Advanced State Management Techniques in Flutter
May 6, 2025



Summary
Summary
Summary
Summary
The tutorial explains advanced Flutter state management with bloc, including reactive streams, multi-bloc architecture, state persistence using hydrated_bloc, and performance tips for minimizing rebuilds and improving responsiveness in large apps.
The tutorial explains advanced Flutter state management with bloc, including reactive streams, multi-bloc architecture, state persistence using hydrated_bloc, and performance tips for minimizing rebuilds and improving responsiveness in large apps.
The tutorial explains advanced Flutter state management with bloc, including reactive streams, multi-bloc architecture, state persistence using hydrated_bloc, and performance tips for minimizing rebuilds and improving responsiveness in large apps.
The tutorial explains advanced Flutter state management with bloc, including reactive streams, multi-bloc architecture, state persistence using hydrated_bloc, and performance tips for minimizing rebuilds and improving responsiveness in large apps.
Key insights:
Key insights:
Key insights:
Key insights:
Reactive Streams: Bloc uses streams to map events to states, enabling fine-grained control and performance tuning.
Feature Isolation: Use MultiBlocProvider and feature folders to structure large apps with multiple interacting blocs.
State Persistence: hydrated_bloc serializes state to disk for seamless recovery and better UX.
Rebuild Efficiency: BlocSelector and Equatable reduce unnecessary widget rebuilds by narrowing state exposure.
Scoped Architecture: Limit bloc scope and split logic into smaller cubits to improve modularity and testability.
Input Throttling: Debounce or throttle input-heavy events like search to reduce state churn.
Introduction
Advanced state management in Flutter becomes critical as applications scale in complexity. While the basic setState and Provider approaches work for small apps, leveraging the bloc pattern unlocks a clear separation between UI and business logic. In this tutorial, we’ll deep‐dive into reactive streams with bloc, structuring multiple blocs, persisting state with hydrated_bloc, and performance optimizations. Familiarity with Dart streams and basic bloc usage is assumed.
Reactive Streams and bloc
At the core of the bloc pattern is the transformation of incoming events into outgoing states using Streams. A typical bloc looks like this:
import 'package:bloc/bloc.dart';
enum CounterEvent { increment, decrement }
class CounterBloc extends Bloc<CounterEvent, int> {
CounterBloc() : super(0) {
on<CounterEvent>((event, emit) {
if (event == CounterEvent.increment) emit(state + 1);
if (event == CounterEvent.decrement) emit(state - 1);
});
}
}
Key points:
on<Event> handlers: Provide fine‐grained control and improve performance over mapEventToState.
Immutable states: Always emit new objects to avoid unexpected UI behavior.
Stream composition: Use transformEvents or RxDart operators (e.g., debounceTime) for advanced throttling or buffering.
Composing Blocs and Structuring Features
Large apps often require multiple blocs collaborating. Use MultiBlocProvider and event forwarding to isolate feature logic.
Example: A CartBloc listens to ProductBloc to update totals.
class ProductBloc extends Bloc<ProductEvent, ProductState> { /* … */ }
class CartBloc extends Bloc<CartEvent, CartState> {
CartBloc(ProductBloc productBloc) : super(CartState.empty()) {
// Forward selected product to cart
_subscription = productBloc.stream.listen((productState) {
if (productState is ProductSelected) {
add(AddItem(productState.product));
}
});
}
late final StreamSubscription _subscription;
@override
Future<void> close() {
_subscription.cancel();
return super.close();
}
}
Best practices:
Group related blocs in a feature folder.
Expose only necessary events/states across modules.
Avoid circular dependencies by defining pure event contracts.
State Persistence with hydrated_bloc
Persisting bloc state across app restarts enhances UX. The hydrated_bloc package serializes state to disk using json.
Setup:
void main() async {
WidgetsFlutterBinding.ensureInitialized();
HydratedBloc.storage = await HydratedStorage.build(
storageDirectory: await getApplicationDocumentsDirectory(),
);
runApp(MyApp());
}
Hydrated bloc:
class SettingsBloc extends HydratedBloc<SettingsEvent, SettingsState> {
SettingsBloc() : super(SettingsState.initial());
@override
SettingsState fromJson(Map<String, dynamic> json) =>
SettingsState(theme: json['theme'] as String);
@override
Map<String, dynamic> toJson(SettingsState state) =>
{'theme': state.theme};
}
Tips:
Keep JSON light and avoid large binary payloads.
Invalidate cache via clear() on major schema changes.
Encrypt sensitive data using custom storage adapters.
Performance Optimization and Best Practices
As your widget tree grows, you must avoid unnecessary rebuilds:
BlocSelector: Select only the slice of state your widget needs.
Equatable: Ensure state classes implement value‐based equality to prevent redundant updates.
Scoped bloc: Limit bloc scope to feature subtrees rather than global providers.
Debounce and throttle: For rapid user input (e.g., search), debounce events to reduce state churn.
Example using BlocSelector:
BlocSelector<CounterBloc, int, bool>(
selector: (count) => count.isEven,
builder: (context, isEven) {
return Text(isEven ? 'Even' : 'Odd');
},
)
Also consider:
Splitting a large bloc into smaller cubits for distinct domains.
