Building Custom Flutter Render Objects for Fine‑Grained Control
May 7, 2025



Summary
Summary
Summary
Summary
Custom render objects in Flutter let advanced developers create bespoke layouts and graphics by overriding low-level methods like performLayout and paint. This guide walks through RenderBox anatomy, triangle rendering, and advanced techniques like intrinsic sizing and layer composition for optimized rendering.
Custom render objects in Flutter let advanced developers create bespoke layouts and graphics by overriding low-level methods like performLayout and paint. This guide walks through RenderBox anatomy, triangle rendering, and advanced techniques like intrinsic sizing and layer composition for optimized rendering.
Custom render objects in Flutter let advanced developers create bespoke layouts and graphics by overriding low-level methods like performLayout and paint. This guide walks through RenderBox anatomy, triangle rendering, and advanced techniques like intrinsic sizing and layer composition for optimized rendering.
Custom render objects in Flutter let advanced developers create bespoke layouts and graphics by overriding low-level methods like performLayout and paint. This guide walks through RenderBox anatomy, triangle rendering, and advanced techniques like intrinsic sizing and layer composition for optimized rendering.
Key insights:
Key insights:
Key insights:
Key insights:
RenderBox Basics: Override
performLayout
,paint
, andhitTest
for full control over layout and interaction.Custom Drawing: Use
Path
andCanvas
inpaint()
to render custom shapes like triangles or graphics.Widget Integration: Wrap your render object in a
RenderObjectWidget
to place it in any layout.Advanced Layout: Support intrinsic sizing by overriding compute methods for responsive layout behavior.
Performance Layers: Use
pushLayer
to optimize paint operations and improve visual performance.Repaint Logic: Trigger repaints with
markNeedsPaint
ormarkNeedsLayout
during dynamic updates.
Introduction
Flutter’s rendering layer grants unparalleled flexibility through render objects. While widgets and render boxes abstract most layouts, mastering custom render objects offers fine-grained control over layout, painting, and hit-testing. In this advanced guide, you’ll learn how to architect your own render box—from measuring and layout to painting—using Flutter’s low-level APIs.
Anatomy of Flutter Render Objects
A render object is the fundamental unit in Flutter’s rendering pipeline. Every widget that affects size or paint delegates to a render object beneath it. The most common base class is RenderBox, which provides a box‐model layout contract:
• performLayout(): measure and set size
• paint(): draw content onto the canvas
• hitTest(): define interactive regions
Key properties you’ll often override or use:
• constraints: BoxConstraints passed down from parent
• size: resolved dimensions after performLayout
• parentData: positional metadata in multi-child scenarios
Understanding these hooks is crucial for custom render objects and creating bespoke layouts or graphical primitives.
Building a Custom RenderObject
Suppose you need a triangle-shaped widget with dynamic color. Begin by subclassing RenderBox and mixing in DebugOverflowIndicatorMixin if you want overflow warnings. Implement performLayout to respect constraints, then paint.
class RenderTriangle extends RenderBox {
Color color;
RenderTriangle({required this.color});
@override
void performLayout() {
// Make it as large as the constraints allow (square)
final sizeValue = constraints.constrainWidth();
size = Size(sizeValue, sizeValue);
}
@override
void paint(PaintingContext context, Offset offset) {
final paint = Paint()..color = color;
final path = Path()
..moveTo(offset.dx + size.width / 2, offset.dy)
..lineTo(offset.dx, offset.dy + size.height)
..lineTo(offset.dx + size.width, offset.dy + size.height)
..close();
context.canvas.drawPath(path, paint);
}
@override
bool hitTestSelf(Offset position) {
// Treat entire bounding box as interactive
return Path()
.moveTo(size.width / 2, 0)
.lineTo(0, size.height)
.lineTo(size.width, size.height)
.close()
.contains(position);
}
}
In performLayout, we call constraints.constrainWidth() to pick the maximum permitted width, then make a square. In paint, we build a triangular Path and draw it. The hitTestSelf override ensures taps inside the triangle boundaries register.
