Custom Widget Development in Flutter: A Step-by-Step Guide
May 6, 2025



Summary
Summary
Summary
Summary
The guide walks through designing a custom GradientButton widget in Flutter, covering encapsulation, composition, theming, testing, and animation—while highlighting how platforms like Vibe Studio can accelerate development by visually managing custom UI components with no code.
The guide walks through designing a custom GradientButton widget in Flutter, covering encapsulation, composition, theming, testing, and animation—while highlighting how platforms like Vibe Studio can accelerate development by visually managing custom UI components with no code.
The guide walks through designing a custom GradientButton widget in Flutter, covering encapsulation, composition, theming, testing, and animation—while highlighting how platforms like Vibe Studio can accelerate development by visually managing custom UI components with no code.
The guide walks through designing a custom GradientButton widget in Flutter, covering encapsulation, composition, theming, testing, and animation—while highlighting how platforms like Vibe Studio can accelerate development by visually managing custom UI components with no code.
Key insights:
Key insights:
Key insights:
Key insights:
Encapsulation Benefits: Custom widgets bundle behavior, layout, and styling for reuse and consistency.
Composition First: Use
Ink
,InkWell
, and theming to create flexible, ripple-enabled buttons.Clean APIs: Expose minimal, meaningful parameters with sensible defaults for ease of use.
Theme Integration: Leverage
ThemeExtension
and context-based theming for dark mode and platform support.Advanced Customization: Add animation and state to enhance interactivity using
StatefulWidget
.Visual Development: Vibe Studio enables visual editing and deployment of custom widgets with instant code output.
Introduction
In Flutter, creating a custom widget allows you to encapsulate design patterns, improve code reuse, and tailor UI components to your exact specifications. This guide dives deep into custom widget development, walking you through design, implementation, and advanced techniques. We’ll build a reusable GradientButton, explore composition, apply animations, and show how to integrate your widget into larger apps.
Why Build a Custom Widget
Before writing a single line of code, understand the benefits of a custom widget:
• Encapsulation: Bundle layout, styling, and behavior in one class.
• Reusability: Share a single source of truth across screens.
• Consistency: Enforce design tokens (colors, typography).
• Maintenance: Update features in one place without hunting through the codebase.
Custom widgets are the foundation of scalable Flutter apps. Whether you’re customizing widgets for theme overrides or creating a complex form control, a well-designed custom widget can streamline both development and testing.
Designing Your Custom Widget
Identify parameters: color, size, callbacks.
Define a clear API: keep constructors simple and required.
Optimize for composition: allow child widgets and theming.
Let’s draft a GradientButton interface:
class GradientButton extends StatelessWidget {
final Widget child;
final Gradient gradient;
final VoidCallback onPressed;
final EdgeInsets padding;
const GradientButton({
Key? key,
required this.child,
required this.gradient,
required this.onPressed,
this.padding = const EdgeInsets.symmetric(vertical: 12, horizontal: 16),
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Material(
color: Colors.transparent,
child: Ink(
decoration: BoxDecoration(
gradient: gradient,
borderRadius: BorderRadius.circular(8),
),
child: InkWell(
onTap: onPressed,
borderRadius: BorderRadius.circular(8),
child: Padding(
padding: padding,
child: Center(child: child),
),
),
),
);
}
}
Key takeaways:
• Use composition over inheritance: wrap Ink and InkWell for ripple effects.
• Expose only essential properties, keep defaults sensible.
• Leverage const constructors for performance.
Implementing and Integrating the Widget
With your GradientButton defined, integrate it into a screen:
class DemoScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Center(
child: GradientButton(
gradient: LinearGradient(colors: [Colors.blue, Colors.purple]),
onPressed: () => print('GradientButton tapped'),
child: Text('Press Me', style: TextStyle(color: Colors.white)),
),
);
}
}
Tips for smooth integration:
• Theming: wrap your widget in Theme or pass Theme.of(context) values.
• Responsiveness: use MediaQuery or layout builders for adaptive sizes.
• Testing: write widget tests to assert layout, tap callbacks, and visual states.
Advanced Customization Techniques
1. State Management & Animation
For dynamic UI, convert your custom widget into a StatefulWidget and inject animations:
class AnimatedGradientButton extends StatefulWidget {
// same constructor args
@override
_AnimatedGradientButtonState createState() => _AnimatedGradientButtonState();
}
class _AnimatedGradientButtonState extends State<AnimatedGradientButton>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
late Animation<Alignment> _alignmentAnim;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: Duration(seconds: 3),
vsync: this,
)..repeat(reverse: true);
_alignmentAnim = Tween<Alignment>(
begin: Alignment.topLeft,
end: Alignment.bottomRight,
).animate(_controller);
}
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: _alignmentAnim,
builder: (context, child) => GradientButton(
gradient: LinearGradient(
begin: _alignmentAnim.value,
end: Alignment.center,
colors: [Colors.teal, Colors.greenAccent],
),
onPressed: widget.onPressed,
child: widget.child,
),
);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
}
2. Theming and Inheritance
To make your custom widgets theme-aware:
• Define ThemeExtension for custom properties.
