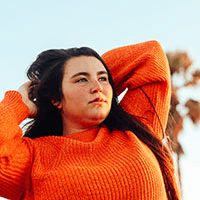



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025