Integrating Your Custom RenderObject into the Widget Tree
To expose your RenderTriangle as a reusable widget, wrap it in a RenderObjectWidget and corresponding Element:
class Triangle extends LeafRenderObjectWidget {
final Color color;
const Triangle({Key? key, this.color = Colors.blue}) : super(key: key);
@override
RenderTriangle createRenderObject(BuildContext context) {
return RenderTriangle(color: color);
}
@override
void updateRenderObject(BuildContext context, RenderTriangle renderObject) {
renderObject.color = color;
renderObject.markNeedsPaint();
}
}
With this widget, you can place Triangle() in any layout:
Center(child: SizedBox(width: 100, height: 100, child: Triangle(color: Colors.red)));
Advanced Techniques and Best Practices
Custom Constraints Logic: For compound render objects (RenderBoxContainerDefaultsMixin), override computeMinIntrinsicWidth/Height and computeMaxIntrinsicWidth/Height to support intrinsic sizing.
Painting Layers: Use context.pushLayer for background or shadow layers, improving performance by compositing.
Text Painters: For text within a render object, manage a TextPainter instance and call layout() on it.
Schedule Repaints: If your render object depends on external animations or timers, call markNeedsLayout or markNeedsPaint judiciously.
Debugging: leverage debugPaintSizeEnabled, and override debugFillProperties to expose your custom properties in Flutter’s DevTools.
Example: scheduling an animation repaint:
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_ticker = owner.createTicker(_tick)..start();
}
void _tick(Duration elapsed) {
// Update internal state, e.g., oscillating angle
markNeedsPaint();
}
@override
void detach() {
_ticker.dispose();
super.detach();
}
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Custom render objects unlock Flutter’s lowest-level APIs, enabling pixel-perfect layouts, custom hit-testing, and bespoke painting pipelines. By subclassing RenderBox (or other base classes like RenderSliver), overriding performLayout and paint, and wrapping your logic in a RenderObjectWidget, you achieve unrivaled control. Use advanced techniques—intrinsic sizing, layers, and scheduled repaint—to optimize both performance and developer experience. Dive into the rendering library, experiment with shapes, text, and even complex layout protocols to master Flutter render objects.
By understanding and leveraging custom render objects, you’ll craft UIs that look and behave exactly as envisioned, without fighting against higher-level abstractions.
Introduction
Flutter’s rendering layer grants unparalleled flexibility through render objects. While widgets and render boxes abstract most layouts, mastering custom render objects offers fine-grained control over layout, painting, and hit-testing. In this advanced guide, you’ll learn how to architect your own render box—from measuring and layout to painting—using Flutter’s low-level APIs.
Anatomy of Flutter Render Objects
A render object is the fundamental unit in Flutter’s rendering pipeline. Every widget that affects size or paint delegates to a render object beneath it. The most common base class is RenderBox, which provides a box‐model layout contract:
• performLayout(): measure and set size
• paint(): draw content onto the canvas
• hitTest(): define interactive regions
Key properties you’ll often override or use:
• constraints: BoxConstraints passed down from parent
• size: resolved dimensions after performLayout
• parentData: positional metadata in multi-child scenarios
Understanding these hooks is crucial for custom render objects and creating bespoke layouts or graphical primitives.
