High‑Performance Flutter Animations with frameScheduling
May 7, 2025



Summary
Summary
Summary
Summary
Standard Flutter animations work for most apps, but fine-tuned performance requires using SchedulerBinding for frame-level control. This guide shows how to schedule animations with addFrameCallback, optimize repaint cycles, and reduce jank. With Vibe Studio, developers can build high-performance UI prototypes quickly and visually.
Standard Flutter animations work for most apps, but fine-tuned performance requires using SchedulerBinding for frame-level control. This guide shows how to schedule animations with addFrameCallback, optimize repaint cycles, and reduce jank. With Vibe Studio, developers can build high-performance UI prototypes quickly and visually.
Standard Flutter animations work for most apps, but fine-tuned performance requires using SchedulerBinding for frame-level control. This guide shows how to schedule animations with addFrameCallback, optimize repaint cycles, and reduce jank. With Vibe Studio, developers can build high-performance UI prototypes quickly and visually.
Standard Flutter animations work for most apps, but fine-tuned performance requires using SchedulerBinding for frame-level control. This guide shows how to schedule animations with addFrameCallback, optimize repaint cycles, and reduce jank. With Vibe Studio, developers can build high-performance UI prototypes quickly and visually.
Key insights:
Key insights:
Key insights:
Key insights:
Frame-Level Control: Use
addPersistentFrameCallback
to drive animations with vsync precision.Minimize Jank: Schedule lightweight updates each frame to avoid layout/paint delays.
Rendering Efficiency: Use
RepaintBoundary
and defer heavy work to isolates or FFI.Profiling is Critical: Target <16ms per frame using Flutter DevTools for UI and GPU analysis.
State Management Discipline: Only mutate essential state per frame to keep rendering lean.
Custom Use Cases: Ideal for complex visuals like particles, physics, or real-time dashboards.
Introduction
High-performance animations are critical in delivering fluid, responsive Flutter experiences. Standard animation APIs like AnimationController and Tween cover most use cases, but millisecond-level control often requires diving into Flutter's frame scheduling internals. This tutorial explores frame scheduling for maximum animation efficiency, reducing jank and improving rendering throughput in performance-critical scenarios.
Frame Scheduling Mechanics
At its core, Flutter orchestrates rendering via the SchedulerBinding, which emits each frame at vsync. By registering frame callbacks (SchedulerBinding.instance.addFrameCallback or addPersistentFrameCallback), you bypass some overhead of higher-level abstractions and exert fine-grained timing control.
SchedulerBinding: the heart of Flutter’s event loop.
FrameCallback: invoked once per frame, just before build/layout.
addPersistentFrameCallback: useful when you need a callback every frame without re-registering.
Under the hood, you control exactly when your logic executes in relation to the screen refresh. For extremely complex animations—particle systems, physics simulations, or large canvas updates—this approach yields smoother, more deterministic behavior.
Implementing a Frame-Scheduled Animation
Below is an example of a custom pulsating circle harnessing addPersistentFrameCallback. It illustrates how you can manage your own tick logic on each vsync.
import 'dart:ui';
import 'package:flutter/scheduler.dart';
import 'package:flutter/widgets.dart';
class PulsatingCircle extends StatefulWidget {
@override
_PulsatingCircleState createState() => _PulsatingCircleState();
}
class _PulsatingCircleState extends State<PulsatingCircle> {
late double radius;
late Duration lastTime;
@override
void initState() {
super.initState();
radius = 20.0;
lastTime = Duration.zero;
SchedulerBinding.instance.addPersistentFrameCallback(_tick);
SchedulerBinding.instance.scheduleFrame();
}
void _tick(Duration timestamp) {
final dt = (lastTime == Duration.zero ? 0 : timestamp.inMilliseconds - lastTime.inMilliseconds).toDouble();
lastTime = timestamp;
// Increase and decrease radius at 60px/sec
radius += (dt / 1000) * 60 * (radius < 50 ? 1 : -1);
if (radius < 20) radius = 20;
setState(() {});
SchedulerBinding.instance.scheduleFrame();
}
@override
Widget build(BuildContext context) {
return CustomPaint(
painter: _CirclePainter(radius),
child: SizedBox.expand(),
);
}
}
class _CirclePainter extends CustomPainter {
final double radius;
_CirclePainter(this.radius);
@override
void paint(Canvas canvas, Size size) {
final center = size.center(Offset.zero);
canvas.drawCircle(center, radius, Paint()..color = const Color(0xFF2196F3));
}
@override
bool shouldRepaint(_CirclePainter old) => old.radius != radius;
}
This code delivers smooth animations by driving state changes in lockstep with frame callbacks, minimizing the window for dropped frames and harnessing Flutter’s GPU pipeline effectively for performance animations.
Profiling and Optimizing
Even with frame scheduling, profiling remains essential. Use Flutter DevTools to capture the timeline and inspect both UI and GPU threads. Look for:
• Frame times consistently below 16ms.
• Long layout or paint spikes.
• Rasterizer thread contention.
Tip: defer expensive work outside frame callbacks. For instance, precompute paths or prepare layers ahead of time, and only trigger a minimal repaint every tick. When you have multiple layers, use RepaintBoundary to isolate expensive paint work. This approach ensures smooth animations and further enhances performance.
If you find CPU-bound code throttling your frame rate, offload complex calculations to isolates or leverage the C++ side via FFI for heavy numeric loops. Keep your frame callback lean—mutate minimal state, trigger a simple setState, and let Flutter’s rendering pipeline optimize the rest.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
By combining Vibe Studio’s rapid app scaffolding with custom performance animations like the ones detailed here, you can build feature-rich prototypes that maintain production-grade smoothness without sacrificing developer velocity.