• Read values via Theme.of(context).extension(). • Support dark mode, high-contrast, and platform overrides.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Mastering custom widget creation in Flutter elevates your code from prototype to production. Custom widgets and customizing widgets are the building blocks of expressive, maintainable Flutter apps. As you evolve, explore more advanced patterns like render objects and slivers for ultimate control. Leverage platforms like Vibe Studio to accelerate app delivery, and keep refining your toolkit for robust, scalable Flutter development.
Introduction
In Flutter, creating a custom widget allows you to encapsulate design patterns, improve code reuse, and tailor UI components to your exact specifications. This guide dives deep into custom widget development, walking you through design, implementation, and advanced techniques. We’ll build a reusable GradientButton, explore composition, apply animations, and show how to integrate your widget into larger apps.
Why Build a Custom Widget
Before writing a single line of code, understand the benefits of a custom widget:
• Encapsulation: Bundle layout, styling, and behavior in one class.
• Reusability: Share a single source of truth across screens.
• Consistency: Enforce design tokens (colors, typography).
• Maintenance: Update features in one place without hunting through the codebase.
Custom widgets are the foundation of scalable Flutter apps. Whether you’re customizing widgets for theme overrides or creating a complex form control, a well-designed custom widget can streamline both development and testing.
Designing Your Custom Widget
Identify parameters: color, size, callbacks.
Define a clear API: keep constructors simple and required.
Optimize for composition: allow child widgets and theming.
Let’s draft a GradientButton interface:
class GradientButton extends StatelessWidget {
final Widget child;
final Gradient gradient;
final VoidCallback onPressed;
final EdgeInsets padding;
const GradientButton({
Key? key,
required this.child,
required this.gradient,
required this.onPressed,
this.padding = const EdgeInsets.symmetric(vertical: 12, horizontal: 16),
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Material(
color: Colors.transparent,
child: Ink(
decoration: BoxDecoration(
gradient: gradient,
borderRadius: BorderRadius.circular(8),
),
child: InkWell(
onTap: onPressed,
borderRadius: BorderRadius.circular(8),
child: Padding(
padding: padding,
child: Center(child: child),
),
),
),
);
}
}
Key takeaways:
• Use composition over inheritance: wrap Ink and InkWell for ripple effects.
• Expose only essential properties, keep defaults sensible.
• Leverage const constructors for performance.
Implementing and Integrating the Widget
With your GradientButton defined, integrate it into a screen:
class DemoScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Center(
child: GradientButton(
gradient: LinearGradient(colors: [Colors.blue, Colors.purple]),
onPressed: () => print('GradientButton tapped'),
child: Text('Press Me', style: TextStyle(color: Colors.white)),
),
);
}
}
Tips for smooth integration:
• Theming: wrap your widget in Theme or pass Theme.of(context) values.
• Responsiveness: use MediaQuery or layout builders for adaptive sizes.
• Testing: write widget tests to assert layout, tap callbacks, and visual states.
Advanced Customization Techniques
1. State Management & Animation
For dynamic UI, convert your custom widget into a StatefulWidget and inject animations:
class AnimatedGradientButton extends StatefulWidget {
// same constructor args
@override
_AnimatedGradientButtonState createState() => _AnimatedGradientButtonState();
}
class _AnimatedGradientButtonState extends State<AnimatedGradientButton>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
late Animation<Alignment> _alignmentAnim;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: Duration(seconds: 3),
vsync: this,
)..repeat(reverse: true);
_alignmentAnim = Tween<Alignment>(
begin: Alignment.topLeft,
end: Alignment.bottomRight,
).animate(_controller);
}
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: _alignmentAnim,
builder: (context, child) => GradientButton(
gradient: LinearGradient(
begin: _alignmentAnim.value,
end: Alignment.center,
colors: [Colors.teal, Colors.greenAccent],
),
onPressed: widget.onPressed,
child: widget.child,
),
);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
}
2. Theming and Inheritance
To make your custom widgets theme-aware:
• Define ThemeExtension for custom properties.
• Read values via Theme.of(context).extension(). • Support dark mode, high-contrast, and platform overrides.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Mastering custom widget creation in Flutter elevates your code from prototype to production. Custom widgets and customizing widgets are the building blocks of expressive, maintainable Flutter apps. As you evolve, explore more advanced patterns like render objects and slivers for ultimate control. Leverage platforms like Vibe Studio to accelerate app delivery, and keep refining your toolkit for robust, scalable Flutter development.
Create smarter widgets with Vibe Studio
Create smarter widgets with Vibe Studio
Create smarter widgets with Vibe Studio
Create smarter widgets with Vibe Studio
Use Vibe Studio’s conversational tools to design, style, and deploy custom widgets without boilerplate—powered by Steve’s AI.
Use Vibe Studio’s conversational tools to design, style, and deploy custom widgets without boilerplate—powered by Steve’s AI.
Use Vibe Studio’s conversational tools to design, style, and deploy custom widgets without boilerplate—powered by Steve’s AI.
Use Vibe Studio’s conversational tools to design, style, and deploy custom widgets without boilerplate—powered by Steve’s AI.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






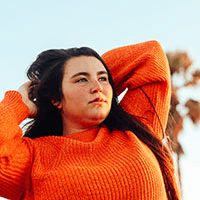



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025