Flutter Advanced Accessibility: Semantic Nodes and Custom Actions
May 8, 2025



Summary
Summary
Summary
Summary
The tutorial explores advanced Flutter accessibility techniques using semantic nodes and custom actions, offering strategies to improve screen-reader support, create meaningful interactions, and optimize performance.
The tutorial explores advanced Flutter accessibility techniques using semantic nodes and custom actions, offering strategies to improve screen-reader support, create meaningful interactions, and optimize performance.
The tutorial explores advanced Flutter accessibility techniques using semantic nodes and custom actions, offering strategies to improve screen-reader support, create meaningful interactions, and optimize performance.
The tutorial explores advanced Flutter accessibility techniques using semantic nodes and custom actions, offering strategies to improve screen-reader support, create meaningful interactions, and optimize performance.
Key insights:
Key insights:
Key insights:
Key insights:
Semantic Node Control: Use
Semantics
widgets to define labels, hints, values, and roles for assistive tech.Custom Actions: Add bespoke interactions to accessibility menus using
SemanticsActionHandler
.Grouping & Focus: Use
MergeSemantics
andFocusableActionDetector
to refine screen-reader flow and keyboard navigation.Testing Tools: Leverage semantic debuggers, TalkBack, VoiceOver, and automated audits for thorough testing.
Performance Balance: Optimize semantic trees by grouping, excluding decorative elements, and caching dynamic values.
Inclusive Design Focus: Accessibility should enhance UX meaningfully, not just meet minimum requirements.
Introduction
As Flutter apps grow in complexity, ensuring inclusive design becomes critical. This tutorial dives into accessibility advanced techniques, focusing on semantic nodes and custom actions. You’ll learn how to fine-tune screen-reader experiences, define bespoke interactions, and maintain performance. By mastering these accessibility improvements, you’ll deliver highly usable apps for all users.
Understanding Semantic Nodes in Flutter
Flutter’s accessibility layer represents each render object as a SemanticsNode. These nodes expose properties like labels, hints, roles, and tappable areas to assistive technologies (TalkBack, VoiceOver). By default, widgets like Text, Button, and IconButton generate basic semantics. For advanced accessibility, you often need to modify or create custom nodes.
Use the Semantics widget to override or extend defaults:
Semantics(
label: 'Profile picture, double tap to open settings',
button: true,
onTap: () => Navigator.pushNamed(context, '/profile'),
child: CircleAvatar(
radius: 30,
backgroundImage: NetworkImage(user.photoUrl),
),
)
Key properties:
• label – A description read aloud.
• hint – Guidance for complex interactions.
• value – Dynamic state information (e.g., “3 of 5”).
• increasedValue/decreasedValue – For sliders or steppers.
• toggled – Boolean for checkboxes, switches.
Beyond one-to-one overrides, use MergeSemantics to group multiple widgets into a single semantic node. This avoids fragmented announcements:
MergeSemantics(
child: Row(
children: [
Icon(Icons.warning, color: Colors.red),
Text('Low battery'),
Text('20% remaining'),
],
),
)
Implementing Custom Actions
Custom actions let you attach arbitrary commands to semantic nodes, unlocking advanced accessibility scenarios. For example, a drawing app might support “undo” and “redo” via the accessibility menu.
Define a custom action by supplying a SemanticsActionHandler:
Semantics(
label: 'Canvas, draw area',
customSemanticsActions: {
CustomSemanticsAction(label: 'Undo'): () => canvasController.undo(),
CustomSemanticsAction(label: 'Redo'): () => canvasController.redo(),
},
child: RepaintBoundary(
child: CustomPaint(painter: MyCanvasPainter(canvasController)),
),
)
Assistive technologies will list “Undo” and “Redo” in their gesture/action menus. Use descriptive labels and localize them. Combine custom actions with highlightable focus nodes by wrapping your widget in a FocusableActionDetector, further bridging keyboard accessibility and screen readers:
FocusableActionDetector(
shortcuts: {LogicalKeySet(LogicalKeyboardKey.keyZ): UndoIntent()},
actions: {
UndoIntent: CallbackAction<UndoIntent>(
onInvoke: (_) => canvasController.undo(),
),
},
child: Semantics(
label: 'Draw area',
customSemanticsActions: {CustomSemanticsAction(label: 'Undo'): () => canvasController.undo()},
child: Container(color: Colors.white),
),
)
Testing and Debugging Accessibility
Automate and manual testing both matter. Flutter’s accessibilityAdvanced tooling includes semantics debugger overlays and integration with a11y audit tools.
Enable the semantics debugger in your app:
WidgetsApp.debugAllowBannerOverride = false;
WidgetsBinding.instance.debugSemantics = true;
In debug mode, run flutter run --show-semantic-debugger to visualize semantic nodes. Colored outlines reveal bounding boxes and hierarchy. Inspect grouping, role assignments, and ensure no unlabeled tappable areas.
For manual testing:
• Android: Use TalkBack gestures to navigate focus. Verify labels, hints, and custom actions appear.
• iOS: Enable VoiceOver. Confirm swipe and rotor settings trigger your custom actions.
Automated audits can be integrated via Flutter’s flutter_test package:
testWidgets('Every tappable has a label', (tester) async {
await tester.pumpWidget(MyApp());
final List<SemanticsNode> nodes = tester.binding.pipelineOwner.semanticsOwner!.rootSemanticsNode!.getChildrenInTraversalOrder();
for (final node in nodes) {
if (node.hasAction(SemanticsAction.tap)) {
expect(node.label, isNotEmpty, reason: 'Tappable node missing label: $node');
}
}
});
Performance Considerations