Conclusion
Mastering frame scheduling elevates your performance animations beyond what standard APIs provide. By tapping directly into SchedulerBinding, you control animation timing, reduce overhead, and maintain sub-16ms frame times. Pair these techniques with disciplined profiling in Flutter DevTools, strategic use of RepaintBoundary, and offloading CPU-heavy tasks for maximal throughput. Whether you’re crafting interactive infographics, particle systems, or real-time data visualizations, frame scheduling is your key to silky-smooth, high-performance animations in Flutter.
Introduction
High-performance animations are critical in delivering fluid, responsive Flutter experiences. Standard animation APIs like AnimationController and Tween cover most use cases, but millisecond-level control often requires diving into Flutter's frame scheduling internals. This tutorial explores frame scheduling for maximum animation efficiency, reducing jank and improving rendering throughput in performance-critical scenarios.
Frame Scheduling Mechanics
At its core, Flutter orchestrates rendering via the SchedulerBinding, which emits each frame at vsync. By registering frame callbacks (SchedulerBinding.instance.addFrameCallback or addPersistentFrameCallback), you bypass some overhead of higher-level abstractions and exert fine-grained timing control.
SchedulerBinding: the heart of Flutter’s event loop.
FrameCallback: invoked once per frame, just before build/layout.
addPersistentFrameCallback: useful when you need a callback every frame without re-registering.
Under the hood, you control exactly when your logic executes in relation to the screen refresh. For extremely complex animations—particle systems, physics simulations, or large canvas updates—this approach yields smoother, more deterministic behavior.
Implementing a Frame-Scheduled Animation
Below is an example of a custom pulsating circle harnessing addPersistentFrameCallback. It illustrates how you can manage your own tick logic on each vsync.
import 'dart:ui';
import 'package:flutter/scheduler.dart';
import 'package:flutter/widgets.dart';
class PulsatingCircle extends StatefulWidget {
@override
_PulsatingCircleState createState() => _PulsatingCircleState();
}
class _PulsatingCircleState extends State<PulsatingCircle> {
late double radius;
late Duration lastTime;
@override
void initState() {
super.initState();
radius = 20.0;
lastTime = Duration.zero;
SchedulerBinding.instance.addPersistentFrameCallback(_tick);
SchedulerBinding.instance.scheduleFrame();
}
void _tick(Duration timestamp) {
final dt = (lastTime == Duration.zero ? 0 : timestamp.inMilliseconds - lastTime.inMilliseconds).toDouble();
lastTime = timestamp;
// Increase and decrease radius at 60px/sec
radius += (dt / 1000) * 60 * (radius < 50 ? 1 : -1);
if (radius < 20) radius = 20;
setState(() {});
SchedulerBinding.instance.scheduleFrame();
}
@override
Widget build(BuildContext context) {
return CustomPaint(
painter: _CirclePainter(radius),
child: SizedBox.expand(),
);
}
}
class _CirclePainter extends CustomPainter {
final double radius;
_CirclePainter(this.radius);
@override
void paint(Canvas canvas, Size size) {
final center = size.center(Offset.zero);
canvas.drawCircle(center, radius, Paint()..color = const Color(0xFF2196F3));
}
@override
bool shouldRepaint(_CirclePainter old) => old.radius != radius;
}
This code delivers smooth animations by driving state changes in lockstep with frame callbacks, minimizing the window for dropped frames and harnessing Flutter’s GPU pipeline effectively for performance animations.
Profiling and Optimizing
Even with frame scheduling, profiling remains essential. Use Flutter DevTools to capture the timeline and inspect both UI and GPU threads. Look for:
• Frame times consistently below 16ms.
• Long layout or paint spikes.
• Rasterizer thread contention.
Tip: defer expensive work outside frame callbacks. For instance, precompute paths or prepare layers ahead of time, and only trigger a minimal repaint every tick. When you have multiple layers, use RepaintBoundary to isolate expensive paint work. This approach ensures smooth animations and further enhances performance.
If you find CPU-bound code throttling your frame rate, offload complex calculations to isolates or leverage the C++ side via FFI for heavy numeric loops. Keep your frame callback lean—mutate minimal state, trigger a simple setState, and let Flutter’s rendering pipeline optimize the rest.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
By combining Vibe Studio’s rapid app scaffolding with custom performance animations like the ones detailed here, you can build feature-rich prototypes that maintain production-grade smoothness without sacrificing developer velocity.
Conclusion
Mastering frame scheduling elevates your performance animations beyond what standard APIs provide. By tapping directly into SchedulerBinding, you control animation timing, reduce overhead, and maintain sub-16ms frame times. Pair these techniques with disciplined profiling in Flutter DevTools, strategic use of RepaintBoundary, and offloading CPU-heavy tasks for maximal throughput. Whether you’re crafting interactive infographics, particle systems, or real-time data visualizations, frame scheduling is your key to silky-smooth, high-performance animations in Flutter.
Prototype High-Performance Flutter UIs
Prototype High-Performance Flutter UIs
Prototype High-Performance Flutter UIs
Prototype High-Performance Flutter UIs
Use Vibe Studio to visually build Flutter apps with frame-synced, production-grade animations.
Use Vibe Studio to visually build Flutter apps with frame-synced, production-grade animations.
Use Vibe Studio to visually build Flutter apps with frame-synced, production-grade animations.
Use Vibe Studio to visually build Flutter apps with frame-synced, production-grade animations.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






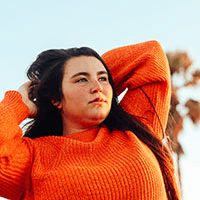



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025