Building a Custom RenderObject
Suppose you need a triangle-shaped widget with dynamic color. Begin by subclassing RenderBox and mixing in DebugOverflowIndicatorMixin if you want overflow warnings. Implement performLayout to respect constraints, then paint.
class RenderTriangle extends RenderBox {
Color color;
RenderTriangle({required this.color});
@override
void performLayout() {
// Make it as large as the constraints allow (square)
final sizeValue = constraints.constrainWidth();
size = Size(sizeValue, sizeValue);
}
@override
void paint(PaintingContext context, Offset offset) {
final paint = Paint()..color = color;
final path = Path()
..moveTo(offset.dx + size.width / 2, offset.dy)
..lineTo(offset.dx, offset.dy + size.height)
..lineTo(offset.dx + size.width, offset.dy + size.height)
..close();
context.canvas.drawPath(path, paint);
}
@override
bool hitTestSelf(Offset position) {
// Treat entire bounding box as interactive
return Path()
.moveTo(size.width / 2, 0)
.lineTo(0, size.height)
.lineTo(size.width, size.height)
.close()
.contains(position);
}
}
In performLayout, we call constraints.constrainWidth() to pick the maximum permitted width, then make a square. In paint, we build a triangular Path and draw it. The hitTestSelf override ensures taps inside the triangle boundaries register.
Integrating Your Custom RenderObject into the Widget Tree
To expose your RenderTriangle as a reusable widget, wrap it in a RenderObjectWidget and corresponding Element:
class Triangle extends LeafRenderObjectWidget {
final Color color;
const Triangle({Key? key, this.color = Colors.blue}) : super(key: key);
@override
RenderTriangle createRenderObject(BuildContext context) {
return RenderTriangle(color: color);
}
@override
void updateRenderObject(BuildContext context, RenderTriangle renderObject) {
renderObject.color = color;
renderObject.markNeedsPaint();
}
}
With this widget, you can place Triangle() in any layout:
Center(child: SizedBox(width: 100, height: 100, child: Triangle(color: Colors.red)));
Advanced Techniques and Best Practices
Custom Constraints Logic: For compound render objects (RenderBoxContainerDefaultsMixin), override computeMinIntrinsicWidth/Height and computeMaxIntrinsicWidth/Height to support intrinsic sizing.
Painting Layers: Use context.pushLayer for background or shadow layers, improving performance by compositing.
Text Painters: For text within a render object, manage a TextPainter instance and call layout() on it.
Schedule Repaints: If your render object depends on external animations or timers, call markNeedsLayout or markNeedsPaint judiciously.
Debugging: leverage debugPaintSizeEnabled, and override debugFillProperties to expose your custom properties in Flutter’s DevTools.
Example: scheduling an animation repaint:
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_ticker = owner.createTicker(_tick)..start();
}
void _tick(Duration elapsed) {
// Update internal state, e.g., oscillating angle
markNeedsPaint();
}
@override
void detach() {
_ticker.dispose();
super.detach();
}
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Custom render objects unlock Flutter’s lowest-level APIs, enabling pixel-perfect layouts, custom hit-testing, and bespoke painting pipelines. By subclassing RenderBox (or other base classes like RenderSliver), overriding performLayout and paint, and wrapping your logic in a RenderObjectWidget, you achieve unrivaled control. Use advanced techniques—intrinsic sizing, layers, and scheduled repaint—to optimize both performance and developer experience. Dive into the rendering library, experiment with shapes, text, and even complex layout protocols to master Flutter render objects.
By understanding and leveraging custom render objects, you’ll craft UIs that look and behave exactly as envisioned, without fighting against higher-level abstractions.
Master rendering with Vibe Studio’s visual power
Master rendering with Vibe Studio’s visual power
Master rendering with Vibe Studio’s visual power
Master rendering with Vibe Studio’s visual power
Vibe Studio lets you build advanced Flutter UIs—combining custom render logic with AI-powered no-code tools and Firebase integration.
Vibe Studio lets you build advanced Flutter UIs—combining custom render logic with AI-powered no-code tools and Firebase integration.
Vibe Studio lets you build advanced Flutter UIs—combining custom render logic with AI-powered no-code tools and Firebase integration.
Vibe Studio lets you build advanced Flutter UIs—combining custom render logic with AI-powered no-code tools and Firebase integration.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






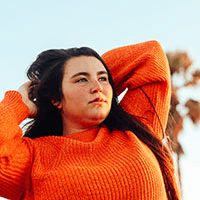



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025