Adding semantics come at a cost. Each Semantics widget introduces additional objects and tree complexity. Overusing MergeSemantics or nested Semantics can degrade frame rendering. Follow these guidelines for optimal performance:
• Group siblings with MergeSemantics instead of wrapping each child.
• Remove redundant semantics on purely decorative widgets by setting excludeSemantics: true.
• Profile semantics in release mode; debug overhead can differ from production.
• Cache results for dynamic labels or values to avoid unnecessary rebuilds.
By balancing granular control with performance, your advanced accessibility support will scale without slowing down animations or user interactions.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Advanced accessibility is about more than ticking off checkboxes; it’s about crafting meaningful experiences for every user. By leveraging semantic nodes and custom actions, you can provide rich interactions that work seamlessly with assistive technologies. Continually test, profile, and iterate to uphold both usability and performance standards.
Introduction
As Flutter apps grow in complexity, ensuring inclusive design becomes critical. This tutorial dives into accessibility advanced techniques, focusing on semantic nodes and custom actions. You’ll learn how to fine-tune screen-reader experiences, define bespoke interactions, and maintain performance. By mastering these accessibility improvements, you’ll deliver highly usable apps for all users.
Understanding Semantic Nodes in Flutter
Flutter’s accessibility layer represents each render object as a SemanticsNode. These nodes expose properties like labels, hints, roles, and tappable areas to assistive technologies (TalkBack, VoiceOver). By default, widgets like Text, Button, and IconButton generate basic semantics. For advanced accessibility, you often need to modify or create custom nodes.
Use the Semantics widget to override or extend defaults:
Semantics(
label: 'Profile picture, double tap to open settings',
button: true,
onTap: () => Navigator.pushNamed(context, '/profile'),
child: CircleAvatar(
radius: 30,
backgroundImage: NetworkImage(user.photoUrl),
),
)
Key properties:
• label – A description read aloud.
• hint – Guidance for complex interactions.
• value – Dynamic state information (e.g., “3 of 5”).
• increasedValue/decreasedValue – For sliders or steppers.
• toggled – Boolean for checkboxes, switches.
Beyond one-to-one overrides, use MergeSemantics to group multiple widgets into a single semantic node. This avoids fragmented announcements:
MergeSemantics(
child: Row(
children: [
Icon(Icons.warning, color: Colors.red),
Text('Low battery'),
Text('20% remaining'),
],
),
)
Implementing Custom Actions
Custom actions let you attach arbitrary commands to semantic nodes, unlocking advanced accessibility scenarios. For example, a drawing app might support “undo” and “redo” via the accessibility menu.
Define a custom action by supplying a SemanticsActionHandler:
Semantics(
label: 'Canvas, draw area',
customSemanticsActions: {
CustomSemanticsAction(label: 'Undo'): () => canvasController.undo(),
CustomSemanticsAction(label: 'Redo'): () => canvasController.redo(),
},
child: RepaintBoundary(
child: CustomPaint(painter: MyCanvasPainter(canvasController)),
),
)
Assistive technologies will list “Undo” and “Redo” in their gesture/action menus. Use descriptive labels and localize them. Combine custom actions with highlightable focus nodes by wrapping your widget in a FocusableActionDetector, further bridging keyboard accessibility and screen readers:
FocusableActionDetector(
shortcuts: {LogicalKeySet(LogicalKeyboardKey.keyZ): UndoIntent()},
actions: {
UndoIntent: CallbackAction<UndoIntent>(
onInvoke: (_) => canvasController.undo(),
),
},
child: Semantics(
label: 'Draw area',
customSemanticsActions: {CustomSemanticsAction(label: 'Undo'): () => canvasController.undo()},
child: Container(color: Colors.white),
),
)
Testing and Debugging Accessibility
Automate and manual testing both matter. Flutter’s accessibilityAdvanced tooling includes semantics debugger overlays and integration with a11y audit tools.
Enable the semantics debugger in your app:
WidgetsApp.debugAllowBannerOverride = false;
WidgetsBinding.instance.debugSemantics = true;
In debug mode, run flutter run --show-semantic-debugger to visualize semantic nodes. Colored outlines reveal bounding boxes and hierarchy. Inspect grouping, role assignments, and ensure no unlabeled tappable areas.
For manual testing:
• Android: Use TalkBack gestures to navigate focus. Verify labels, hints, and custom actions appear.
• iOS: Enable VoiceOver. Confirm swipe and rotor settings trigger your custom actions.
Automated audits can be integrated via Flutter’s flutter_test package:
testWidgets('Every tappable has a label', (tester) async {
await tester.pumpWidget(MyApp());
final List<SemanticsNode> nodes = tester.binding.pipelineOwner.semanticsOwner!.rootSemanticsNode!.getChildrenInTraversalOrder();
for (final node in nodes) {
if (node.hasAction(SemanticsAction.tap)) {
expect(node.label, isNotEmpty, reason: 'Tappable node missing label: $node');
}
}
});
Performance Considerations
Adding semantics come at a cost. Each Semantics widget introduces additional objects and tree complexity. Overusing MergeSemantics or nested Semantics can degrade frame rendering. Follow these guidelines for optimal performance:
• Group siblings with MergeSemantics instead of wrapping each child.
• Remove redundant semantics on purely decorative widgets by setting excludeSemantics: true.
• Profile semantics in release mode; debug overhead can differ from production.
• Cache results for dynamic labels or values to avoid unnecessary rebuilds.
By balancing granular control with performance, your advanced accessibility support will scale without slowing down animations or user interactions.
Vibe Studio