Avoid heavy synchronous logic in blocs; offload to repositories or isolate heavy computations.
Profile your app with Flutter DevTools to spot rebuild hotspots.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
In this tutorial, we covered advanced Flutter state management using the bloc pattern, from reactive streams and multi‐bloc composition to state hydration and performance tuning. By adopting these techniques, you’ll maintain a scalable, testable codebase and deliver smooth user experiences.
Introduction
Advanced state management in Flutter becomes critical as applications scale in complexity. While the basic setState and Provider approaches work for small apps, leveraging the bloc pattern unlocks a clear separation between UI and business logic. In this tutorial, we’ll deep‐dive into reactive streams with bloc, structuring multiple blocs, persisting state with hydrated_bloc, and performance optimizations. Familiarity with Dart streams and basic bloc usage is assumed.
Reactive Streams and bloc
At the core of the bloc pattern is the transformation of incoming events into outgoing states using Streams. A typical bloc looks like this:
import 'package:bloc/bloc.dart';
enum CounterEvent { increment, decrement }
class CounterBloc extends Bloc<CounterEvent, int> {
CounterBloc() : super(0) {
on<CounterEvent>((event, emit) {
if (event == CounterEvent.increment) emit(state + 1);
if (event == CounterEvent.decrement) emit(state - 1);
});
}
}
Key points:
on<Event> handlers: Provide fine‐grained control and improve performance over mapEventToState.
Immutable states: Always emit new objects to avoid unexpected UI behavior.
Stream composition: Use transformEvents or RxDart operators (e.g., debounceTime) for advanced throttling or buffering.
Composing Blocs and Structuring Features
Large apps often require multiple blocs collaborating. Use MultiBlocProvider and event forwarding to isolate feature logic.
Example: A CartBloc listens to ProductBloc to update totals.
class ProductBloc extends Bloc<ProductEvent, ProductState> { /* … */ }
class CartBloc extends Bloc<CartEvent, CartState> {
CartBloc(ProductBloc productBloc) : super(CartState.empty()) {
// Forward selected product to cart
_subscription = productBloc.stream.listen((productState) {
if (productState is ProductSelected) {
add(AddItem(productState.product));
}
});
}
late final StreamSubscription _subscription;
@override
Future<void> close() {
_subscription.cancel();
return super.close();
}
}
Best practices:
Group related blocs in a feature folder.
Expose only necessary events/states across modules.
Avoid circular dependencies by defining pure event contracts.
State Persistence with hydrated_bloc
Persisting bloc state across app restarts enhances UX. The hydrated_bloc package serializes state to disk using json.
Setup:
void main() async {
WidgetsFlutterBinding.ensureInitialized();
HydratedBloc.storage = await HydratedStorage.build(
storageDirectory: await getApplicationDocumentsDirectory(),
);
runApp(MyApp());
}
Hydrated bloc:
class SettingsBloc extends HydratedBloc<SettingsEvent, SettingsState> {
SettingsBloc() : super(SettingsState.initial());
@override
SettingsState fromJson(Map<String, dynamic> json) =>
SettingsState(theme: json['theme'] as String);
@override
Map<String, dynamic> toJson(SettingsState state) =>
{'theme': state.theme};
}
Tips:
Keep JSON light and avoid large binary payloads.
Invalidate cache via clear() on major schema changes.
Encrypt sensitive data using custom storage adapters.
Performance Optimization and Best Practices
As your widget tree grows, you must avoid unnecessary rebuilds:
BlocSelector: Select only the slice of state your widget needs.
Equatable: Ensure state classes implement value‐based equality to prevent redundant updates.
Scoped bloc: Limit bloc scope to feature subtrees rather than global providers.
Debounce and throttle: For rapid user input (e.g., search), debounce events to reduce state churn.
Example using BlocSelector:
BlocSelector<CounterBloc, int, bool>(
selector: (count) => count.isEven,
builder: (context, isEven) {
return Text(isEven ? 'Even' : 'Odd');
},
)
Also consider:
Splitting a large bloc into smaller cubits for distinct domains.
Avoid heavy synchronous logic in blocs; offload to repositories or isolate heavy computations.
Profile your app with Flutter DevTools to spot rebuild hotspots.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
In this tutorial, we covered advanced Flutter state management using the bloc pattern, from reactive streams and multi‐bloc composition to state hydration and performance tuning. By adopting these techniques, you’ll maintain a scalable, testable codebase and deliver smooth user experiences.
Elevate your app logic with Vibe Studio
Elevate your app logic with Vibe Studio
Elevate your app logic with Vibe Studio
Elevate your app logic with Vibe Studio
Vibe Studio streamlines advanced state management setups, making state persistence simple—even for non-coders.
Vibe Studio streamlines advanced state management setups, making state persistence simple—even for non-coders.
Vibe Studio streamlines advanced state management setups, making state persistence simple—even for non-coders.
Vibe Studio streamlines advanced state management setups, making state persistence simple—even for non-coders.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






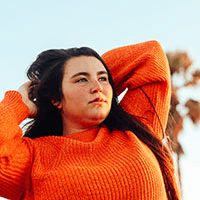



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025