Building Desktop Apps with Flutter on macOS and Windows
May 8, 2025



Summary
Summary
Summary
Summary
The tutorial outlines how to set up Flutter for desktop development, customize macOS and Windows builds, and optimize UI and performance for large screens, high DPI, and native input handling.
The tutorial outlines how to set up Flutter for desktop development, customize macOS and Windows builds, and optimize UI and performance for large screens, high DPI, and native input handling.
The tutorial outlines how to set up Flutter for desktop development, customize macOS and Windows builds, and optimize UI and performance for large screens, high DPI, and native input handling.
The tutorial outlines how to set up Flutter for desktop development, customize macOS and Windows builds, and optimize UI and performance for large screens, high DPI, and native input handling.
Key insights:
Key insights:
Key insights:
Key insights:
Environment Setup: Install platform-specific SDKs and enable desktop support in Flutter.
Cross-Platform Project Structure: Use a shared codebase with native build folders for macOS and Windows.
Native Customization: Add macOS menus or modify Windows window styles for native UX.
UI Scaling: Use LayoutBuilder, MediaQuery, and DPI-aware settings for responsive desktop layouts.
Performance Tuning: Enable shaders, lazy-load assets, and profile with DevTools to meet desktop demands.
Input Support: Enhance user experience with keyboard shortcuts and mouse interaction widgets.
Introduction
Flutter’s shift to stable desktop support unlocks true cross-platform desktop development. Whether targeting macOS desktop or Windows desktop environments, Flutter’s single codebase streamlines UI consistency, benefiting startups, solo founders, and agile engineering teams. This tutorial dives into setting up, configuring, and optimizing a Flutter desktop app on both macOS and Windows, with code snippets and best practices.
Setting Up Your Environment
Before coding, confirm your machine meets the requirements:
• macOS desktop: macOS 10.15+ with Xcode installed. • Windows desktop: Windows 10 SDK (10.0.18362.0+), Visual Studio 2019+ with the “Desktop development with C++” workload.
Enable Flutter’s desktop support in your channel:
flutter channel stable
flutter upgrade
flutter config --enable-macos-desktop
flutter config --enable-windows-desktop
Verify targets:
flutter devices
# Should list macOS and Windows (if on the respective host)
These steps make flutter create scaffold desktop folders alongside lib/, android/, and ios/.
Creating a Desktop Flutter Project
Generate a new app:
flutter create my_desktop_app
cd my_desktop_app
Review the project tree—desktop/macos and desktop/windows contain native build files. Open macOS in Xcode (open macos/Runner.xcworkspace) or Windows in Visual Studio. Test your app:
flutter run -d macos # on macOS host
flutter run -d windows # on Windows host
The default main.dart uses MaterialApp. For desktop, you might switch to a responsive layout or add menu bars. Example minimal main.dart:
import 'package:flutter/material.dart';
void main() {
runApp(const DesktopApp());
}
class DesktopApp extends StatelessWidget {
const DesktopApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Desktop Example',
home: Scaffold(
appBar: AppBar(title: const Text('Desktop Home')),
body: const Center(child: Text('Hello, Desktop Flutter!')),
),
);
}
}
Run and verify window resizing, DPI scaling, and keyboard/mouse interactions.
Platform-Specific Considerations
While Flutter’s core UI code is platform-agnostic, desktop exposes native extensions.
macOS desktop:
• Add menus via NSMenu in AppDelegate.swift.
• Handle dark mode and retina scaling in Info.plist.
Example addition in macos/Runner/AppDelegate.swift:
override func applicationDidFinishLaunching(_ notification: Notification) {
let mainMenu = NSMenu()
mainMenu.addItem(NSMenuItem(title: "File", action: nil, keyEquivalent: ""))
NSApp.mainMenu = mainMenu
super.applicationDidFinishLaunching(notification)
}
Windows desktop:
• Modify windows/runner/win32_window.cpp for window style (resizable, minimum size).
• Customize DPI awareness in win32_window.cpp by invoking SetProcessDpiAwarenessContext(DPI_AWARENESS_CONTEXT_PER_MONITOR_AWARE);.
These tweaks ensure native look-and-feel. Use conditional imports in Dart for platform-specific services:
import 'dart:io';
void setupPlatformFeatures() {
if (Platform.isMacOS) {
// initialize macOS-specific plugin
} else if (Platform.isWindows) {
// initialize Windows-specific plugin
}
}
Optimizing Performance and UI Adaptations
Desktop apps often demand higher frame rates, large window sizes, and support for keyboard/mouse inputs.
• Enable desktop shaders: flutter build windows --release --bundle-sksl-path=path/to/sksl.json.
• Use LayoutBuilder and MediaQuery.of(context).size to adapt layouts for large screens.
• Implement keyboard shortcuts with Shortcuts and Actions widgets:
Shortcuts(
shortcuts: {LogicalKeySet(LogicalKeyboardKey.control, LogicalKeyboardKey.s): SaveIntent()},
child: Actions(
actions: {SaveIntent: CallbackAction(onInvoke: (_) => saveDocument())},
child: Focus(
autofocus: true,
child: MyEditorWidget(),
),
),
);