Vibe Studio, powered by Steve’s advanced AI agents, is a revolutionary no-code, conversational platform that empowers users to quickly and efficiently create full-stack Flutter applications integrated seamlessly with Firebase backend services. Ideal for solo founders, startups, and agile engineering teams, Vibe Studio allows users to visually manage and deploy Flutter apps, greatly accelerating the development process. The intuitive conversational interface simplifies complex development tasks, making app creation accessible even for non-coders.
Conclusion
Advanced accessibility is about more than ticking off checkboxes; it’s about crafting meaningful experiences for every user. By leveraging semantic nodes and custom actions, you can provide rich interactions that work seamlessly with assistive technologies. Continually test, profile, and iterate to uphold both usability and performance standards.
Design Accessible Apps with Vibe Studio
Design Accessible Apps with Vibe Studio
Design Accessible Apps with Vibe Studio
Design Accessible Apps with Vibe Studio
Vibe Studio helps you build Flutter apps that are not only functional—but inclusive, performant, and accessible by default.
Vibe Studio helps you build Flutter apps that are not only functional—but inclusive, performant, and accessible by default.
Vibe Studio helps you build Flutter apps that are not only functional—but inclusive, performant, and accessible by default.
Vibe Studio helps you build Flutter apps that are not only functional—but inclusive, performant, and accessible by default.
References
References
References
References
Join a growing community of builders today
Join a growing
community
of builders today
Join a growing
community
of builders today






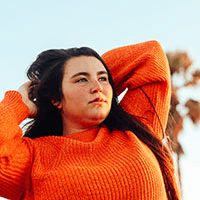



© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025


© Steve • All Rights Reserved 2025