• Lazy-load large assets and leverage compute for CPU-bound tasks. • Profile with flutter devtools to inspect memory usage and frame rendering times. Desktop targets often push beyond mobile’s memory and CPU envelope—profiling is key.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Flutter desktop development on macOS and Windows empowers teams to ship high-fidelity desktop apps using a unified Dart codebase. By configuring native build settings, customizing platform-specific behaviors, and optimizing for large screens and input devices, you can deliver production-ready desktop applications quickly and efficiently.
Introduction
Flutter’s shift to stable desktop support unlocks true cross-platform desktop development. Whether targeting macOS desktop or Windows desktop environments, Flutter’s single codebase streamlines UI consistency, benefiting startups, solo founders, and agile engineering teams. This tutorial dives into setting up, configuring, and optimizing a Flutter desktop app on both macOS and Windows, with code snippets and best practices.
Setting Up Your Environment
Before coding, confirm your machine meets the requirements:
• macOS desktop: macOS 10.15+ with Xcode installed. • Windows desktop: Windows 10 SDK (10.0.18362.0+), Visual Studio 2019+ with the “Desktop development with C++” workload.
Enable Flutter’s desktop support in your channel:
flutter channel stable
flutter upgrade
flutter config --enable-macos-desktop
flutter config --enable-windows-desktop
Verify targets:
flutter devices
# Should list macOS and Windows (if on the respective host)
These steps make flutter create scaffold desktop folders alongside lib/, android/, and ios/.
Creating a Desktop Flutter Project
Generate a new app:
flutter create my_desktop_app
cd my_desktop_app
Review the project tree—desktop/macos and desktop/windows contain native build files. Open macOS in Xcode (open macos/Runner.xcworkspace) or Windows in Visual Studio. Test your app:
flutter run -d macos # on macOS host
flutter run -d windows # on Windows host
The default main.dart uses MaterialApp. For desktop, you might switch to a responsive layout or add menu bars. Example minimal main.dart:
import 'package:flutter/material.dart';
void main() {
runApp(const DesktopApp());
}
class DesktopApp extends StatelessWidget {
const DesktopApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Desktop Example',
home: Scaffold(
appBar: AppBar(title: const Text('Desktop Home')),
body: const Center(child: Text('Hello, Desktop Flutter!')),
),
);
}
}
Run and verify window resizing, DPI scaling, and keyboard/mouse interactions.
Platform-Specific Considerations
While Flutter’s core UI code is platform-agnostic, desktop exposes native extensions.
macOS desktop:
• Add menus via NSMenu in AppDelegate.swift.
• Handle dark mode and retina scaling in Info.plist.
Example addition in macos/Runner/AppDelegate.swift:
override func applicationDidFinishLaunching(_ notification: Notification) {
let mainMenu = NSMenu()
mainMenu.addItem(NSMenuItem(title: "File", action: nil, keyEquivalent: ""))
NSApp.mainMenu = mainMenu
super.applicationDidFinishLaunching(notification)
}
Windows desktop:
• Modify windows/runner/win32_window.cpp for window style (resizable, minimum size).
• Customize DPI awareness in win32_window.cpp by invoking SetProcessDpiAwarenessContext(DPI_AWARENESS_CONTEXT_PER_MONITOR_AWARE);.
These tweaks ensure native look-and-feel. Use conditional imports in Dart for platform-specific services:
import 'dart:io';
void setupPlatformFeatures() {
if (Platform.isMacOS) {
// initialize macOS-specific plugin
} else if (Platform.isWindows) {
// initialize Windows-specific plugin
}
}
Optimizing Performance and UI Adaptations
Desktop apps often demand higher frame rates, large window sizes, and support for keyboard/mouse inputs.
• Enable desktop shaders: flutter build windows --release --bundle-sksl-path=path/to/sksl.json.
• Use LayoutBuilder and MediaQuery.of(context).size to adapt layouts for large screens.
• Implement keyboard shortcuts with Shortcuts and Actions widgets:
Shortcuts(
shortcuts: {LogicalKeySet(LogicalKeyboardKey.control, LogicalKeyboardKey.s): SaveIntent()},
child: Actions(
actions: {SaveIntent: CallbackAction(onInvoke: (_) => saveDocument())},
child: Focus(
autofocus: true,
child: MyEditorWidget(),
),
),
);
• Lazy-load large assets and leverage compute for CPU-bound tasks. • Profile with flutter devtools to inspect memory usage and frame rendering times. Desktop targets often push beyond mobile’s memory and CPU envelope—profiling is key.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Flutter desktop development on macOS and Windows empowers teams to ship high-fidelity desktop apps using a unified Dart codebase. By configuring native build settings, customizing platform-specific behaviors, and optimizing for large screens and input devices, you can deliver production-ready desktop applications quickly and efficiently.
Launch Desktop Apps with Vibe Studio
Launch Desktop Apps with Vibe Studio
Launch Desktop Apps with Vibe Studio
Launch Desktop Apps with Vibe Studio
Vibe Studio helps you build and deploy cross-platform Flutter desktop apps—fast, visual, and no-code.
Vibe Studio helps you build and deploy cross-platform Flutter desktop apps—fast, visual, and no-code.
Vibe Studio helps you build and deploy cross-platform Flutter desktop apps—fast, visual, and no-code.
Vibe Studio helps you build and deploy cross-platform Flutter desktop apps—fast, visual, and no-code.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






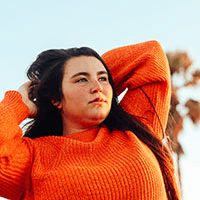



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025